【Linear Programming Beginner's Guide】: Unveiling the Mysteries of Linear Programming, from Concept to Practice
发布时间: 2024-09-13 13:48:00 阅读量: 30 订阅数: 28 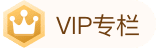
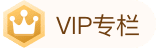
# Linear Programming Introduction Guide: Unveiling the Mysteries of Linear Programming from Concepts to Practical Application
## 1. Overview of Linear Programming
Linear programming is a mathematical optimization technique used to solve decision-making problems with linear objective functions and linear constraints. It is widely applied in fields such as economics, engineering, and management science.
A linear programming model consists of an objective function and constraint conditions. The objective function represents the goal to be maximized or minimized, such as profit or cost. Constraint conditions represent the limitations of the problem, such as resource availability or production capacity.
There are mainly two methods to solve linear programming problems: the graphical method and the simplex method. The graphical method is suitable for small-scale problems, while the simplex method is suitable for large-scale problems.
## 2. Theoretical Foundations of Linear Programming
### 2.1 Establishing a Linear Programming Model
#### 2.1.1 Standard Form Linear Programming Model
The standard form linear programming model is a mathematical model used to represent linear programming problems. It consists of the following parts:
- **Objective Function:** A linear function to be maximized or minimized.
- **Constraint Conditions:** A series of linear inequalities or equations that limit the range of decision variables.
- **Decision Variables:** Variables to be determined to optimize the objective function.
The general form of a standard form linear programming model is:
```
Maximize/Minimize Z = c1x1 + c2x2 + ... + cnxn
Subject to:
a11x1 + a12x2 + ... + a1nxn ≤/≥/ = b1
a21x1 + a22x2 + ... + a2nxn ≤/≥/ = b2
am1x1 + am2x2 + ... + amnxn ≤/≥/ = bm
x1, x2, ..., xn ≥ 0
```
Where:
- Z is the objective function.
- c1, c2, ..., cn are the coefficients of the objective function.
- x1, x2, ..., xn are the decision variables.
- a11, a12, ..., amn are the coefficients of the constraint conditions.
- b1, b2, ..., bm are the right-hand constants of the constraints.
#### 2.1.2 Transformation of the Standard Form Linear Programming Model
In some cases, linear programming problems may not be in standard form. To solve these problems, they need to be transformed into standard form. Transformation methods include:
***Introducing Slack Variables:** For inequality constraints, introduce slack variables to transform them into equality constraints.
***Introducing Surrogate Variables:** For minimization problems, introduce surrogate variables to transform them into maximization problems.
### 2.2 Solutions to Linear Programming
#### 2.2.1 Graphical Method
The graphical method is a small, intuitive approach to solving linear programming problems. It is suitable for problems with fewer decision variables.
**Steps:**
1. Draw the boundaries of all constraint inequalities or equations.
2. Determine the feasible region, which is the area that satisfies all constraints.
3. Find the maximum or minimum value of the objective function within the feasible region.
#### 2.2.2 Simplex Method
The simplex method is an iterative algorithm used to solve linear programming problems. It is suitable for problems with more decision variables.
**Steps:**
1. Convert the linear programming problem into standard form.
2. Construct the initial simplex tableau.
3. Iteratively perform the following steps until the optimal solution is found:
* Find the pivot element.
* Perform row operations on the pivot row.
* Perform column operations on the pivot column.
