线性搜索在数据结构中的妙用:解锁数据查找的利器
发布时间: 2024-08-25 12:08:02 阅读量: 21 订阅数: 24 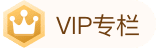
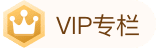
# 1. 线性搜索的概念和原理
线性搜索是一种基本的数据查找算法,它通过逐个检查数据结构中的元素来查找目标元素。线性搜索的原理很简单,它从数据结构的第一个元素开始,依次检查每个元素,直到找到目标元素或遍历完整个数据结构。
线性搜索的复杂度为 O(n),其中 n 是数据结构中的元素数量。这意味着随着数据结构中元素数量的增加,线性搜索的时间复杂度也会线性增加。在数据结构较小的情况下,线性搜索是一种简单有效的查找算法。
# 2. 线性搜索的算法实现
### 2.1 顺序搜索
顺序搜索是一种最简单的线性搜索算法,它从列表或数组的第一个元素开始,逐个元素地比较,直到找到目标元素或遍历完整个列表。
```python
def sequential_search(arr, target):
"""
顺序搜索算法
参数:
arr: 待搜索的列表或数组
target: 要查找的目标元素
返回:
目标元素在列表中的索引,如果未找到则返回 -1
"""
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
```
**逻辑分析:**
* `for` 循环遍历列表中的每个元素。
* 对于每个元素,检查它是否等于目标元素。
* 如果找到目标元素,返回其索引。
* 如果遍历完整个列表仍未找到目标元素,返回 -1。
### 2.2 二分搜索
二分搜索是一种更高级的线性搜索算法,它适用于有序列表或数组。它通过将列表或数组分成两半并递归地搜索目标元素来工作。
```python
def binary_search(arr, target):
"""
二分搜索算法
参数:
arr: 待搜索的有序列表或数组
target: 要查找的目标元素
返回:
目标元素在列表中的索引,如果未找到则返回 -1
"""
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
```
**逻辑分析:**
* `low` 和 `high` 变量分别表示列表或数组的左边界和右边界。
* `while` 循环在 `low` 小于或等于 `high` 时执行。
* 每个循环迭代中,计算列表或数组的中间索引 `mid`。
* 检查 `arr[mid]` 是否等于目标元素。
* 如果相等,返回 `mid`。
* 如果 `arr[mid]` 小于目标元素,则将 `low` 设置为 `mid + 1`,缩小搜索范围。
* 如果 `arr[mid]` 大于目标元素,则将 `high` 设置为 `mid - 1`,缩小搜索范围。
* 如果 `while` 循环结束时未找到目标元素,返回 -1。
# 3. 线性搜索的应用场景
### 3.1 无序数据的查找
线性搜索在无序数据中查找元素时非常简单。它从数组的第一个元素开始,逐个比较,直到找到目标元素或遍历完整个数组。
```python
def linear_search(arr, target):
"""
在无序数组中查找目标元素
参数:
arr (list): 无序数组
target (any): 要查找的目标元素
返回:
int: 目标元素在数组中的索引,如果未找到则返回 -1
"""
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
```
**逻辑分析:**
* 函数 `linear_search` 接受两个参数:无序数组 `arr` 和要查找的目标元素 `target`。
* 它遍历数组,逐个比较每个元素是否等于 `target`。
* 如果找到匹配项,函数返回该元素的索引。
* 如果遍历完整个数组都没有找到匹配项,函数返回 -1。
### 3.2 有序数据的查找(二分搜索)
当数据是有序时,可以使用二分搜索来提高查找效率。二分搜索通过将搜索空间不断减半,从而以对数时间复杂度查找目标元素。
```python
def binary_search(arr, target):
"""
在有序数组中查找目标元素
参数:
arr (list): 有序数组
target (any): 要查找的目标元素
返回:
int: 目标元素在数组中的索引,如果未找到则返回 -1
"""
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
```
**逻辑分析:**
* 函数 `binary_search` 接受两个参数:有序数组 `arr` 和要查找的目标元素 `target`。
* 它使用两个指针 `low` 和 `high` 来表示搜索空间的范围。
* 函数不断计算中间索引 `mid`,并比较 `arr[mid]` 与 `target`。
* 如果匹配,函数返回 `mid`。
* 如果 `arr[mid]` 小于 `target`,则搜索空间移动到 `[mid + 1, high]`。
* 如果 `arr[mid]` 大于 `target`,则搜索空间移动到 `[low, mid - 1]`。
* 算法重复上述步骤,直到找到 `target` 或搜索空间为空。
**表格:线性搜索与二分搜索的比较**
| 特征 | 线性搜索 | 二分搜索 |
|---|---|---|
| 时间复杂度 | O(n) | O(log n) |
| 数据要求 | 无序或有序 | 有序 |
| 查找效率 | 低 | 高 |
