Flutter中的状态管理与数据传递
发布时间: 2024-01-18 02:31:43 阅读量: 11 订阅数: 11 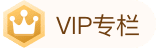
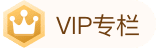
# 1. 理解Flutter中的状态管理
在Flutter中,状态管理是应用程序开发中至关重要的一个方面。状态管理涉及到如何跟踪和管理应用程序的数据和状态,并将其传递给需要使用它们的部分。在本章中,我将介绍什么是状态管理以及为什么它在Flutter中如此重要。
### 1.1 什么是状态管理?
状态管理是指在应用程序中维护和管理数据的过程。当用户与应用程序交互时,应用程序的数据和状态可能会发生变化。状态管理的目的是确保应用程序的各个部分(如UI组件)能够获取到最新的数据,并且能够根据数据的变化进行相应的操作和展示。
### 1.2 Flutter中的状态管理方式
在Flutter中,有多种方式可以进行状态管理,每种方式都有各自的优点和适用场景。以下是Flutter中常用的状态管理方式:
- StatefulWidget和StatelessWidget:Flutter中的基本组件,StatefulWidget可以管理自己的状态并重新渲染UI。
- setState()方法:通过调用setState()方法,可以通知Flutter框架更新UI,并重新构建相关的组件。
- InheritedWidget:一种特殊的Widget,可以在组件树中共享和传递数据。
### 1.3 状态管理的重要性
良好的状态管理是构建高效、可维护和可扩展的Flutter应用程序的关键。正确管理应用程序的状态能够提高应用程序的性能,减少不必要的重绘和渲染操作。同时,良好的状态管理也能够增强代码的可读性和可维护性,使开发者更容易理解和修改代码。
在接下来的章节中,我们将具体探讨Flutter中各种状态管理方式的使用方法和最佳实践。通过学习和掌握这些技术,您将能够更好地处理Flutter应用程序的状态管理和数据传递。
# 2. 基本的状态管理技术
在Flutter应用程序开发过程中,状态管理是至关重要的方面。理解Flutter中的基本状态管理技术对于构建可靠的应用至关重要。本章将介绍Flutter中的基本状态管理技术,包括StatefulWidget和StatelessWidget的区别、setState()方法的使用,以及InheritedWidget的用法。让我们逐一深入了解这些技术。
### 2.1 StatefulWidget和StatelessWidget
在Flutter中,Widget是构建用户界面的基本单元。StatelessWidget是一种不可变的Widget,它在构建后不会再被修改。而StatefulWidget则是可变的Widget,它在构建后可以根据需要重新构建。
```dart
// 示例代码:StatelessWidget和StatefulWidget的使用
class MyStatelessWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
child: Text('This is a Stateless Widget'),
);
}
}
class MyStatefulWidget extends StatefulWidget {
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Container(
child: Column(
children: <Widget>[
Text('You have pushed the button this many times:'),
Text('$_counter'),
],
),
);
}
}
```
在上面的示例中,MyStatelessWidget是一个简单的无状态Widget,而MyStatefulWidget是一个有状态Widget,它可以动态更新状态及界面。
### 2.2 setState()方法
在Flutter中,如果想要更新UI以反映应用的新状态,可以使用setState()方法。当调用setState()时,Flutter会自动调用build()方法来重新构建UI。
```dart
// 示例代码:使用setState()方法更新UI
class MyStatefulWidget extends StatefulWidget {
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
// UI部分省略
}
}
```
在上面的示例中,当调用_incrementCounter()方法时,使用setState()方法更新_counter的值,Flutter会自动调用build()方法来重新构建UI,从而更新界面显示的计数值。
### 2.3 InheritedWidget的用法
InheritedWidget是Flutter中用于在Widget树中共享数据的一种特殊Widget。它在父Widget中包装一些共享的数据,并允许子Widget访问这些数据。
```dart
// 示例代码:使用InheritedWidget共享数据
class MyInheritedWidget extends InheritedWidget {
final int myValue;
MyInheritedWidget({Key key, this.myValue, Widget child})
: super(key: key, child: child);
static MyInheritedWidget of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<MyInheritedWidget>();
}
@override
bool updateShouldNotify(MyInheritedWidget oldWidget) {
return myValue != oldWidget.myValue;
}
}
class MyInheritedWidgetExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MyInheritedWidget(
myValue: 42,
child: Builder(
builder: (BuildContext context) {
return Text('The answer is: ${MyInheritedWidget.of(context).myValue}');
},
),
);
}
}
```
在上面的示例中,MyInheritedWidget将myValue数据共享给其子Widget,在MyInheritedWidgetExample中可以通过MyInheritedWidget.of(context)
0
0
相关推荐
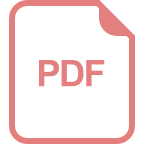
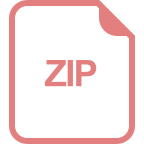
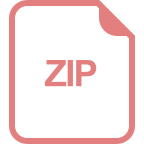





