【避免Python排序陷阱】:性能优化与常见误区破解
发布时间: 2024-09-01 00:12:25 阅读量: 150 订阅数: 62 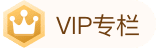
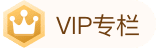
.webp)
# 1. Python排序机制的原理
Python中的排序机制是学习任何数据处理任务的基础。理解排序机制可以帮助我们编写更高效、更优雅的代码。Python排序依赖于`Timsort`算法,这是结合了归并排序和插入排序的一种算法。`Timsort`是Python内建的排序函数`sorted()`和列表的`.sort()`方法背后使用的算法,它针对连续序列进行了优化。
## 理解Python的Timsort算法
Python的排序算法Timsort是为真实世界的非随机数据设计的,它以较小的性能损失换取了对各种实际数据的广泛适用性。理解Timsort的工作原理,可以让我们更好地使用Python排序。Timsort算法会在小片段内使用插入排序,然后将排序好的片段归并,以达到整体排序的目的。它会根据数据的局部性原理,找出已经有序的子序列,并利用这些子序列进行排序。
## 排序在Python中的表现形式
Python排序机制的实现,不仅限于上述的排序算法。它还包括了对排序过程中各种边缘情况的处理,比如稳定排序,保证具有相同值的元素的相对位置不被改变。在排序时使用`key`参数,可以定义一个函数来指定排序的依据,这使得排序操作更为灵活。下一章将深入探讨如何优化Python的排序性能。
# 2. Python排序性能的优化策略
## 2.1 排序算法的基本原理
### 2.1.1 理解不同排序算法的时间复杂度
排序算法的时间复杂度是衡量算法效率的关键指标。为了深入理解,我们可以从几种常见的排序算法开始分析其时间复杂度。
- **冒泡排序**:冒泡排序是最简单的排序算法之一,其时间复杂度为O(n²),在最坏和平均的情况下都需要比较和交换n²次。尽管它实现起来很简单,但效率低下,仅适用于小数据集。
- **选择排序**:选择排序在每个步骤中选择最小的元素放到排序序列的起始位置。它的时间复杂度也是O(n²),而且由于其需要进行多次的交换操作,它通常比冒泡排序稍慢。
- **插入排序**:插入排序在进行排序时,将元素逐个插入到已排序的序列中。它的平均时间复杂度为O(n²),但当输入数据已经基本有序时,其效率可以接近O(n)。
- **快速排序**:快速排序是一种分而治之的算法,其平均时间复杂度为O(nlogn),最坏情况下为O(n²),但由于其平均表现优秀,快速排序是实际应用中最常用的排序算法之一。
- **归并排序**:归并排序是一种稳定的排序方法,它的时间复杂度始终为O(nlogn),不依赖于输入数据的初始顺序。由于它需要额外的空间来合并数组,所以它不是原地排序算法。
- **堆排序**:堆排序是一种原地排序算法,利用堆这种数据结构进行排序。其时间复杂度与快速排序相似,平均和最坏情况都是O(nlogn)。
### 2.1.2 空间复杂度对性能的影响
在排序算法中,空间复杂度同样重要,尤其对于大数据集。以下是几种常见排序算法的空间复杂度分析:
- **快速排序**:快速排序的空间复杂度主要取决于递归栈的深度,平均为O(logn),但在最坏的情况下会变成O(n)。
- **归并排序**:归并排序需要与原数组等长的额外空间来存放合并后的数组,空间复杂度为O(n)。
- **堆排序**:堆排序不需要额外的空间来存储子数组,因此它的空间复杂度为O(1)。
- **原地排序算法**:例如冒泡排序、选择排序和插入排序是原地排序算法,它们的空间复杂度为O(1)。
理解空间复杂度有助于我们根据实际应用场景选择合适的排序算法。
## 2.2 高效排序实践
### 2.2.1 使用内置的sorted()函数和list.sort()方法
Python提供了两个内置函数来处理排序任务,分别是`sorted()`和`list.sort()`。
- **sorted()**:这个函数接受任何可迭代对象并返回一个新的排序后的列表,不会修改原列表。
- **list.sort()**:这是一个列表的内置方法,用于就地排序,不返回任何值。
为了演示这两个函数的用法,可以使用以下代码示例:
```python
# 使用sorted()函数
numbers = [3, 1, 4, 1, 5, 9, 2]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # 输出:[1, 1, 2, 3, 4, 5, 9]
# 使用list.sort()方法
numbers.sort()
print(numbers) # 输出:[1, 1, 2, 3, 4, 5, 9]
```
在处理大数据集时,要避免使用`list.sort()`,因为它会改变原列表,如果需要保留原列表的顺序,应使用`sorted()`函数。
### 2.2.2 排序键(key)的优化技巧
在Python中,`sorted()`函数和`list.sort()`方法都可以接受一个`key`参数,这个参数是一个函数,用来在排序前对列表中的每个元素进行处理。
例如,如果你有一个元组列表,你可能只关心元组中某个特定位置的值来进行排序。你可以使用`key`参数来指定这个位置:
```python
people = [('Alice', 25), ('Bob', 20), ('Carol', 30)]
sorted_people = sorted(people, key=lambda x: x[1]) # 按年龄排序
