Comprehensive Application of Linear Programming in Supply Chain Management: Optimizing Processes and Enhancing Efficiency
发布时间: 2024-09-13 14:10:54 阅读量: 29 订阅数: 30 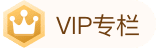
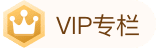
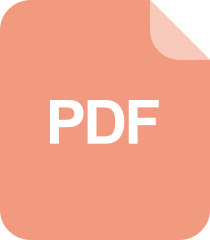
PGWinFunc: Optimizing Window Aggregate Functions in PostgreSQL and its application for trajectory data
# Comprehensive Application of Linear Programming in Supply Chain Management: Optimizing Processes and Enhancing Efficiency
## 1. Overview of Linear Programming**
Linear programming is a mathematical optimization technique used to solve optimization problems with linear objective functions and linear constraints. It is widely applied in supply chain management, production planning, finance, and logistics, among other fields.
A linear programming model consists of an objective function and constraints. The objective function represents the goal to be optimized, such as maximizing profit or minimizing cost. Constraints define the limitations of the problem, such as resource availability or production capacity. By solving the linear programming model, a decision can be found that satisfies all constraints and optimizes the objective function to its best value.
The primary methods for solving linear programming models are the simplex method and the interior-point method. The simplex method is an iterative algorithm that gradually optimizes feasible solutions to the optimal solution by continuously exchanging basic variables. The interior-point method is a direct approach that obtains the optimal solution by solving a system of linear equations.
## 2. Theoretical Foundation of Linear Programming in Supply Chain Management
### 2.1 Establishment of Linear Programming Models
**Components of a Linear Programming Model**
A linear programming model is composed of the following elements:
- **Decision variables:** Unknowns in the problem requiring optimization.
- **Objective function:** Represents the goal to be optimized, typically a linear function.
- **Constraints:** A set of equations or inequalities that limit the range of values for the decision variables.
**Steps for Model Construction**
1. **Identify decision variables:** Recognize the variables that need to be optimized.
2. **Establish an objective function:** Develop a linear function based on the optimization goal.
3. **Establish constraints:** Create constraints based on actual conditions that limit the range of values for the decision variables.
**Example**
Consider a stock management problem aimed at determining the optimal inventory level for each product to minimize total inventory costs.
- **Decision variables:** Inventory levels for each product.
- **Objective function:** Total inventory cost = ordering cost + holding cost.
- **Constraints:** Inventory levels cannot be negative and cannot exceed warehouse capacity.
### 2.2 Solving Linear Programming Models
**Simplex Method**
The simplex method is a classic approach to solving linear programming models. It iteratively searches for the best feasible solution that satisfies the constraints.
**Steps of the Simplex Method**
1. **Initialization:** Convert the model to standard form and establish an initial feasible solution.
2. **Select an entering variable:** Choose a variable that can improve the objective function.
3. **Select a leaving variable:** Choose a variable that maintains feasibility.
4. **Update basic variables:** Replace the leaving variable with the entering variable and update the basic variables.
5. **Repeat steps 2-4:** Until the optimal solution is found.
**Other Solving Methods**
In addition to the simplex method, other methods for solving linear programming models include the interior-point method and the dual simplex method, among others.
**Code Example**
```python
import pulp
# Create a linear programming model
model = pulp.LpProblem("Inventory Management", pulp.LpMinimize)
# Define decision variables
x1 = pulp.LpVariable("Product1 Inventory Level", lowBound=0)
x2 = pulp.LpVariable("Product2 Inventory Level", lowBound=0)
# Define the objective function
objective = x1 + 2 * x2
# Define constraints
constraints = [
x1 + x2 <= 100, # Total inventory capacity constraint
x1 >= 20, # Product1 minimum inventory level
x2 >= 10, # Product2 minimum inventory level
]
# Add the objective function and constraints to the model
model += objective
for constraint in constraints:
model += constraint
# Solve the model
model.solve()
# Print the optimal solution
print("Product1 Inventory Level:", pulp.value(x1))
print("Product2 Inventory Level:", pulp.value(x2))
```
**Code Logic Analysis**
- `pulp.LpProblem`: Create a linear programming model, specifying the name and optimization goal (minimization).
- `pulp.LpVariable`: Define decision variables and specify the lower bound (`lowBound`).
- `objective`: Define the objective function, which is a linear function.
- `constraints`: Define constraints, which form a system of equations or inequalities.
- `model += objective`: Add the objective function to the model.
- `for constraint in constraints: model += constraint`: Add constraints to the model.
- `model.solve()`: Solve the model.
- `pulp.value(x1)` and `pulp.value(x2)`: Output the
0
0
相关推荐







