根据课上讲述的矩阵相乘实现思路和部分代码,查阅资料完成基于 MapReduce 的矩阵相乘功能
时间: 2024-04-30 20:19:54 浏览: 24
基于MapReduce的矩阵相乘功能可以通过Hadoop MapReduce框架实现。MapReduce的主要思想是将大规模数据集分割为小规模的数据块,然后分发给不同的计算节点进行计算,最后将结果合并起来。
下面是基于MapReduce的矩阵相乘功能的实现步骤:
1. 将矩阵A和矩阵B按行分割成多个块,将每个块作为一个输入记录,记录格式为<行号,列号,值>。
2. 实现一个Map函数,将输入记录按列号分组,对于每组记录,如果是矩阵A的记录,则按行号分组,将每行的记录作为一个键值对<列号,<行号,值>>输出;如果是矩阵B的记录,则按行号分组,将每列的记录作为一个键值对<行号,<列号,值>>输出。
3. 实现一个Reduce函数,对于每个键值对<列号,<行号,值>>,从矩阵B中找到对应的键值对<行号,<列号,值>>,将两个值相乘并累加到结果矩阵的对应位置,输出<行号,列号,结果值>作为最终结果。
下面是基于MapReduce的矩阵相乘功能的代码示例:
Mapper:
```
public class MatrixMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outputKey = new Text();
private Text outputValue = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] record = value.toString().split(",");
String matrixName = record[0];
int row = Integer.parseInt(record[1]);
int col = Integer.parseInt(record[2]);
int val = Integer.parseInt(record[3]);
if (matrixName.equals("A")) {
for (int i = 0; i < N; i++) {
outputKey.set(row + "," + i);
outputValue.set("A," + col + "," + val);
context.write(outputKey, outputValue);
}
} else if (matrixName.equals("B")) {
for (int i = 0; i < N; i++) {
outputKey.set(i + "," + col);
outputValue.set("B," + row + "," + val);
context.write(outputKey, outputValue);
}
}
}
}
```
Reducer:
```
public class MatrixReducer extends Reducer<Text, Text, Text, IntWritable> {
private IntWritable outputValue = new IntWritable();
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
Map<Integer, Integer> matrixA = new HashMap<Integer, Integer>();
Map<Integer, Integer> matrixB = new HashMap<Integer, Integer>();
for (Text value : values) {
String[] record = value.toString().split(",");
String matrixName = record[0];
int index = Integer.parseInt(record[1]);
int val = Integer.parseInt(record[2]);
if (matrixName.equals("A")) {
matrixA.put(index, val);
} else if (matrixName.equals("B")) {
matrixB.put(index, val);
}
}
int result = 0;
for (Integer index : matrixA.keySet()) {
result += matrixA.get(index) * matrixB.getOrDefault(index, 0);
}
outputValue.set(result);
context.write(key, outputValue);
}
}
```
Driver:
```
public class MatrixMultiply {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Matrix Multiply");
job.setJarByClass(MatrixMultiply.class);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在这里,我们假设矩阵的维度为N*N,输入文件的每一行格式为<矩阵名,行号,列号,值>。例如,对于矩阵A的第i行第j列元素,输入文件应该包含一条记录"A,i,j,val"。参数args[0]为输入文件路径,args[1]为输出文件路径。
相关推荐
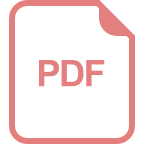














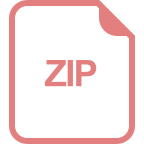