【WeakHashMap内存管理】:Java垃圾回收与内存管理的秘密武器
发布时间: 2024-09-11 02:57:38 阅读量: 67 订阅数: 26 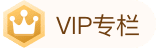
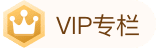
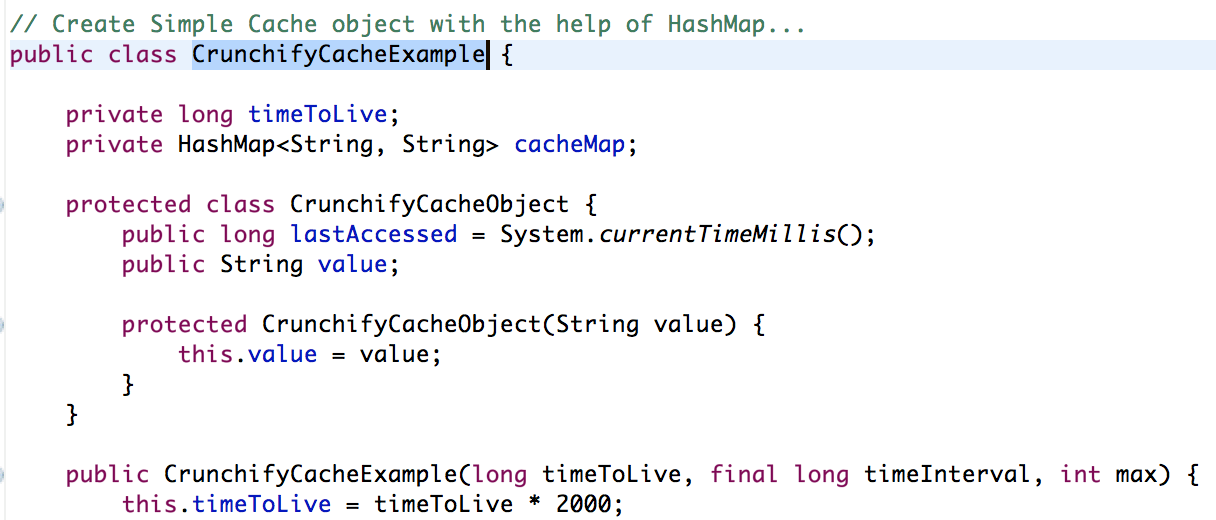
# 1. WeakHashMap简介与内存管理基础
## 1.1 WeakHashMap简介
Java中的`WeakHashMap`是一种基于哈希表的Map接口实现,它具有一个特殊的行为:当其键(key)所引用的对象不再被其他对象引用时,这些键值对会被自动地从映射中移除。这一点与普通的`HashMap`相比,后者需要用户自行调用`remove`方法来删除不再使用的条目。
`WeakHashMap`非常适用于那些不希望键值对永久占用内存的场景。例如,在实现缓存系统时,当外部数据源的对象被废弃或更新时,我们可能希望内存中的映射也随之清除。
## 1.2 内存管理基础
在理解`WeakHashMap`的工作机制之前,有必要先了解Java的内存管理基础。Java内存管理主要涉及堆(Heap)内存和非堆(Non-Heap)内存,其中堆内存是运行时数据区域,所有的对象实例和数组都在这里分配内存。而非堆内存包括方法区、直接内存等。
在Java中,垃圾回收(GC)主要负责回收堆内存中不再使用的对象,释放内存空间。在`WeakHashMap`的情况下,即使它的键不再被其他对象引用,也会在GC运行时被标记为可回收的对象。
```java
// 示例代码
WeakHashMap<WeakReference<String>, Object> weakMap = new WeakHashMap<>();
String key = new String("key");
Object value = new Object();
weakMap.put(new WeakReference<>(key), value);
key = null; // key不再被其他对象引用
System.gc(); // 手动触发垃圾回收,提示JVM进行垃圾回收
```
在上面的代码中,如果`key`对象不再有其他强引用指向它,那么在下一次垃圾回收过程中,该键值对可能会被`WeakHashMap`的实现自动移除。需要注意的是,`System.gc()`方法只是一种提示,并不能保证JVM会立即执行垃圾回收。
# 2. WeakHashMap的内部原理详解
### 2.1 WeakHashMap的数据结构
#### 2.1.1 Entry节点的设计
在Java集合框架中,`WeakHashMap`是一种基于哈希表的Map实现,它对于键采用弱引用。为了深入理解`WeakHashMap`的工作原理,我们首先需要探讨其数据结构,尤其是`Entry`节点的设计。
`Entry`节点是`WeakHashMap`中的基本单元,它继承自`HashMap.Node`类,并且有自己的特殊属性。一个典型的`Entry`节点包含四个字段:`key`、`value`、`hash`和`next`。其中,`key`是一个弱引用(WeakReference),指向用户指定的键对象,而`value`则是实际存储的值。`hash`字段存储键的哈希码,`next`是一个指针,用于解决哈希冲突问题。
```java
static class Entry<K,V> extends WeakReference<Object> implements Map.Entry<K,V> {
V value;
final int hash;
Entry<K,V> next;
Entry(Object key, V value,
ReferenceQueue<Object> queue,
int hash, Entry<K,V> next) {
super(key, queue);
this.value = value;
this.hash = hash;
this.next = next;
}
}
```
在上述代码中,`Entry`类的构造器接受键对象、值对象、引用队列、哈希码和下一个节点作为参数。通过继承`WeakReference`,`WeakHashMap`能够监听键对象的垃圾回收事件。
#### 2.1.2 引用队列的作用与实现
`WeakReference`对象被封装在`WeakHashMap`中的`Entry`节点内,其主要目的是让键对象可以被垃圾回收器回收。为了感知键对象的回收事件,`WeakHashMap`内部使用了一个`ReferenceQueue`。
