Comprehensive Guide to Date Handling Functions in MATLAB
发布时间: 2024-09-15 16:12:33 阅读量: 24 订阅数: 27 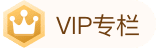
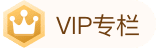
# Chapter 1: Fundamental Concepts of Date Data in MATLAB
Handling date data in MATLAB is a common task, and this chapter will introduce the basic concepts of date data in MATLAB, including how date data is represented, commonly used date data formats, and how to create and represent date data in MATLAB.
## 1.1 Representation of Date Data in MATLAB
In MATLAB, date data is typically represented as a date vector containing components such as year, month, day, hour, minute, and second. This representation facilitates date calculations and analysis.
## 1.2 Commonly Used Date Data Formats in MATLAB
MATLAB supports a variety of date data formats, including date strings, serial date numbers, and date vectors. Different formats are suitable for different operation scenarios.
## 1.3 Creating and Representing Date Data in MATLAB
In MATLAB, date data can be created and represented using built-in date functions or by directly specifying a date vector. Date data can be input directly or imported from other formats using conversion functions.
Through the study of this chapter, readers will gain a clear understanding of the basic concepts of date data in MATLAB, laying the foundation for subsequent date data processing.
# Chapter 2: Importing and Exporting Date Data
In this chapter, we will explore how to import and export date data in MATLAB. Importing and exporting date data is a common requirement when dealing with actual data, and effective import and export operations can improve data processing efficiency and accuracy.
### 2.1 Importing Date Data from External Files into MATLAB
In MATLAB, date data can be imported from external files in various ways, including using the `readtable` function to import Excel or CSV files and the `load` function to import MAT files. When importing date data, special attention should be paid to the format of the date data to ensure that MATLAB can correctly identify the date information.
```matlab
% Using the readtable function to import date data from an Excel file
data = readtable('data.xlsx');
% Displaying the imported date data
disp(data);
```
### 2.2 Exporting Date Data from MATLAB to External Files
Exporting date data from MATLAB to external files is equally important and can be done using the `writetable` function to save data as Excel or CSV files, or the `save` function to save data as MAT files.
```matlab
% Saving date data as an Excel file
writetable(data, 'output.xlsx');
% Saving date data as a MAT file
save('output.mat', 'data');
```
### 2.3 Handling the Import and Export of Date Data in Different Formats
In practice, you may encounter date data in different formats, such as "yyyy-MM-dd HH:mm:ss" or "dd/MM/yyyy". When importing and exporting, you need to adjust MATLAB's reading and saving settings according to the specific date format to ensure the accuracy and consistency of the date data.
```matlab
% Specifying the date format and importing
opts = detectImportOptions('data.csv');
opts = setvaropts(opts, 'DateColumn', 'InputFormat', 'yyyy-MM-dd HH:mm:ss');
data = readtable('data.csv', opts);
% Saving the date data in the specified format
writetable(data, 'output.csv', 'WriteVariableNames', true, 'DatetimeFormat', 'yyyy-MM-dd HH:mm:ss');
```
With these methods, we can effectively perform import and export operations for date data and adjust the format of the date data according to actual needs, ensuring the accuracy and flexibility of data processing.
# Chapter 3: Displaying and Formatting Date Data
In this chapter, we will focus on how to display and format date data in MATLAB, including built-in date formatting functions, custom date display formats, and handling and displaying time zones for date data.
#### 3.1 Built-in Date Formatting Functions in MATLAB
MATLAB provides some built-in functions for formatting date data, with commonly used functions including `datestr()` and `datenum()`. The `datestr()` function can convert date data into a date string of a specified format, while the `datenum()` function can convert date strings into MATLAB's serialized date format.
```matlab
% Example: Using the datestr function to format dates
date = datetime('now'); % Get the current date and time
formatted_date = datestr(date, 'yyyy-mm-dd HH:MM:SS'); % Format as 'yyyy-mm-dd HH:MM:SS'
disp(formatted_date);
```
#### 3.2 Customizing the Display Format of Date Data
In addition to built-in functions, we can also customize the display format of date data, for example, using the `strftime()` function. This allows you to flexibly define the display of dates according to your own needs.
```matlab
% Example: Custom date formatting display
date = datetime('now'); % Get the current date and time
custom_format = 'Today is %A, %B %d, %Y'; % Custom formatting string
formatted_date = datestr(date, custom_format);
disp(formatted_date);
```
#### 3.3 Handling and Displaying Time Zones for Date Data
When dealing with date data involving different time zones, special attention must be p
0
0
相关推荐