# 4. 线性搜索的优化技巧
### 4.1 数据预处理
数据预处理是指在进行线性搜索之前,对数据进行一定的处理,以提高搜索效率。常见的数据预处理技术包括:
**排序:**将数据按照某种顺序排列,如升序或降序。排序后的数据可以利用二分搜索算法进行快速查找。
**哈希表:**将数据以键值对的形式存储在哈希表中。哈希表可以根据键值快速查找数据,时间复杂度为 O(1)。
### 4.2 索引的使用
索引是一种数据结构,它将数据项与一个或多个关键字关联起来。通过索引,可以快速定位数据项,从而提高搜索效率。
**B-树:**一种平衡搜索树,它可以高效地查找和插入数据。B-树通常用于数据库中,可以将数据搜索时间复杂度降低到 O(log n)。
**红黑树:**一种自平衡二叉搜索树,它具有良好的性能和时间复杂度为 O(log n) 的查找操作。
### 4.3 分治法
分治法是一种递归算法,它将一个大问题分解成多个小问题,然后递归地解决这些小问题。分治法可以用于线性搜索的优化,通过将数据分成较小的块,并并行搜索这些块,从而提高搜索效率。
**代码示例:**
```python
def parallel_linear_search(arr, target):
"""
使用分治法并行进行线性搜索。
参数:
arr: 要搜索的数组
target: 要查找的目标值
返回:
目标值在数组中的索引,如果没有找到则返回 -1
"""
# 检查数组是否为空
if not arr:
return -1
# 将数组分成两部分
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
# 并行搜索两个部分
left_result = parallel_linear_search(left_half, target)
right_result = parallel_linear_search(right_half, target)
# 如果在左半部分找到目标值,则返回索引
if left_result != -1:
return left_result
# 如果在右半部分找到目标值,则返回索引
if right_result != -1:
return mid + right_result
# 如果在两个部分都没有找到目标值,则返回 -1
return -1
```
**逻辑分析:**
该代码使用分治法并行进行线性搜索。它将数组分成两部分,然后并行搜索这两个部分。如果在任何一个部分找到目标值,则返回索引。如果在两个部分都没有找到目标值,则返回 -1。这种方法可以提高线性搜索的效率,尤其是在数组较大时。
# 5.1 查找最值
线性搜索还可以用于查找数据中的最值,例如最大值或最小值。
```python
def find_max(arr):
"""
查找数组中的最大值
参数:
arr: 输入数组
返回:
数组中的最大值
"""
max_value = arr[0]
for i in range(1, len(arr)):
if arr[i] > max_value:
max_value = arr[i]
return max_value
```
```python
def find_min(arr):
"""
查找数组中的最小值
参数:
arr: 输入数组
返回:
数组中的最小值
"""
min_value = arr[0]
for i in range(1, len(arr)):
if arr[i] < min_value:
min_value = arr[i]
return min_value
```
## 5.2 查找重复元素
线性搜索还可以用于查找数据中重复出现的元素。
```python
def find_duplicates(arr):
"""
查找数组中重复出现的元素
参数:
arr: 输入数组
返回:
一个包含重复元素的列表
"""
duplicates = []
for i in range(len(arr)):
for j in range(i + 1, len(arr)):
if arr[i] == arr[j]:
duplicates.append(arr[i])
return duplicates
```
## 5.3 查找特定模式
线性搜索还可以用于查找数据中是否存在特定的模式或子序列。
```python
def find_pattern(arr, pattern):
"""
查找数组中是否存在特定的模式
参数:
arr: 输入数组
pattern: 要查找的模式
返回:
如果模式存在,返回 True,否则返回 False
"""
for i in range(len(arr) - len(pattern) + 1):
if arr[i:i+len(pattern)] == pattern:
return True
return False
```
0
0
相关推荐
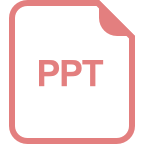
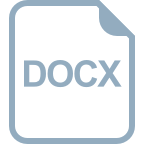
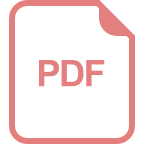
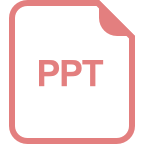
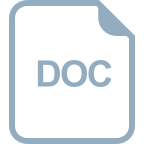
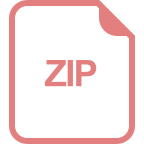
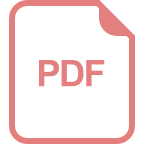
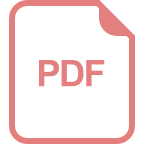