`ReferenceQueue`是一个线程安全的队列,用于存储等待回收的`WeakReference`对象。当垃圾回收器决定回收一个被`WeakReference`引用的对象时,该`WeakReference`对象会被加入到这个队列中。`WeakHashMap`通过定期检查这个队列来得知哪些键已经被回收,并相应地清理映射中的键值对。
```java
ReferenceQueue<Object> queue = new ReferenceQueue<>();
```
在上述代码中,创建了一个`ReferenceQueue`实例,它将用于接收被回收的键对象对应的`WeakReference`。`WeakHashMap`的实现中会轮询这个队列,处理被回收的条目,即当某个键的弱引用被回收后,对应的键值对也会从`WeakHashMap`中被移除。
### 2.2 WeakHashMap的键值对机制
#### 2.2.1 键的弱引用特性
`WeakHashMap`的核心特性在于其键的弱引用。与普通的`HashMap`相比,其键对象不是直接存储在映射中,而是通过弱引用(`WeakReference`)持有。
弱引用是一种特殊的引用,它不会阻止其所引用的对象被垃圾回收。换句话说,即使存在弱引用,当没有强引用指向一个对象时,该对象可能会被垃圾回收器回收。
在`WeakHashMap`中,键对象作为弱引用存储,因此一旦键对象变得不可达,即使映射本身还存在,该键对象也可能被垃圾回收。这种设计允许`WeakHashMap`用作缓存,自动释放不再使用的缓存项。
#### 2.2.2 值的存储与管理
与键的弱引用不同,`WeakHashMap`中的值并不通过弱引用存储。这表明即使键被回收,只要对应的值对象没有其他强引用指向,其生命周期完全依赖于其是否被映射本身或其他对象引用。
值对象直接存储在`Entry`节点中,因此它们遵循普通的垃圾回收规则。只有当值对象没有任何强引用指向时,垃圾回收器才会回收它。
### 2.3 WeakHashMap的垃圾回收流程
#### 2.3.1 JVM垃圾回收机制概述
在Java虚拟机(JVM)中,垃圾回收(GC)是自动管理内存的主要方式。JVM的垃圾回收器负责追踪、标记和回收不再被任何强引用所指向的对象,释放内存资源给新的对象使用。
垃圾回收机制通常分为几种不同的算法,包括标记-清除(Mark-Sweep)、复制(Copying)、标记-整理(Mark-Compact)和分代收集(Generational Collection)。分代收集是最常用的,它根据对象的存活时间将内存分为不同代,如新生代(Young Generation)和老年代(Old Generation),不同代使用不同的垃圾回收算法。
#### 2.3.2 WeakHashMap中对象的回收时机
在`WeakHashMap`中,键的弱引用对象的回收时机依赖于垃圾回收器的工作。一旦JVM确定某个键对象不再被任何强引用所指向,该键对象就处于可被回收的状态。
当键对象被回收后,`WeakHashMap`通过检查`ReferenceQueue`来感知到这一事件。此时,`WeakHashMap`会清除与被回收键相关的键值对。这个过程不是实时的,它依赖于`WeakHashMap`的`finalize`方法的调用,或者其`poll`方法的主动检查。因此,直到下一次访问或修改映射时,或者通过显式调用`poll`方法,被回收的键值对才会被真正移除。
这个机制意味着,对于开发者来说,`WeakHashMap`提供了自动的内存管理能力,允许缓存或映射只保留在内存中,当键不再被使用时,它们将自动被回收,从而避免内存泄漏。
# 3. WeakHashMap在实践中的应用
## 3.1 缓存机制中的应用案例
### 3.1.1 缓存的有效期与清除策略
在许多应
0
0
相关推荐
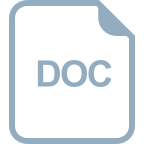
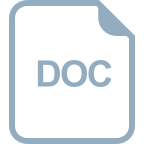
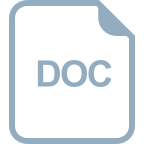
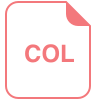
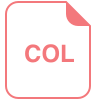
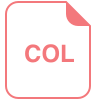
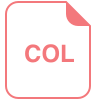
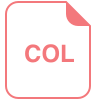
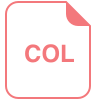