【PyTorch实战秘技】:加速Python深度学习项目的五大技巧
发布时间: 2024-08-31 08:34:58 阅读量: 231 订阅数: 74 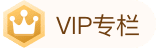
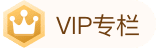
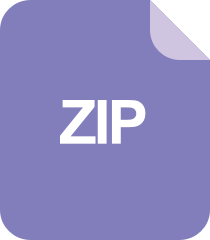
基于PyTorch的Python深度学习实战源码

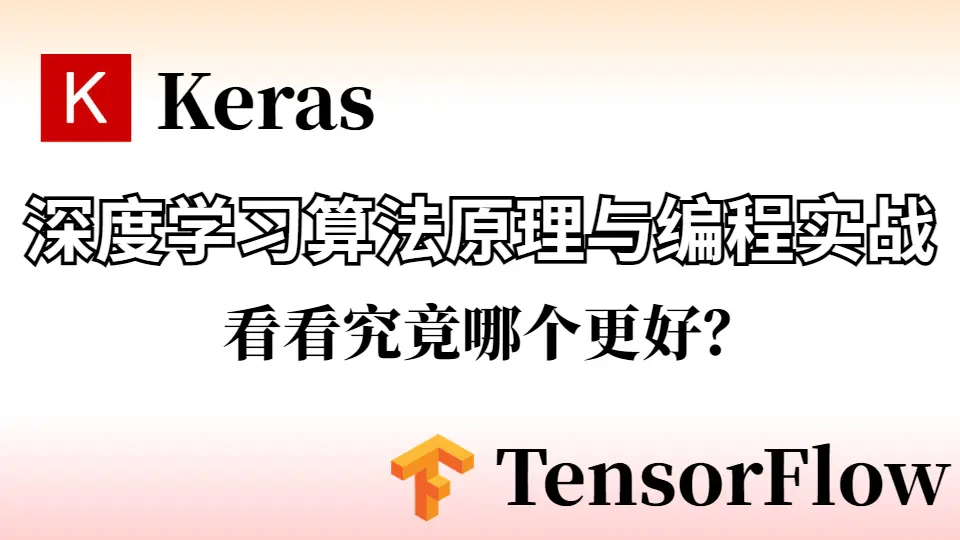
# 1. 深度学习与PyTorch简介
## 简介深度学习
深度学习是一种以多层神经网络为架构的机器学习方法,它可以自动学习数据的复杂结构,从而在图像识别、语音识别、自然语言处理等领域取得了革命性的进展。它的核心是通过非线性变换对高维数据进行特征学习,这在传统算法中难以实现。
## 什么是PyTorch
PyTorch是一个开源机器学习库,广泛应用于计算机视觉和自然语言处理等领域。它以动态计算图著称,使得构建灵活的深度神经网络变得简单。PyTorch支持GPU加速计算,可以无缝地进行大规模数据的并行处理。
## 深度学习与PyTorch的关系
PyTorch提供了一整套工具和库,用以简化深度学习模型的开发和训练过程。它直观的API设计和动态计算图机制,使得研究人员和开发者可以快速实验和迭代,从而加速了深度学习的研究和应用开发。
# 2. PyTorch基础操作优化
### 2.1 张量和操作的高效使用
#### 2.1.1 张量的基本概念
在深度学习框架PyTorch中,张量(Tensor)是基础的数据结构,可以看作是多维数组,用于存储数据并进行计算。张量和NumPy的ndarrays类似,但张量可以在GPU上加速计算,这使得它特别适合于深度学习任务。
张量的操作丰富,包括但不限于数据创建、索引、切片、数学运算、广播等。理解这些操作不仅对初学者至关重要,对资深开发者而言,掌握如何高效地使用张量也是提升程序性能的关键。
#### 2.1.2 张量运算的优化技巧
高效利用张量操作是优化PyTorch模型训练过程的关键。以下是一些核心技巧:
- **使用合适的数据类型**:利用PyTorch提供的不同数据类型,如`torch.float32`和`torch.float16`,可以根据模型需求选择内存占用更小的数据类型,提高运算速度。
- **减少不必要的内存占用**:可以使用`in-place`操作来节省内存,例如`zero_()`函数可以将一个张量的值直接置为零,而不是创建一个新的张量。
- **利用并行计算**:启用CUDA加速(GPU)可以让张量的计算更快,如果设备支持的话。
- **合并操作**:减少在Python中的循环,转而使用批量操作来处理数据。例如,如果要在张量的每一行上应用相同的函数,可以使用`torch.relu()`这类批量操作,而不是循环每行。
### 2.2 自动求导与计算图
#### 2.2.1 反向传播机制概述
自动求导是深度学习框架的核心特性之一,它极大地简化了模型训练过程。PyTorch使用动态计算图(也称为定义-运行(define-by-run)图),这意味着图是在运行代码时动态构建的。
反向传播机制是通过图的反向遍历来计算梯度的。这个过程通常分为两个阶段:
1. **前向传播**:在图中从输入节点到输出节点传递数据并执行计算。
2. **反向传播**:根据链式法则,从输出节点开始向输入节点传递梯度,计算每个节点的梯度。
#### 2.2.2 计算图的构建与优化
虽然自动求导为开发者节省了大量的手动微分工作,但构建高效计算图仍然需要一些技巧:
- **理解并优化计算图结构**:在构建模型时,应减少不必要的计算节点,从而减少内存的占用和计算的冗余。
- **利用PyTorch内置函数**:尽量使用PyTorch提供的函数而不是自己实现,因为内置函数通常经过优化。
- **使用`torch.no_grad()`**:在不需要计算梯度的情况下,使用`torch.no_grad()`可以节省内存和计算资源。
### 2.3 数据加载与批量处理
#### 2.3.1 DataLoader的高级使用方法
PyTorch中的`DataLoader`类提供了高效的数据加载方法。它可以将数据划分为小批量进行迭代,通过其`collate_fn`参数,可以对批量数据进行自定义处理,比如拼接张量、填充等。
