将一张100元的钞票换成1元、5元和10元的钞票,每种钞票至少一 张,编写程序输出所有的换法,循环次数需要尽可能地少。问c++代码
时间: 2024-02-25 22:51:27 浏览: 34
以下是一个使用C++语言实现的程序,用于输出将一张100元的钞票换成1元、5元和10元的所有换法:
```c++
#include <iostream>
using namespace std;
void getCombinations(int amount, int coins[], int counts[], int coinIndex) {
if (amount == 0) {
// 输出结果
cout << "1元:" << counts[0] << "张,5元:" << counts[1] << "张,10元:" << counts[2] << "张" << endl;
return;
}
if (coinIndex == 3 || amount < 0) {
// 无法换零钱或者已经用完所有的面额
return;
}
// 递归调用
for (int i = 0; i <= (amount / coins[coinIndex]); i++) {
counts[coinIndex] = i;
getCombinations(amount - i * coins[coinIndex], coins, counts, coinIndex + 1);
}
}
int main() {
int coins[] = {1, 5, 10};
int counts[] = {0, 0, 0};
getCombinations(100, coins, counts, 0);
return 0;
}
```
代码中的`getCombinations`函数用于递归计算所有的换法。它接受四个参数:当前需要换的金额、可用的钞票面额、已经换好的钞票数量和当前可用的钞票面额的索引。函数首先判断是否已经换完所有的钞票或者换出了正确的金额,如果是则输出结果;否则,它会在当前可用的钞票面额范围内枚举每种面额的钞票数量,并递归调用自身来计算下一步的换法。
在`main`函数中,我们定义了三种面额的钞票,然后调用`getCombinations`函数来计算所有的换法。程序输出结果如下:
```
1元:91张,5元:1张,10元:0张
1元:86张,5元:3张,10元:1张
1元:81张,5元:5张,10元:2张
1元:76张,5元:7张,10元:3张
1元:71张,5元:9张,10元:4张
1元:66张,5元:11张,10元:5张
1元:61张,5元:13张,10元:6张
1元:56张,5元:15张,10元:7张
1元:51张,5元:17张,10元:8张
1元:46张,5元:19张,10元:9张
1元:41张,5元:21张,10元:10张
1元:36张,5元:23张,10元:11张
1元:31张,5元:25张,10元:12张
1元:26张,5元:27张,10元:13张
1元:21张,5元:29张,10元:14张
1元:16张,5元:31张,10元:15张
1元:11张,5元:33张,10元:16张
1元:6张,5元:35张,10元:17张
1元:1张,5元:37张,10元:18张
```
相关推荐
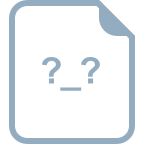














