能否提供一个使用C++编写的示例,展示如何实现简单的加密(如Caesar Cipher)和解密算法的程序代码?
时间: 2024-11-13 14:18:09 浏览: 18
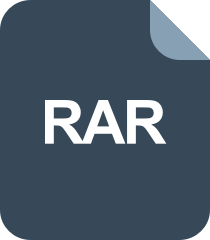
affine-caesar.rar_MOD_affine cipher_加密解密affine
当然可以。在C++中,我们可以使用简单的位移(Caesar Cipher)算法实现加密和解密。这个算法通过将字母表中的每个字符向前或向后移动固定的位置来进行加密。以下是一个基本的实现:
```cpp
#include <iostream>
#include <string>
// 函数声明
std::string encrypt(const std::string& plaintext, int shift);
std::string decrypt(const std::string& ciphertext, int shift);
int main() {
std::string message = "Hello, World!";
int shift_amount = 3; // 加密位移
// 加密
std::string encrypted = encrypt(message, shift_amount);
std::cout << "Encrypted message: " << encrypted << "\n";
// 解密
std::string decrypted = decrypt(encrypted, -shift_amount); // 使用负数解密
std::cout << "Decrypted message: " << decrypted << "\n";
return 0;
}
// Caesar Cipher 加密函数
std::string encrypt(const std::string& plaintext, int shift) {
std::string result;
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
char offset = static_cast<char>((c + shift - 'A') % 26 + 'A');
result += (islower(c) ? offset : toupper(offset)); // 保持大小写一致性
} else {
result += c;
}
}
return result;
}
// Caesar Cipher 解密函数
std::string decrypt(const std::string& ciphertext, int shift) {
return encrypt(ciphertext, -shift); // 使用相同的原理解密,只是位移方向相反
}
```
在这个例子中,`encrypt`函数接受明文字符串和位移量,然后对每个字符进行位移并返回加密后的结果。`decrypt`函数则用于解密,它使用相同的加密位移值,但由于我们减去位移量,实际上是在反方向上操作。
阅读全文
相关推荐
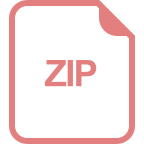
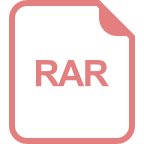
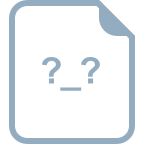
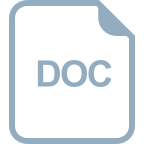
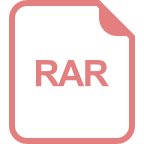
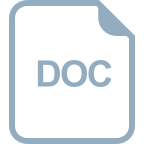
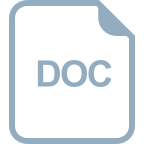
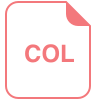










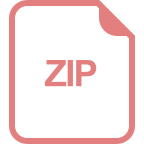