Redis Caching Mechanism Unveiled: From Data Structures to Eviction Strategies
发布时间: 2024-09-13 19:55:32 阅读量: 37 订阅数: 27 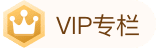
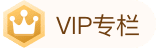
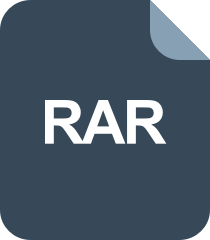
redis可视化工具:RedisDesktopManager
# Redis Caching Mechanism Unveiled: From Data Structures to Eviction Strategies
Redis is a popular in-memory database renowned for its high performance and rich feature set. It is widely used for caching to enhance application responsiveness and reduce database load.
The core idea behind Redis's caching mechanism is to store frequently accessed data in memory, thereby avoiding slower access to persistent storage (such as disks). When an application needs to access data, it first checks if the data is present in the cache. If it exists, the data is retrieved directly from the cache, significantly speeding up the access time. If it does not exist, the application fetches the data from the persistent storage and adds it to the cache for future access.
# Redis Data Structures
Redis offers a variety of data structures, each with its unique features and purposes. Understanding these data structures is crucial for effectively utilizing Redis.
### 2.1 String Type
The string type is the most basic data structure, capable of storing binary-safe data. The string type supports the following operations:
- **SET**: Sets a key-value pair
- **GET**: Retrieves a value by key
- **APPEND**: Appends data to the end of an existing value
- **INCR**: Increments the value by a specified amount
- **DECR**: Decrements the value by a specified amount
```python
# Sets a key-value pair
redis.set("name", "John Doe")
# Retrieves a value by key
value = redis.get("name")
# Appends data to the end of an existing value
redis.append("name", " (Software Engineer)")
# Increments the value by a specified amount
redis.incr("age", 1)
# Decrements the value by a specified amount
redis.decr("age", 1)
```
### 2.2 List Type
The list type is an ordered collection capable of storing multiple values. The list type supports the following operations:
- **LPUSH**: Adds one or more values to the beginning of a list
- **RPUSH**: Adds one or more values to the end of a list
- **LPOP**: Removes and returns a value from the beginning of a list
- **RPOP**: Removes and returns a value from the end of a list
- **LINDEX**: Retrieves the value at a specified index in a list
```python
# Adds one or more values to the beginning of a list
redis.lpush("fruits", "apple", "banana", "orange")
# Adds one or more values to the end of a list
redis.rpush("fruits", "grape", "strawberry")
# Removes and returns a value from the beginning of a list
fruit = redis.lpop("fruits")
# Removes and returns a value from the end of a list
fruit = redis.rpop("fruits")
# Retrieves the value at a specified index in a list
fruit = redis.lindex("fruits", 2)
```
### 2.3 Set Type
The set type is an unordered collection capable of storing unique elements. The set type supports the following operations:
- **SADD**: Adds one or more members to a set
- **SMEMBERS**: Retrieves all members in a set
- **SREM**: Removes one or more members from a set
- **SCARD**: Retrieves the number of members in a set
- **SINTER**: Retrieves the intersection of two or more sets
```python
# Adds one or more members to a set
redis.sadd("programming_languages", "Python", "Java", "C++")
# Retrieves all members in a set
members = redis.smembers("programming_languages")
# Removes one or more members from a set
redis.srem("programming_languages", "C++")
# Retrieves the number of members in a set
count = redis.scard("programming_languages")
# Retrieves the intersection of two or more sets
intersection = redis.sinter("programming_languages", "web_frameworks")
```
### 2.4 Hash Type
The hash type is a collection of key-value pairs where the keys are strings and the values can be any type. The hash type supports the following operations:
- **HSET**: Sets a key-value pair within the hash
- **HGET**: Retrieves the value for a specified key in the hash
- **HGETALL**: Retrieves all key-value pairs in the hash
- **HDEL**: Deletes one or more key-value pairs from the hash
- **HKEYS**: Retrieves all keys in the hash
- **HVALS**: Retrieves all values in the hash
```python
# Sets a key-value pair within the hash
redis.hset("user:1", "name", "John Doe")
redis.hset("user:1", "age", 30)
# Retrieves the value for a specified key in the hash
name = redis.hget("user:1", "name")
# Retrieves all key-value pairs in the hash
data = redis.hgetall("user:1")
# Deletes one or more key-value pairs from the hash
redis.hdel("user:1", "age")
# Retrieves all keys in the hash
keys = redis.hkeys("user:1")
# Retrieves all values in the hash
values = redis.hvals("user:1")
```
# Redis Caching Eviction Policies
Caching eviction policies dictate which cached data will be evicted when the cache space is insufficient. Redis offers a variety of eviction strategies to meet different application scenarios and performance requirements.
### 3.1 LRU (Least Recently Used)
The LRU (Least Recently Used) algorithm evicts the least recently used cached data. It maintains a doubly linked list where the most recently used cached data is at the head of the list, and the least recently used data is at the tail. When cache space runs out, the data at the tail of the list is evicted.
```python
# Implementing LRU cache using LRUCache
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
```
0
0
相关推荐
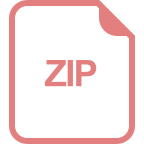
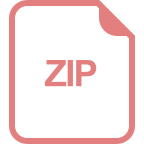
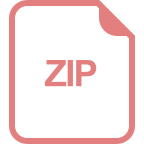
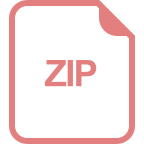
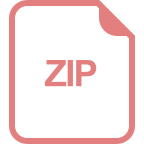
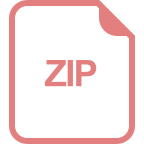