futures.add(executorService.submit(() -> table.get(subList)));
时间: 2024-04-19 09:24:52 浏览: 14
这段代码使用 `executorService.submit()` 方法将一个任务提交给 `executorService` 线程池进行执行,并将返回的 `Future` 对象添加到 `futures` 列表中。
在这里,使用了 Lambda 表达式 `() -> table.get(subList)` 创建了一个匿名的 `Callable` 对象,该对象会调用 `table.get(subList)` 方法进行计算。`submit()` 方法会将这个任务提交给线程池,并返回一个 `Future` 对象,以便你可以在需要时获取任务的执行结果。
通过将返回的 `Future` 对象添加到 `futures` 列表中,你可以稍后遍历列表,获取每个任务的执行结果或进行其他操作。
相关问题
再精简优化下这个段代码 int batchCount = 500; int dataSizePerThread = Math.max(1, awardIds.size() / batchCount); List<CompletableFuture<List<TCaAwardInfo>>> futures = new ArrayList<>(); for (int i = 0; i < dataSizePerThread; i++) { int startIndex = i * batchCount; int endIndex = Math.min((i + 1) * batchCount, awardIds.size()); List<Long> subList = awardIds.subList(startIndex, endIndex); CompletableFuture<List<TCaAwardInfo>> future = CompletableFuture.supplyAsync(() -> { List<TCaAwardInfo> result = new ArrayList<>(); result = awardApiService.queryActivationCode(subList); return result; }, taskExecutor); futures.add(future); } List<TCaAwardInfo> activationCodeList = futures.stream() .flatMap(future -> future.join().stream()) .collect(Collectors.toList());
可以将for循环中的部分代码提取出来,简化代码如下:
int batchCount = 500;
int dataSizePerThread = Math.max(1, awardIds.size() / batchCount);
List<CompletableFuture<List<TCaAwardInfo>>> futures = new ArrayList<>();
for (int i = 0; i < dataSizePerThread; i++) {
int startIndex = i * batchCount;
int endIndex = Math.min((i + 1) * batchCount, awardIds.size());
List<Long> subList = awardIds.subList(startIndex, endIndex);
CompletableFuture<List<TCaAwardInfo>> future = CompletableFuture.supplyAsync(() -> awardApiService.queryActivationCode(subList), taskExecutor);
futures.add(future);
}
List<TCaAwardInfo> activationCodeList = futures.stream()
.flatMap(CompletableFuture::join)
.collect(Collectors.toList());
这里简化了supplyAsync方法中的Lambda表达式,将返回值直接作为Lambda表达式的返回值,避免了创建ArrayList对象。同时使用方法引用的方式替换了flatMap中的Lambda表达式。
concurrent.futures.ThreadPoolExecutor shutdown
concurrent.futures.ThreadPoolExecutor的shutdown()方法被用来优雅地关闭线程池,确保所有的线程都已经完成任务并且退出。
shutdown()方法的作用是向线程池发送一个信号,告诉它停止接受新的任务,并且等待当前正在执行的任务完成后再关闭线程池。
在使用shutdown()方法之后,线程池将不再接受新的任务,而是等待已经提交的任务全部完成。
当所有的任务都完成之后,线程池会立即关闭。如果有任务尚未完成,线程池会等待所有任务完成后再关闭。
需要注意的是,shutdown()方法只会关闭线程池,而不会停止已经在执行的任务。如果需要立即停止正在执行的任务,可以使用shutdown(wait=True)方法。
这个方法会等待所有线程池中的任务都完成后再停止线程池。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [concurrent.futures模块ThreadPoolExecutor、ProcessPoolExecutor讲解及使用实例](https://blog.csdn.net/weixin_43587784/article/details/129167145)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [concurrent.futures.ThreadPoolExecutor并发库详解](https://blog.csdn.net/The_Time_Runner/article/details/99652083)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
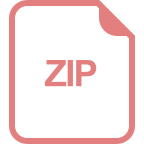
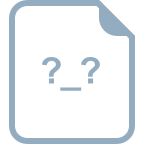













