Unveiling MySQL Deadlock Issues: How to Analyze and Completely Solve
发布时间: 2024-09-13 19:47:07 阅读量: 14 订阅数: 23 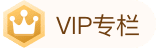
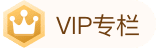
# Demystifying MySQL Deadlocks: How to Analyze and Completely Resolve Them
## 1. The Essence and Causes of MySQL Deadlocks
A deadlock is a concept in computer science that refers to a state where two or more processes are stuck in a situation where each is waiting for the other to release resources, causing the system to grind to a halt. In MySQL, deadlocks typically occur when multiple transactions simultaneously update the same row or multiple rows, with each transaction holding locks on one or more rows and waiting for other transactions to release their locks before continuing.
The causes of deadlocks mainly include:
- **Concurrent Access:** When multiple transactions access the same resource simultaneously, a deadlock may occur.
- **Resource Dependence:** When a transaction needs to wait for a resource released by another transaction, a deadlock may occur.
- **Sequential Access:** When multiple transactions access resources in the same order, a deadlock may occur.
## 2. MySQL Deadlock Analysis and Diagnosis
### 2.1 Deadlock Detection and Monitoring Tools
#### 2.1.1 SHOW PROCESSLIST Command
The SHOW PROCESSLIST command displays information about threads currently executing, including thread ID, status, statement being executed, etc. With this command, we can check for deadlock threads and their specific states.
```sql
SHOW PROCESSLIST;
```
*Parameter Explanation:*
- **Id:** Thread ID
- **User:** User
- **Host:** Host
- **db:** Current database
- **Command:** Current command being executed
- **Time:** Execution time
- **State:** Thread state, including:
* Running: Executing
* Sleeping: Dormant
* Waiting for lock: Waiting for a lock
* Locked: Locked
- **Info:** Other information, such as the lock resource being waited for
*Code Logic Analysis:*
This command queries the system table to obtain information about the threads currently executing and presents it in table format. By examining the thread's state, we can determine if there are any deadlock threads.
#### 2.1.2 INFORMATION_SCHEMA.INNODB_TRX Table
The INFORMATION_SCHEMA.INNODB_TRX table stores information about currently executing transactions, including transaction ID, status, and the lock resources being waited for. This table can be used to further analyze specific deadlock situations.
```sql
SELECT * FROM INFORMATION_SCHEMA.INNODB_TRX;
```
*Parameter Explanation:*
- **TRX_ID:** Transaction ID
- **TRX_STATE:** Transaction state, including:
* RUNNING: Executing
* COMMITTED: Committed
* ROLLED BACK: Rolled back
- **TRX_STARTED:** Transaction start time
- **TRX_ISOLATION_LEVEL:** Transaction isolation level
- **TRX_READ_ONLY:** Whether the transaction is read-only
- **TRX_LOCK_MODE:** Transaction lock mode
- **TRX_AUTOCOMMIT:** Whether the transaction is in autocommit mode
- **TRX_FOREIGN_KEY_CHECKS:** Whether foreign key checks are enabled
- **TRX_UNIQUE_CHECKS:** Whether uniqueness checks are enabled
- **TRX_WAIT_STARTED:** Time waiting started
- **TRX_WAIT_REASON:** Reason for waiting, including:
* LOCK WAIT: Waiting for a lock
* COMMIT WAIT: Waiting for a commit
* ROLLBACK WAIT: Waiting for a rollback
- **TRX_WAIT_ROW_LOCKS:** Number of row locks being waited for
- **TRX_WAIT_TABLE_LOCKS:** Number of table locks being waited for
- **TRX_WAIT_DEADLOCK:** Whether waiting for a deadlock
*Code Logic Analysis:*
This command queries the system table to obtain information about currently executing transactions and presents it in table format. By examining the transaction's state and reason for waiting, we can determine if there are any deadlock transactions.
### 2.2 Deadlock Analysis Methods
#### 2.2.1 Wait-for Graph Analysis
A wait-for graph is a visualization tool that shows the waiting relationships between threads. Through the wait-for graph, we can visually see the waiting conditions of deadlock threads and analyze the root causes of deadlocks.
**Mermaid Flowchart:**
```mermaid
graph LR
subgraph Deadlock
A[Thread A] -->|waits for| B[Thread B]
B[Thread B] -->|waits for| C[Thread C]
C[Thread C] -->|waits for| A[Thread A]
end
```
*Code Logic Analysis:*
The flowchart illustrates a deadlock scenario where Thread A is waiting for Thread B to release a lock, Thread B is waiting for Thread C to release a lock, and Thread C is waiting for Thread A to release a lock. This cyclical waiting relationship results in a deadlock.
#### 2.2.2 Transaction Log Analysis
Transaction logs record all transaction operations in the database. By analyzing transaction logs, we can understand the sequence and timing of transactions, thereby determining when and why a deadlock occurred.
**Code Block:**
```sql
SELECT * FROM mysql.general_log WHERE command_type = 'Query' ORDER BY event_time DESC;
```
*Parameter Explanation:*
- **command_type:** Command type
- **event_time:** Event time
*Code Logic Analysis:*
This command queries all log records of the Query command type from the mysql.general_log table and sorts them in reverse chronological order. By examining the log records, we can understand the sequence and timing of transactions, thereby determining when and why a deadlock occurred.
## 3.1 Deadlock Prevention Strategies
0
0
相关推荐
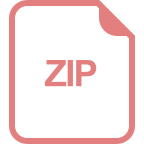
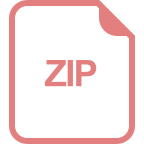
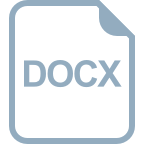
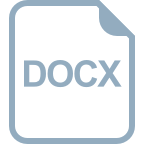