对于特定类型的数据,例如文本或图像,可以使用`DataLoader`结合`Dataset`类来构建复杂的批量处理流程。例如,可以设计一个`Dataset`类来处理图像数据,其中可以集成加载图像、缩放、归一化等预处理步骤。
#### 2.3.2 批量处理中的内存管理
内存管理是优化PyTorch模型时的一个重要方面。在批量处理时,以下策略可以帮助管理内存:
- **使用`pin_memory=True`**:在`DataLoader`中启用`pin_memory`可以加速数据从CPU内存传输到GPU内存的过程。
- **及时清理不再使用的张量**:在Python中,垃圾回收不会立即执行,所以确保不再使用的张量(或对象)的引用被删除是很重要的。
为了深入理解批量处理中的内存管理,这里展示一个简单的`DataLoader`使用示例:
```python
import torch
from torch.utils.data import DataLoader, TensorDataset
# 创建一个简单的张量数据集
data = torch.randn(50, 20)
labels = torch.randint(0, 2, (50,))
dataset = TensorDataset(data, labels)
# 使用DataLoader进行批量处理
batch_size = 10
loader = DataLoader(dataset, batch_size=batch_size, shuffle=True, pin_memory=True)
for inputs, targets in loader:
# 假设在模型训练或评估中使用inputs和targets
pass
```
在上面的代码中,`pin_memory=True`确保数据在传输到GPU时更快。此外,`shuffle=True`确保在每次迭代时随机打乱数据,这通常有助于模型训练的稳定性和收敛速度。
上述内容仅为本章节的概览,下面将深入探讨具体细节和进阶操作。
# 3. PyTorch模型训练加速技巧
## 3.1 并行计算与多GPU训练
### 3.1.1 PyTorch中的并行计算基础
在当今的数据科学领域,数据集的规模持续增长,计算需求也愈发庞大。为了应对这一挑战,PyTorch 提供了强大的并行计算能力,尤其是在多 GPU 环境下。这一节将探讨 PyTorch 中并行计算的基础知识,为理解多 GPU 训练奠定基础。
并行计算的实现基于 PyTorch 中的 `torch.nn.DataParallel` 和 `torch.nn.parallel.DistributedDataParallel`(简称 `DDP`)。`DataParallel` 是最简单的并行计算方式,它将一个模型复制到多个 GPU 上,并将输入数据均匀地分发到各个 GPU 上进行计算。然而,这种方法可能不会充分发挥每个 GPU 的全部潜力,因为数据传输和模型复制可能会造成一定的开销。
另一方面,`DDP` 提供了一种更为高效的并行方式。它通过在每个进程上复制模型,并在不同的进程之间传递梯度来实现。相比于 `DataParallel`,`DDP` 在多个 GPU 上提供了更加细粒度的控制,减少了内存的复制,并且可以更好地扩展到多节点、多 GPU 的环境中。
在使用 `DDP` 时,要注意以下几个关键点:
- 每个进程都有自己的 GPU。
- 每个进程仅运行模型的一个副本。
- 模型的前向传播和反向传播在所有副本上并行执行。
下面是一个使用 `DDP` 进行模型训练的代码示例:
```python
import torch
import torch.distributed as dist
import torch.nn as nn
import torch.optim as optim
from torch.nn.parallel import DistributedDataParallel as DDP
def setup(rank, world_size):
# 初始化进程组
dist.init_process_group("nccl", rank=rank, world_size=world_size)
