Goal Programming: Solving Multiobjective Linear Programming, Balancing Interests
发布时间: 2024-09-13 13:57:25 阅读量: 17 订阅数: 19 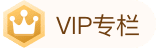
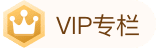
# Fundamental Concepts and Practical Applications of Multi-objective Linear Programming
## 1. Overview of Multi-objective Linear Programming
Multi-objective linear programming (MOLP) is a mathematical optimization technique used to solve decision-making problems with multiple competing objectives. Unlike traditional single-objective linear programming, MOLP aims to optimize several objective functions simultaneously to find a balanced solution that satisfies the needs of all objectives.
The applications of MOLP are widespread, including resource allocation, portfolio management, and environmental protection. By employing MOLP techniques, decision-makers can systematically evaluate trade-offs between different objectives and find an optimal solution to achieve the best combination of all objectives.
## 2. Theoretical Foundations of Multi-objective Linear Programming
### 2.1 Definition of Multi-objective Optimization Problems
A multi-objective optimization problem (MOP) is an optimization problem where multiple objective functions need to be optimized simultaneously. Unlike single-objective optimization problems, there is no single best solution in MOP; instead, there is a set of solutions known as the Pareto optimal set.
A Pareto optimal solution is one where it is impossible to improve any objective function value without decreasing another. In other words, Pareto optimal solutions represent a trade-off among all objectives.
### 2.2 Characteristics of Multi-objective Optimization Problems
MOPs have the following characteristics:
- **Multi-objectivity:** There are multiple objective functions that need to be optimized simultaneously.
- **Conflict:** Different objective functions often conflict, meaning improving one objective may lead to a decrease in another.
- **Pareto optimality:** There exists a set of Pareto optimal solutions that achieve a trade-off among all objectives.
- **Decision-maker involvement:** The solution to MOPs often requires the involvement of decision-makers to determine the relative importance of objective functions.
### 2.3 Solution Methods for Multi-objective Optimization Problems
Solution methods for MOPs can be divided into the following categories:
- **Weighted sum method:** Combines multiple objective functions by weighting them and forming a single objective function.
- **Constraint method:** Treats some objective functions as constraints and optimizes the remaining objective functions.
- **Genetic algorithm:** An algorithm inspired by evolutionary theory that searches for Pareto optimal solutions through selection, crossover, and mutation operations.
**Code Block:**
```python
import numpy as np
# Define objective functions
f1 = lambda x: x[0] ** 2 + x[1] ** 2
f2 = lambda x: (x[0] - 2) ** 2 + (x[1] - 2) ** 2
# Define weights
w1 = 0.5
w2 = 0.5
# Weighted sum method
def weighted_sum(x):
return w1 * f1(x) + w2 * f2(x)
# Constraint method
def constraint_method(x):
if f1(x) <= 1:
return f2(x)
else:
return np.inf
# Genetic algorithm
def genetic_algorithm(pop_size, num_generations):
# Initialize population
population = np.random.uniform(-5, 5, (pop_size, 2))
# Evolutionary loop
for i in range(num_generations):
# Selection
parents = selection(population)
# Crossover
children = crossover(parents)
# Mutation
children = mutation(children)
# Evaluation
fitness = [weighted_sum(x) for x in children]
# Select next generation
population = selection(children, fitness)
# Return the best solution
return population[np.argmin(fitness)]
```
**Logical Analysis:**
- The `weighted_sum` function implements the weighted sum method, which combines the two objective functions.
- The `constraint_method` function implements the constraint method, using `f1` as a constraint to optimize `f2`.
- The `genetic_algorithm` function implements a genetic algorithm, searching for Pareto optimal solutions through selection, crossover, and mutation operations.
**Parameter Explanation:**
- `pop_size`: Population size
- `num_generations`: Number of generations for evolution
- `selection`: Selection function
- `crossover`: Crossover function
- `mutation`: Mutation function
# 3. Practical Applications of Multi-objective Linear Programming
Multi-objective linear programming has a wide range of practical applications, and this article will introduce its typical use cases in resource allocation, portfolio management, and environmental protection.
### 3.1 Application in Resource Allocation
Resource allocation problems are one of the classic use cases for multi-objective linear pro
0
0
相关推荐
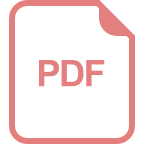
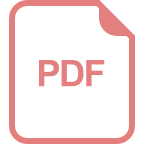
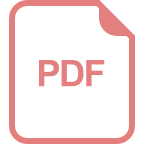
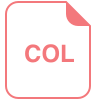
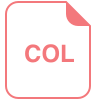
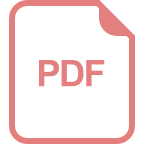
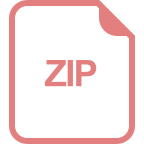
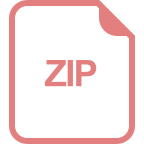
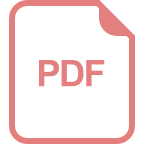