### 2.3 Properties and Applications of Linear Programming
#### 2.3.1 Properties of Linear Programming
Linear programming models have the following properties:
***Convexity:** The feasible region is a convex set.
***Optimality:** The optimal solution always exists at the extreme points of the feasible region.
***Sensitivity:** The optimal solution is sensitive to changes in model parameters.
#### 2.3.2 Applications of Linear Programming
Linear programming is applied in many fields, including:
* Production Planning
* Transportation Problems
* Allocation Problems
* Integer Programming
* Nonlinear Programming
* Stochastic Programming
## 3.1 Production Planning
#### 3.1.1 Mathematical Model of Production Planning
Production planning can be represented as a linear programming model:
```
Maximize: Total Profit = ∑(i=1,n) p_i * x_i
```
Where:
* p_i: Unit price of product i
* x_i: Production quantity of product i
* n: Number of product types
Constraint Conditions:
```
∑(i=1,n) a_ij * x_i ≤ b_j (j=1,m)
```
Where:
* a_ij: Consumption of product i on resource j
* b_j: Availability of resource j
* m: Number of resource types
#### 3.1.2 Solution to Production Planning Problem
The solution to the production planning problem can use the simplex method or the graphical method.
**Simplex Method**
The simplex method is an iterative algorithm that gradually approaches the optimal solution by continuously adjusting basic and non-basic variables. The specific steps are as follows:
1. Convert the model into a standard form linear programming model.
2. Choose an initial feasible solution.
3. Find a non-basic variable to enter the basis.
4. Find a basic variable to leave the basis.
5. Update the values of the basis and non-basic variables.
6. Repeat steps 3-5 until the optimal solution is found.
**Graphical Method**
The graphical method is suitable for linear programming problems with fewer variables. The specific steps are as follows:
1. Draw the constraints in a coordinate system to form a feasible region.
2. Find the vertices of the feasible region.
3. Calculate the objective function value for each vertex.
4. Choose the vertex with the largest objective function value as the optimal solution.
#### Code Example
```python
import pulp
# Define Variables
x1 = pulp.LpVariable("x1", lowBound=0)
x2 = pulp.LpVariable("x2", lowBound=0)
# Define Objective Function
objective = pulp.LpMaximize(5 * x1 + 4 * x2)
# Define Constraints
constraints = [
3 * x1 + 2 * x2 <= 12,
2 * x1 + 3 * x2 <= 15
]
# Create Linear Programming Model
model = pulp.LpProblem("Production Planning", pulp.LpMaximize)
model.setObjective(objective)
model.addConstraints(constraints)
# Solve Model
model.solve()
# Output Optimal Solution
print("Optimal Solution:")
print("x1 =", pulp.value(x1))
print("x2 =", pulp.value(x2))
print("Total Profit =", pulp.value(objective))
```
**Logical Analysis:**
* The `pulp.LpVariable` function defines two non-negative variables `x1` and `x2`, representing the production quantities of Product 1 and Product 2, respectively.
* The `pulp.LpMaximize` function defines the objective function, maximizing total profit.
* The `pulp.LpProblem` function creates a linear programming model, setting the objective function and constraints.
* The `model.solve()` function solves the model to find the optimal solution.
* The `pulp.value()` function retrieves the value of variables or the objective function.
#### Table Example
| Product | Price | Resource 1 Consumption | Resource 2 Consumption |
|---|---|---|---|
| Product 1 | 5 | 3 | 2 |
| Product 2 | 4 | 2 | 3 |
**Flowchart Example**
```mermaid
graph LR
subgraph Production Planning
A[Production Planning Problem] --> B[Establish Mathematical Model]
B --> C[Solve Model]
C --> D[Obtain Optimal Solution]
end
```
## 4. Advanced Applications of Linear Programming
### 4.1 Integer Programming
#### 4.1.1 Mathematical Model of Integer Programming
Integer programming is a special form of linear programming where the decision variables must be integers. The mathematical model of integer programming is as follows:
```
Maximize/Minimize z = c^T x
Subject to:
Ax ≤ b
x ≥ 0
x is an integer
```
Where:
* x is the decision variable vector
* c is the objective function coefficient vector
* A is the constraint matrix
* b is the constraint vector
#### 4.1.2 Solu***
***mon solution methods include:
***Branch and Bound Method:** Decompose the problem into a series of subproblems and solve them layer by layer until the optimal solution is found.
