ros操作系统中,如何使用pid算法控制小车的速度
时间: 2024-10-21 11:02:16 浏览: 57
在Robot Operating System (ROS)中,PID (Proportional-Integral-Derivative) 算法常用于对车辆速度进行精确控制。下面是一个基本步骤指南:
1. **安装所需库**:首先,确保已经安装了ros基本工具包以及任何必要的控制库,如`roscpp`, `geometry_msgs`等。
2. **编写控制节点**:创建一个新的ROS节点并初始化PID控制器。你可以使用像`std_srvs::Empty`这样的服务作为外部输入,以便从其他节点接收目标速度信号。
```cpp
#include <ros/ros.h>
#include <control_toolbox/pid.h>
class SpeedController {
public:
pid_t speed_pid;
};
int main(int argc, char *argv[]){
// 初始化ROS节点和PID控制器
SpeedController controller;
ros::NodeHandle nh;
double target_speed = 0; // 获取目标速度
double current_speed;
// 创建PID实例
controller.speed_pid = pidConstructor(nh, "speed_controller", 1, 0.1, 0); // Kp, Ki, Kd 设置
while (nh.ok()){
// 读取当前速度
nh.getParam("current_speed", current_speed);
// 计算PID输出(调整量)
double error = target_speed - current_speed;
double output = pidUpdate(&controller.speed_pid, error, nh.getWallTime());
// 发送速度命令给车辆
// 这里假设有一个名为"vehicle_cmd"的Topic,将输出速度作为msg发布出去
ros::Publisher cmd_pub = nh.advertise<geometry_msgs::Twist>("vehicle_cmd", 1);
geometry_msgs::Twist msg;
msg.linear.x = output;
cmd_pub.publish(msg);
nh.sleep(0.01); // 控制频率
}
}
```
3. **配置参数**:你需要在ROS参数服务器上设置PID系数(Kp, Ki, Kd),以及其他相关参数,如采样周期(`ros::Rate()`中的时间间隔)。
4. **调试与调整**:运行节点后,根据需要监控和调整PID参数,以达到良好的跟随性能。可能需要通过日志或可视化工具查看PID误差、积分误差等信息。
阅读全文
相关推荐
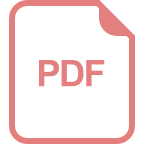
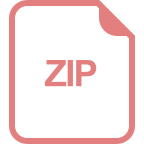
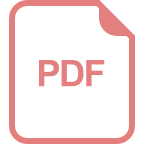
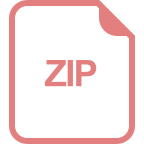
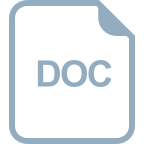
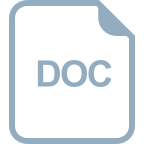
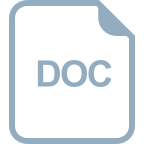
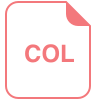
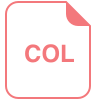









