请帮我用C++创建一个顺序表,表中元素依次为:s、t、d、i、o(均为char类型);查找顺序表中是否包含'b'字符;从顺序表中取出第3个元素并返回其值;在第3个元素后面插入3个新的元素依次为:l、i、b;将顺序表中的最后两个元素删除;将顺序表中的元素输出。
时间: 2024-09-13 18:04:24 浏览: 46
在C++中,你可以使用数组或动态数组(如std::vector)来创建一个顺序表。这里我们选择使用数组。首先,我们需要声明一个数组,并初始化给定的元素。接着,我们可以实现查找、取值、插入和删除操作。这是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义一个大小为5的字符数组,用于存储顺序表
char sequence[5] = {'s', 't', 'd', 'i', 'o'};
// 查找操作
bool containsB() {
for (int i = 0; i < 5; i++) {
if (sequence[i] == 'b') return true;
}
return false;
}
// 取值操作
char getThirdElement() {
return sequence[2]; // 从0开始计数,所以第三个元素索引为2
}
// 插入操作
void insertAfterThird(char newElement) {
if (sequence) { // 检查数组是否为空
int index = 2;
for (; index < 5; index++) {
sequence[index + 1] = sequence[index];
}
sequence[index + 1] = newElement;
} else {
cout << "Array is empty to perform insertion." << endl;
}
}
// 删除操作
void removeLastTwo() {
if (sequence && sequence[4]) { // 如果最后一个元素存在
sequence[4] = '\0'; // 将最后一个元素设为终止符
if (sequence[3]) sequence[3] = '\0'; // 如果还有第三个元素,也设为终止符
} else {
cout << "Array does not have enough elements for deletion." << endl;
}
}
// 输出操作
void printSequence() {
cout << "Current sequence: ";
for (int i = 0; sequence[i] != '\0'; i++) {
cout << sequence[i] << " ";
}
cout << endl;
}
int main() {
// 测试以上函数
if (containsB()) {
cout << "'B' character exists in the sequence." << endl;
} else {
cout << "'B' character not found." << endl;
}
char thirdElement = getThirdElement();
cout << "The third element is: " << thirdElement << endl;
insertAfterThird('l');
insertAfterThird('i');
insertAfterThird('b');
removeLastTwo();
printSequence();
return 0;
}
```
阅读全文
相关推荐
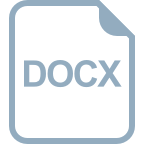
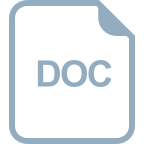
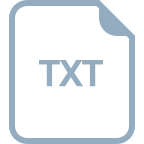
















