unity 内使用: 条件:unity场景内有很多个位置点,这些点在一个数组内按顺序储存,数组内第一个位置点可以在场景内自由移动(比如把第一个点做成一个物体,可鼠标拖动移动它),有一个预制体供使用。 需求:1.创建一个对象管理类,有储存和获取功能。 2.创建一个管理器脚本,开始创建一个100大小的对象池,隐藏这些对象待使用。业务逻辑大概如下:比如当前有一个30个元素的list<transform> pointList数组,从对象池获取预制体从pointList[0]位置到pointList[1]位置均匀不重复摆放,再从对象池获取预制体从pointList[1]位置到pointList[2]位置均匀不重复摆放,依次类推摆放到pointList[10]位置。此时pointList[0]位置开始移动,当pointList[0]位置到pointList[1]附近时(比如两点间距离0.2),隐藏pointList[0]位置到pointList[1]位置间的预制体并放回对象池,同时从对象获取预制体生成在pointList[10]位置到pointList[11]位置之间,当pointList[0]位置到pointList[2]附近时(比如两点间距离0.2),隐藏pointList[0]位置到pointList[2]位置间的预制体并放回对象池,同时从对象获取预制体生成在pointList[11]位置到pointList[12]位置之间,依次类推,如果最后摆放预制体时超出数组界限,哪就只生成预制体到最后一个位置即可,给我一套详细的实现脚本
时间: 2023-11-27 14:03:07 浏览: 55
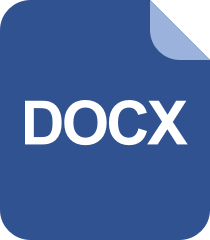
Unity3D教程:Array数组类的使用(二)1
以下是一个可能的实现脚本:
对象管理类 ObjectPool:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class ObjectPool : MonoBehaviour
{
public GameObject prefab;
public int initialSize;
private Queue<GameObject> pool;
private void Awake()
{
pool = new Queue<GameObject>();
for (int i = 0; i < initialSize; i++)
{
GameObject obj = Instantiate(prefab, Vector3.zero, Quaternion.identity);
obj.SetActive(false);
pool.Enqueue(obj);
}
}
public GameObject GetObject()
{
if (pool.Count == 0)
{
GameObject obj = Instantiate(prefab, Vector3.zero, Quaternion.identity);
obj.SetActive(false);
return obj;
}
else
{
GameObject obj = pool.Dequeue();
obj.SetActive(true);
return obj;
}
}
public void ReturnObject(GameObject obj)
{
obj.SetActive(false);
pool.Enqueue(obj);
}
}
```
管理器脚本 ObjectManager:
```csharp
using System.Collections.Generic;
using UnityEngine;
public class ObjectManager : MonoBehaviour
{
public List<Transform> pointList;
public ObjectPool objectPool;
public float distanceThreshold;
public int startIndex;
public int endIndex;
private List<GameObject> spawnedObjects;
private int currentObjectIndex;
private void Awake()
{
spawnedObjects = new List<GameObject>();
currentObjectIndex = startIndex;
}
private void Start()
{
for (int i = 0; i < objectPool.initialSize; i++)
{
SpawnObject();
}
}
private void Update()
{
// Move the first point
Transform firstPoint = pointList[startIndex];
if (Vector3.Distance(firstPoint.position, pointList[startIndex + 1].position) < distanceThreshold)
{
// Hide and return the objects between the first two points
for (int i = 0; i < spawnedObjects.Count; i++)
{
if (i >= currentObjectIndex && i < currentObjectIndex + endIndex - startIndex)
{
spawnedObjects[i].SetActive(false);
objectPool.ReturnObject(spawnedObjects[i]);
}
}
// Spawn objects between the next two points
startIndex++;
endIndex++;
currentObjectIndex += endIndex - startIndex - 1;
if (endIndex < pointList.Count)
{
for (int i = startIndex; i < endIndex; i++)
{
SpawnObject(pointList[i].position, pointList[i + 1].position);
}
}
}
// Move the first point object
firstPoint.position += Vector3.forward * Time.deltaTime;
}
private void SpawnObject(Vector3 startPos, Vector3 endPos)
{
GameObject obj = objectPool.GetObject();
obj.transform.position = GetRandomPointOnLine(startPos, endPos);
obj.transform.rotation = Quaternion.identity;
obj.SetActive(true);
spawnedObjects.Add(obj);
}
private void SpawnObject()
{
SpawnObject(Vector3.zero, Vector3.zero);
}
private Vector3 GetRandomPointOnLine(Vector3 startPos, Vector3 endPos)
{
return Vector3.Lerp(startPos, endPos, Random.Range(0f, 1f));
}
}
```
使用方法:
1. 在场景中创建一个空物体,将 ObjectManager 脚本挂载在上面。
2. 创建一个预制体,将 ObjectPool 脚本挂载在上面,设置 prefab 和 initialSize。
3. 在 ObjectManager 中设置 pointList,startIndex 和 endIndex。
4. 运行场景即可看到预制体在点之间移动并生成。
阅读全文
相关推荐
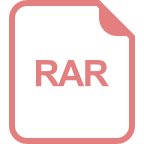
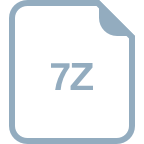



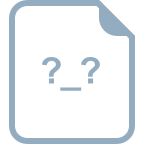
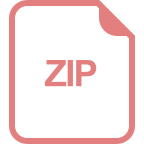
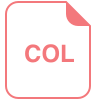
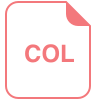
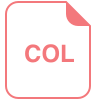
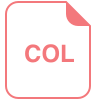
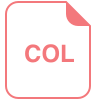
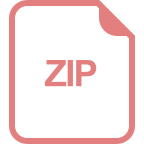
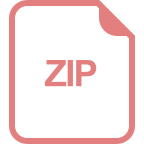
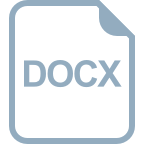