ConstraintLayout的高级特性及应用实践
发布时间: 2024-01-18 02:06:43 阅读量: 31 订阅数: 44 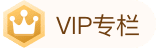
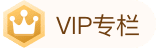
# 1. 理解ConstraintLayout的基本概念
ConstraintLayout是Android中强大的布局管理器,它可以帮助开发人员在Android应用中创建灵活且复杂的布局。本章将深入介绍ConstraintLayout的基本概念,包括其作用和优势、常用的布局属性以及基本使用方法。
## 1.1 ConstraintLayout的作用和优势
ConstraintLayout旨在简化Android布局的创建过程,提供了强大的约束特性,以便更好地管理视图之间的关系。相比于传统的布局管理器,ConstraintLayout具有以下优势:
- 灵活性:可以轻松实现复杂的布局结构,避免嵌套布局的复杂性。
- 响应式布局:适应不同尺寸的屏幕和设备,提供一致的用户体验。
- 性能优化:减少布局层次,提高渲染性能和启动速度。
## 1.2 ConstraintLayout中常用的布局属性
在ConstraintLayout中,常用的布局属性包括但不限于:
- layout_constraintLeft_toLeftOf:将视图的左侧约束到另一个视图的左侧
- layout_constraintTop_toTopOf:将视图的顶部约束到另一个视图的顶部
- layout_constraintRight_toRightOf:将视图的右侧约束到另一个视图的右侧
- layout_constraintBottom_toBottomOf:将视图的底部约束到另一个视图的底部
- layout_constraintHorizontal_bias:水平方向对齐的偏移量
- layout_constraintVertical_bias:垂直方向对齐的偏移量
- layout_constraintDimensionRatio:宽高比约束
## 1.3 ConstraintLayout的基本使用方法
基本使用方法如下所示:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, ConstraintLayout!"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent"/>
</androidx.constraintlayout.widget.ConstraintLayout>
```
上述代码展示了一个简单的ConstraintLayout布局,其中一个TextView被约束到父布局的四个边缘,实现了自适应屏幕的效果。通过设置布局属性,可以轻松实现各种复杂的布局结构。
接下来,我们将探讨ConstraintLayout中的高级约束特性。
# 2. ConstraintLayout中的高级约束特性
在上一章中,我们介绍了ConstraintLayout的基本概念和使用方法。在本章中,我们将深入探讨ConstraintLayout的一些高级约束特性,包括属性约束、环境约束和连接器。
### 2.1 属性约束(Attribute Constraints)
属性约束是指通过设置控件的属性值来实现约束效果。在ConstraintLayout中,我们可以使用特定的属性来控制控件之间的位置关系和大小。
首先,我们需要了解一些常用的属性约束:
- `layout_constraintStart_toStartOf`:将当前控件的起始边与目标控件的起始边对齐。
- `layout_constraintEnd_toEndOf`:将当前控件的结束边与目标控件的结束边对齐。
- `layout_constraintTop_toTopOf`:将当前控件的顶部边与目标控件的顶部边对齐。
- `layout_constraintBottom_toBottomOf`:将当前控件的底部边与目标控件的底部边对齐。
- `layout_constraintBaseline_toBaselineOf`:将当前控件的文本基线与目标控件的文本基线对齐。
除了以上约束属性外,我们还可以使用属性值为百分比的方式来控制控件的位置。例如,设置`layout_constraintHorizontal_bias`属性为0.3,表示控件在水平方向上相对于父容器的起点偏移30%的位置。
下面是一个示例代码,演示了属性约束的使用:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/btn1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintEnd_toStartOf="@id/btn2"
app:layout_constraintBottom_toBottomOf="@id/btn2" />
<Button
android:id="@+id/btn2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2"
app:layout_constraintStart_toEndOf="@id/btn1"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
上述代码中,我们创建了两个按钮控件,并使用属性约束来实现控件之间的位置关系。其中,`btn1`位于父容器的起点,`btn2`位于`btn1`的右侧,并占满剩余的空间。
### 2.2 环境约束(Context Constraints)
除了直接约束控件之间的位置关系外,我们还可以使用环境约束来指定控件在特定上下文(Context)中的布局规则。这样,无论控件在何种环境下都能保持一致的布局效果。
在ConstraintLayout中,我们可以使用`layout_constraintWidth_default`和`layout_constraintHeight_default`属性来设置控件的布局规则。这两个属性的取值可以为`wrap`, `match`或`spread`,分别对应自适应内容、填满父容器和均分父容器三种布局模式。
以下是一个示例代码,演示了环境约束的使用:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/btn1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1"
app:layout_constraintWidth_default="wrap"
app:layout_constraintHeight_default="wrap"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/btn2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button 2"
app:layout_constraintWidth_default="spread"
app:layout_constraintHeight_default="wrap"
app:layout_constraintTop_toBottomOf="@id/btn1"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
上述代码中,`btn1`的布局规则为自适应内容,而`btn2`的布局规则为填满父容器。无论是在何种上下文中,这两个按钮都能保持一致的布局效果。
### 2.3 连接器(Connectors)
连接器是ConstraintLayout中的一个重要特性,它允许我们将控件的属性进行连接,并通过代码动态地改变这些连接关系。
在ConstraintLayout中,我们可以通过设置`layout_constraintHorizontal_chainStyle`属性和`layout_constraintVertical_chainStyle`属性来定义控件之间的链式布局(Chain Layout)。链式布局是指将多个控件以链条的形式连接起来,形成一个整体。
以下是一个示例代码,演示了连接器的使用:
```xml
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/btn1"
android:layout_width="wrap_content"
android:layout_height="wrap_
```
0
0
相关推荐
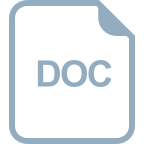
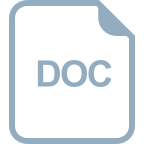
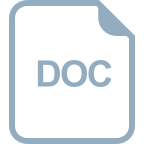
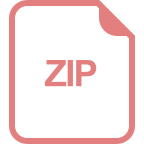
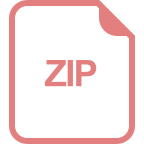
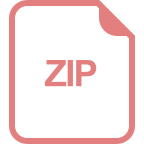
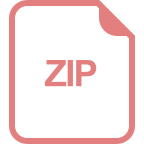
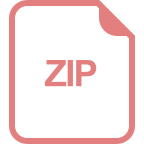
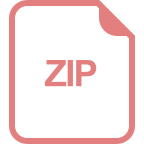