***Cutting Plane Method:** Add additional constraints to narrow the feasible region, thereby improving the quality of the solution.
***Heuristic Algorithms:** Use heuristic rules to quickly find an approximate optimal solution.
### 4.2 Nonlinear Programming
#### 4.2.1 Mathematical Model of Nonlinear Programming
Nonlinear programming is a generalization of linear programming, where the objective function or constraints are nonlinear. The mathematical model of nonlinear programming is as follows:
```
Maximize/Minimize f(x)
Subject to:
g(x) ≤ 0
h(x) = 0
```
Where:
* x is the decision variable vector
* f(x) is the objective function
* g(x) is the inequality constraint function
* h(x) is the equality constraint function
#### 4.2.2 So***
***mon solution methods include:
***Interior Point Method:** Convert the problem into a series of linear programming problems, gradually approaching the optimal solution.
***Gradient Method:** Use the gradient information of the objective function to search for the optimal solution in the gradient direction.
***Genetic Algorithm:** Simulate the biological evolution process, find an approximate optimal solution through selection, crossover, and mutation operations.
### 4.3 Stochastic Programming
#### 4.3.1 Mathematical Model of Stochastic Programming
Stochastic programming is an extension of linear programming, where some parameters are random variables. The mathematical model of stochastic programming is as follows:
```
Maximize/Minimize E[f(x, ξ)]
Subject to:
E[g(x, ξ)] ≤ 0
h(x) = 0
```
Where:
* x is the decision variable vector
* ξ is the random variable vector
* f(x, ξ) is the objective function
* g(x, ξ) is the inequality constraint function
* h(x) is the equality constraint function
#### 4.3.2 Solu***
***mon solution methods include:
***Monte Carlo Simulation:** Estimate the expected value of the objective function and the probability of satisfying constraints through random sampling.
***Scenario Optimization:** Discretize the random variables into a finite number of scenarios and solve a deterministic linear programming problem for each scenario.
***Robust Optimization:** Find the optimal solution that satisfies the constraints under all possible scenarios.
## 5.1 Extensions of Linear Programming
### 5.1.1 Multi-objective Linear Programming
Multi-objective linear programming (MOLP) is an extension of linear programming that considers optimization problems with multiple objectives. In MOLP, the objective function is no longer single but consists of multiple objective functions, which may conflict or be inconsistent with each other.
The mathematical model of MOLP is as follows:
```
min/max (f_1(x), f_2(x), ..., f_k(x))
s.t. Ax ≤ b, x ≥ 0
```
Where:
* `f_1(x), f_2(x), ..., f_k(x)` are the objective functions
* `A` is the constraint matrix
* `b` is the constraint vector
* `x` is the decision variable
The solution methods for MOLP mainly include:
* Weighted Sum Method: Weight and sum multiple objective functions into a single objective function.
* Constraint Method: Convert one or more objective functions into constraint conditions.
* Compromise Method: Transform the conflicts between objective functions into a single objective function.
### 5.1.2 Fuzzy Linear Programming
Fuzzy linear programming (FLP) is another extension of linear programming that considers factors of uncertainty and fuzziness. In FLP, the parameters or variables in the model may be uncertain or fuzzy.
The mathematical model of FLP is as follows:
```
min/max (f_1(x), f_2(x), ..., f_k(x))
s.t. Ax ≤ b, x ≥ 0
```
Where:
* `f_1(x), f_2(x), ..., f_k(x)` are the objective functions
* `A` is the constraint matrix
* `b` is the constraint vector
* `x` is the decision variable
The solution methods for FLP mainly include:
* Fuzzy Set Theory: Use fuzzy set theory to represent uncertainty and fuzziness.
* Stochastic Fuzzy Programming: Convert uncertainty into probability distributions and solve using stochastic programming methods.
* Robust Optimization: Solve the problem by considering the worst-case scenario of uncertainty.
0
0
相关推荐