def cleanup():
# 清理进程组
dist.destroy_process_group()
def main(rank, world_size):
setup(rank, world_size)
# 假设 batch size 是 world_size 的倍数
batch_size = 100 * world_size
# 创建模型并复制到 GPU 上
model = MyModel().to(rank)
ddp_model = DDP(model, device_ids=[rank])
loss_fn = nn.CrossEntropyLoss()
optimizer = optim.SGD(ddp_model.parameters(), lr=0.001)
optimizer.zero_grad()
outputs = ddp_model(torch.randn(batch_size, 10))
labels = torch.randint(0, 10, (batch_size,))
labels = labels.to(rank)
loss_fn(outputs, labels).backward()
optimizer.step()
cleanup()
if __name__ == "__main__":
world_size = 2
torch.multiprocessing.spawn(main, args=(world_size,), nprocs=world_size, join=True)
```
### 3.1.2 多GPU训练的实施策略
在多 GPU 环境中进行训练时,选择合适的并行策略至关重要。这不仅关乎性能的提升,也影响着模型训练的可扩展性和灵活性。在此部分,我们将讨论多 GPU 训练的实施策略。
首先,要确定在多 GPU 训练中的进程分布。如果是在单个机器上,可以通过设置环境变量 `CUDA_VISIBLE_DEVICES` 来指定每个 GPU 设备。例如,有四个 GPU,分别为 0、1、2、3,则可以为每个进程分配不同的 GPU。
其次,要选择正确的并行策略。`DataParallel` 和 `DDP` 的选择依赖于具体的应用场景。`DataParallel` 简单易用,适合单机多 GPU 的快速原型设计。`DDP` 则更适合于大规模分布式训练,尤其是在多机多 GPU 的环境中。
接下来,需要考虑的是数据并行的粒度。过大的批处理大小可能导致内存不足,而过小则可能无法充分利用 GPU 的计算能力。通常需要通过实验来找到最优的批处理大小。
最后,是梯度累积(gradient accumulation)。在内存限制的情况下,可以通过梯度累积来模拟更大的批处理大小。具体做法是在多个迭代中累积梯度,然后一次性进行优化器的更新。
以下是使用梯度累积策略的一个示例:
```python
# 假设我们有一个数据加载器 `train_loader`
num累积 = 2 # 梯度累积次数
for epoch in range(num_epochs):
for batch_idx, (data, target) in enumerate(train_loader):
# 前向传播
optimizer.zero_grad()
output = model(data)
loss = loss_fn(output, target)
# 反向传播和累积梯度
loss.backward()
if (batch_idx + 1) % num累积 == 0:
optimizer.step()
optimizer.zero_grad()
```
这段代码中,通过在一
0
0
相关推荐
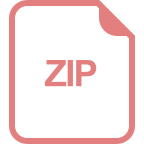
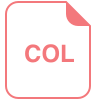
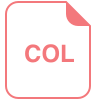
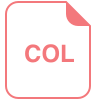
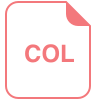
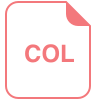
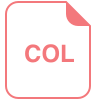