import sensor import image import time from pyb import UART sensor.reset() sensor.set_pixformat(sensor.RGB565) sensor.set_framesize(sensor.QQVGA) sensor.skip_frames(time=2000) clock = time.clock() # 初始化UART uart = UART(3, 115200) # 根据实际情况修改UART的端口和波特率 while True: clock.tick() img = sensor.snapshot().lens_corr(1.8) # 检测圆形 circles = img.find_circles(threshold=3500, x_margin=10, y_margin=10, r_margin=10, r_min=2, r_max=100, r_step=2) for c in circles: img.draw_circle(c.x(), c.y(), c.r(), color=(255, 0, 0)) uart.write("1") # 发送圆形标识为1 print('圆形') img = sensor.snapshot() # 检测矩形 rects = img.find_rects(threshold=10000) for r in rects: img.draw_rectangle(r.rect(), color=(255, 0, 0)) for p in r.corners(): img.draw_circle(p[0], p[1], 5, color=(0, 255, 0)) uart.write("2") # 发送矩形标识为2 print('矩形') # 检测三角形 sum_theta = 0 count = 0 lines = img.find_line_segments(merge_distance=10, max_theta_diff=10) for l in lines: img.draw_line(l.line(), color=(255, 0, 0)) sum_theta += l.theta() count += 1 avg_theta = sum_theta / count if count > 0 else 0 if 1 < avg_theta < 75: uart.write("3") # 发送三角形标识为3 print('三角形') print("FPS %f" % clock.fps())
时间: 2024-04-17 11:23:16 浏览: 291
这段代码是一个基于OpenMV的图像处理程序。它使用了OpenMV的库来进行图像处理和形状检测,并通过UART与其他设备进行通信。
代码的主要逻辑如下:
1. 初始化摄像头和串口。
2. 进入无限循环,每次循环读取一帧图像。
3. 在图像中检测圆形,如果检测到,则向串口发送"1"。
4. 再次读取图像。
5. 在图像中检测矩形,如果检测到,则向串口发送"2"。
6. 再次读取图像。
7. 在图像中检测三角形,如果检测到,则向串口发送"3"。
8. 打印当前帧率。
9. 重复步骤2-8。
请注意,此代码需要在OpenMV设备上运行,并且需要连接相应的传感器和串口设备。详细的代码功能和使用方法,请参考OpenMV官方文档和示例代码。
相关问题
可以把这段代码精简吗import sensor, image, time, lcd from pyb import UART sensor.reset() sensor.set_pixformat(sensor.RGB565) sensor.set_framesize(sensor.QQVGA) sensor.skip_frames(time = 2000) lcd.init() sensor.set_auto_gain(False) sensor.set_auto_whitebal(False) clock = time.clock() uart = UART(3, 19200, timeout_char=2000) while(True): clock.tick() img = sensor.snapshot().lens_corr(1.8) lcd.display(img) for c in img.find_circles(threshold = 3500, x_margin = 10, y_margin = 10, r_margin = 10, r_min = 2, r_max = 100, r_step = 2): area = (c.x()-c.r(), c.y()-c.r(), 2c.r(), 2c.r()) statistics = img.get_statistics(roi=area) print(statistics) if 0<statistics.l_mode()<100 and 0<statistics.a_mode()<127 and 0<statistics.b_mode()<127: img.draw_circle(c.x(), c.y(), c.r(), color = (255, 0, 0)) uart.write("find red cirl!\n") time.sleep_ms(2000) else: img.draw_rectangle(area, color = (255, 255, 255)) uart.write("nothing!\n") time.sleep_ms(2000) print("FPS %f" % clock.fps())
可以,精简后的代码如下:
```python
import sensor, image, time, lcd
from pyb import UART
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QQVGA)
sensor.skip_frames(time=2000)
lcd.init()
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
clock = time.clock()
uart = UART(3, 19200, timeout_char=2000)
while True:
clock.tick()
img = sensor.snapshot().lens_corr(1.8)
lcd.display(img)
for c in img.find_circles(threshold=3500, x_margin=10, y_margin=10, r_margin=10, r_min=2, r_max=100, r_step=2):
area = (c.x()-c.r(), c.y()-c.r(), 2*c.r(), 2*c.r())
statistics = img.get_statistics(roi=area)
if 0 < statistics.l_mode() < 100 and 0 < statistics.a_mode() < 127 and 0 < statistics.b_mode() < 127:
img.draw_circle(c.x(), c.y(), c.r(), color=(255, 0, 0))
uart.write("find red circle!\n")
time.sleep_ms(2000)
else:
img.draw_rectangle(area, color=(255, 255, 255))
uart.write("nothing!\n")
time.sleep_ms(2000)
print("FPS %f" % clock.fps())
```
这里主要是将代码进行了格式化,去除了一些冗余的空格和一些无用的注释。
THRESHOLD = (22, 0, -128, 127, -128, 127) import sensor, image, time from pyb import LED import time from pyb import UART import math LED(1).on() LED(2).on() LED(3).on() uart = UART(3,115200) sensor.reset() sensor.set_vflip(True) sensor.set_hmirror(True) sensor.set_pixformat(sensor.RGB565) sensor.set_framesize(sensor.QQQVGA) sensor.skip_frames(time = 2000) clock = time.clock() while(True): clock.tick() img = sensor.snapshot().binary([THRESHOLD]) line = img.get_regression([(100,100)], robust = True) if (line): rho_err = abs(line.rho())-img.width()/2 if line.theta()>90: theta_err = line.theta()-180 else: theta_err = line.theta() img.draw_line(line.line(), color = 127) print(rho_err,line.magnitude(),rho_err) pass 帮我分析这段代码
这段代码是一个简化版本的前面的代码,去除了控制车辆行驶的部分,仅保留了图像处理的部分。
代码首先导入了所需的库和模块,包括sensor、image、time、pyb和math。然后定义了一个阈值THRESHOLD,用于图像二值化。
接下来,代码进行了一些初始化设置,包括重置传感器、设置图像格式和帧大小等。
在主循环中,代码通过sensor.snapshot()获取当前图像,并通过二值化处理得到二值图像img。然后使用img.get_regression()函数检测图像中的直线。
如果检测到直线(line存在),则计算直线的偏移量rho_err和角度偏差theta_err,并绘制检测到的直线。
最后,代码通过打印输出显示rho_err、line.magnitude()和rho_err的值。
总体而言,这段代码的功能是基于图像检测直线,计算直线的偏移量和角度偏差,并绘制检测到的直线。具体的应用场景和后续的处理逻辑可能需要根据实际需求进行调整和优化。
阅读全文
相关推荐
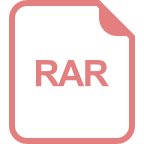
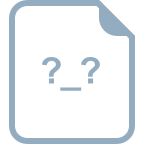











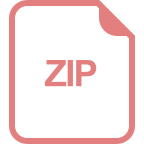
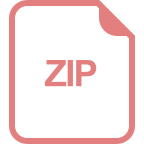
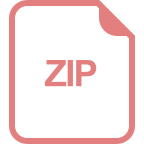