Multistage Linear Programming: A Linear Programming Model for Sequential Decision Making, Solving Complex Problems
发布时间: 2024-09-13 14:05:39 阅读量: 17 订阅数: 20 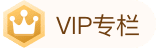
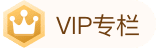
# 1. Introduction to Multistage Linear Programming
Multistage Linear Programming (MPLP) is an optimization problem where decisions are made over multiple stages, each based on the decisions made in the preceding stage. The goal of MPLP is to find a set of decisions that maximizes or minimizes a linear objective function over all stages.
MPLP is widely used in various practical problems such as portfolio optimization, production planning, and logistics distribution. In these problems, decision-makers need to make decisions over multiple time periods, and each decision affects the available options and the objective function value in subsequent stages.
# 2. Mathematical Model of Multistage Linear Programming
### 2.1 Basics of Linear Programming Model
**Linear Programming Model** is a mathematical model used to solve optimization problems characterized by the following:
***Linear Objective Function:** The objective function must be a linear combination of variables.
***Linear Constraints:** Constraint conditions must be linear equations or inequalities, which are linear combinations of variables equal to or less than/greater than a constant.
***Non-negative Variables:** All decision variables must be non-negative.
**Standard Form of Linear Programming Model:**
```
Maximize/Minimize z = c^T x
Subject to:
Ax ≤ b
x ≥ 0
```
Where:
* z is the objective function value
* x is the decision variable vector
* c is the objective function coefficient vector
* A is the constraint matrix
* b is the constraint vector
### 2.2 Expansion of Multistage Linear Programming Model
**Multistage Linear Programming Model** is an extension of the linear programming model, characterized by the following features:
***Multistage:** The problem is divided into multiple stages, each with its own objective function and constraints.
***Inter-stage Connections:** There is a connection between the decision variables of each stage, meaning that the decision variables of subsequent stages may depend on the decisions of preceding stages.
***Feasibility:** Each stage's decisions must satisfy that stage's constraints, and all stages' decisions must collectively satisfy the constraints of the entire problem.
**Mathematical Form of Multistage Linear Programming Model:**
```
Maximize/Minimize z = ∑_{t=1}^T z_t
Subject to:
Ax_t ≤ b_t, ∀t = 1, 2, ..., T
x_t ≥ 0, ∀t = 1, 2, ..., T
x_{t+1} = D_t x_t, ∀t = 1, 2, ..., T-1
```
Where:
* z_t is the objective function value of stage t
* x_t is the decision variable vector of stage t
* A_t is the constraint matrix of stage t
* b_t is the constraint vector of stage t
* D_t is the decision variable transition matrix from stage t to stage t+1
**Code Example:**
```python
import numpy as np
from scipy.optimize import linprog
# Define the number of stages
T = 3
# Define the objective function coefficient vector
c = np.array([1, 2, 3])
# Define the constraint matrix
A = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Define the constraint vector
b = np.array([10, 15, 20])
# Define the decision variable transition matrix
D = np.array([[0.5, 0.2, 0.3], [0.4, 0.6, 0.5], [0.1, 0.3, 0.6]])
# Solve the multistage linear programming problem
for t in range(T):
# Define the constraint matrix and constraint vector for stage t
A_t = A[t * 3:(t + 1) * 3, :]
b_t = b[t * 3:(t + 1) * 3]
# Define the decision variable transition matrix for stage t
D_t = D[t * 3:(t + 1) * 3, :]
# Solve the linear programming problem for stage t
res = linprog(c[t * 3:(t + 1) * 3], A_eq=A_t, b_eq=b_t, bounds=(None, None))
# Update the decision variables
x_t = res.x
# Print the decision variables for stage t
print("Decision Variables x{}: {}".format(t + 1, x_t))
```
**Code Logic Analysis:**
* Loops through each stage t.
* For each stage t, defines the constraint matrix A_t, constraint vector b_t, and decision variable transition matrix D_t for that stage.
* Uses scipy.optimize.linprog to solve the linear programming problem for the current stage.
* Updates the decision variables x_t.
* Prints the decision variables for stage t.
# 3.1 Stage-by-Stage Solving Algorithm
The stage-by-stage solving algorithm is a classic method for solving multistage linear programming problems. Its basic idea is to decompose the multistage problem into a series of single-stage linear programming problems, solving them stage by stage.
**Algorithm Steps:**
1. **Initialization:** Set the current stage to 1, and the initial feasible solution to the feasible solution of stage 1.
2. **Solve Single-Stage Linear Programming:** Solve the single-stage linear programming problem of the current stage to obtain the optimal solution.
3. **Update State:** Use the optimal solution of the current stage as the initial feasible solution for the next stage.
4. **Determine Termination:** If the current stage is the last stage, the algorithm terminates; otherwise, return to step 2.
**Code Example:**
```python
import numpy as np
from scipy.optimize import linprog
def multi_stage_linear_programming(c, A, b, n):
"""
Solve multistage linear programming problems in a stage-by-stage manner.
Parameters:
c: Objective function coefficient vector
A: Constraint matrix
b: Constraint vector
n: Number of stages
Returns:
Optimal solution
"""
# Initialization
x = np.zeros(c.shape)
for i in range(n):
# Solve single-stage linear programming
res = linprog(c[i], A_eq=A[i], b_eq=b[i])
x[i * c.
```
0
0
相关推荐
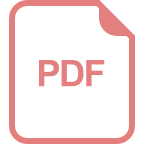
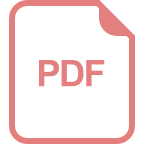
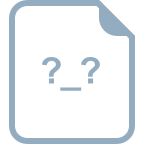
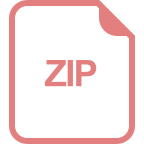
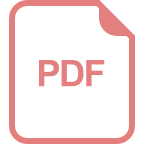
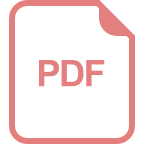
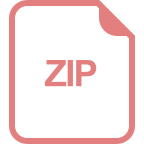
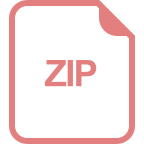
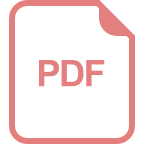