print(sorted_people) # 输出:[('Bob', 20), ('Alice', 25), ('Carol', 30)]
```
在这个例子中,我们使用了一个lambda函数作为`key`参数的值。这样的优化技巧可以帮助我们避免预先构建一个排序用的新列表,减少内存消耗并提高效率。
### 2.2.3 复杂数据结构的排序方法
在处理包含复杂数据结构(如对象列表或字典列表)的排序时,我们可以使用`itemgetter`或`attrgetter`方法,它们比lambda函数更高效。
例如,假设我们有一个包含字典的列表,每个字典代表一个学生及其成绩:
```python
students = [
{'name': 'Alice', 'score': 88},
{'name': 'Bob', 'score': 75},
{'name': 'Carol', 'score': 91}
]
from operator import itemgetter
sorted_students = sorted(students, key=itemgetter('score'))
print(sorted_students) # 按成绩排序
```
这种方法不仅使代码更加简洁,而且由于`itemgetter`是专门用于获取对象属性的函数,因此执行效率更高。
## 2.3 排序优化的案例分析
### 2.3.1 大数据量排序优化
在处理大规模数据量时,排序性能变得尤为重要。例如,在数据科学和大数据分析中,数据集可能包含数十万或数百万行数据。在这些情况下,即使是微小的性能提升也可能对总体运行时间产生显著影响。
**案例说明**:
假设我们有一个大数据集,我们需要对它进行排序。一种优化方法是分块排序:将数据集分成多个小块,然后对每个块进行排序,最后将这些块合并起来。这种方法类似于归并排序的过程。
### 2.3.2 并行排序与多线程排序技巧
在现代多核处理器上,通过使用并行计算可以显著提高排序性能。
**案例说明**:
考虑一个大文件,需要对其行进行排序。我们可以将文件分成多个部分,并在多个线程或进程上并行排序这些部分。排序完成后,我们可以使用一个归并步骤将这些排序好的部分合并成一个完整的排序结果。
使用Python中的`concurrent.futures`模块可以帮助我们轻松地实现多线程排序:
```python
import concurrent.futures
from itertools import islice
def sort_file(file_path):
with open(file_path, 'r') as ***
***
***
*** [data[i:i + chunk_size] for i in range(0, len(data), chunk_size)]
# 并行排序各个块
with concurrent.futures.ThreadPoolExecutor() as executor:
sorted_chunks = executor.map(sorted, chunked_data)
# 归并排序后的块
sorted_data = merge_chunks(sorted_chunks)
with open(file_path, 'w') as ***
***
*** []
for lines in zip(*chunks):
sorted_chunks.extend(sorted(lines))
return sorted_chunks
# 假设有一个大文件路径
file_path = 'large_file.txt'
sort_file(file_path)
```
通过利用多核处理器,这种方法可以显著加速大规模数据集的排序过程。
# 3. ```
# 第三章:Python排序常见误区与破解
Python作为一门高级编程语言,其内置的排序功能是非常强大和灵活的,但同时也存在一些容易让人产生误解的方面。本章将深入分析这些常见误区,并提供相应的破解方法。
## 3.1 误区一:对排序算法的误解
### 3.1.1 分辨不同的排序算法适用场景
许多开发者在选择排序算法时,并没有深入分析不同场景下的排序需求,导致在不适用的场景中选择了错误的排序算法,从而造成性能低下。
例如,快速排序在平均情况下具有很好的时间复杂度,但其在最坏情况下的时间复杂度为O(n^2),且是不稳定的排序算法。而归并排序虽然时间复杂度稳定在O(n log n),但其空间复杂度较高。
**破解方法:**
选择排序算法时应考虑数据规模、数据类型、稳定性要求等因素。例如:
- **小规模数据**:直接插入排序或冒泡排序可能更合适。
- **大规模数据**:快速排序、归并排序或堆排序等算法更合适。
- **稳定排序**:归并排序和插入排序是稳定的算法。
### 3.1.2 理解算法的时间复杂度并非一成不变
时间复杂度是衡量算法性能的一个重要指标,但它并非在所有情况下都是决定性的。不同的排序算法,在不同的数据分布下,其实际表现可能会有较大差异。
例如,尽管归并排序在理论上有O(n log n)的时间复杂度,但在实际操作中,由于其
```
0
0
相关推荐
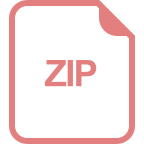
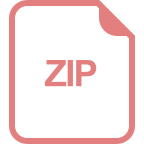
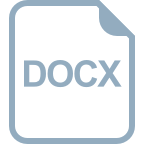
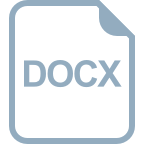