使用python写一个强化学习的网络
时间: 2023-07-19 19:45:03 浏览: 85
好的,我可以为您提供一个简单的强化学习网络的 Python 实现示例。这个网络使用 Q-learning 算法来训练一个智能体在迷宫中寻找宝藏。
首先,需要导入相关的库,包括 numpy, random 和 time:
```python
import numpy as np
import random
import time
```
然后,定义迷宫的大小和宝藏的位置:
```python
# 迷宫的大小
N_ROWS = 6
N_COLS = 9
# 宝藏的位置
treasure_row = 2
treasure_col = 8
```
接着,定义智能体的初始位置和动作空间:
```python
# 智能体的初始位置
agent_row = 0
agent_col = 0
# 动作空间
actions = ['up', 'down', 'left', 'right']
num_actions = len(actions)
```
接下来,定义 Q 表格并初始化为零:
```python
# 初始化 Q 表格为零
Q_table = np.zeros((N_ROWS, N_COLS, num_actions))
```
然后,定义 Q-learning 算法的参数,包括学习率、折扣因子和探索率:
```python
# Q-learning 算法的参数
learning_rate = 0.1
discount_factor = 0.99
exploration_rate = 0.1
```
接着,定义一个函数来选择下一个动作。这个函数使用 ε-贪心策略来选择动作,即以一定的概率选择最优动作,以一定的概率随机选择动作。
```python
# 选择下一个动作
def select_action(state):
if random.uniform(0, 1) < exploration_rate:
# 随机选择动作
action = random.choice(actions)
else:
# 选择最优动作
action = actions[np.argmax(Q_table[state[0], state[1], :])]
return action
```
然后,定义一个函数来执行一个动作并更新 Q 表格。这个函数接收当前状态和动作作为输入,并返回新的状态和奖励。
```python
# 执行一个动作并更新 Q 表格
def take_action(state, action):
if action == 'up':
new_state = (max(state[0] - 1, 0), state[1])
elif action == 'down':
new_state = (min(state[0] + 1, N_ROWS - 1), state[1])
elif action == 'left':
new_state = (state[0], max(state[1] - 1, 0))
elif action == 'right':
new_state = (state[0], min(state[1] + 1, N_COLS - 1))
if new_state == (treasure_row, treasure_col):
# 找到宝藏
reward = 1
else:
# 没有找到宝藏
reward = 0
Q_table[state[0], state[1], actions.index(action)] += learning_rate * (reward + discount_factor * np.max(Q_table[new_state[0], new_state[1], :]) - Q_table[state[0], state[1], actions.index(action)])
return new_state, reward
```
最后,定义一个主函数来训练智能体并测试其性能:
```python
# 主函数
def main():
# 训练智能体
for i in range(1000):
state = (agent_row, agent_col)
done = False
while not done:
# 选择动作
action = select_action(state)
# 执行动作并更新 Q 表格
new_state, reward = take_action(state, action)
# 更新状态
state = new_state
# 判断是否完成任务
if state == (treasure_row, treasure_col):
done = True
# 降低探索率
exploration_rate *= 0.99
# 测试智能体
state = (agent_row, agent_col)
done = False
steps = 0
while not done:
# 选择最优动作
action = actions[np.argmax(Q_table[state[0], state[1], :])]
# 执行动作并更新状态
new_state, reward = take_action(state, action)
# 更新步数
steps += 1
# 输出状态
print('Step:', steps)
print('State:', new_state)
print('Reward:', reward)
# 判断是否完成任务
if new_state == (treasure_row, treasure_col):
done = True
# 更新状态
state = new_state
# 等待一段时间
time.sleep(0.5)
if __name__ == '__main__':
main()
```
这个示例实现了一个简单的 Q-learning 算法来训练一个智能体在迷宫中寻找宝藏。您可以根据自己的需要进行修改和扩展。
相关推荐
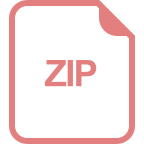
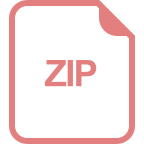
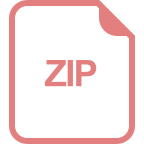
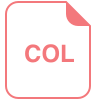
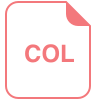
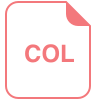
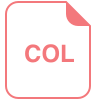
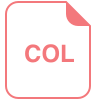









