Common Issues and Solutions with Date Data in MATLAB
发布时间: 2024-09-15 16:16:44 阅读量: 23 订阅数: 18 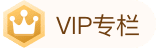
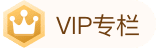
# 1. Understanding Date Data Types in MATLAB
When working with date data in MATLAB, it's crucial to understand the date data types. Here's a basic introduction to the date data types in MATLAB.
## 1.1 Date Data Types in MATLAB
In MATLAB, date data is usually represented as a serial number or a set of numbers that indicate the number of days or time elapsed since a specific starting date (e.g., "0000-01-00"). MATLAB provides built-in functions and tools for handling date data, making it easy to perform date calculations, comparisons, and display.
## 1.2 Representations of Date Data
Date data can be represented in various ways, including date vectors, serial numbers, or character formats. Date vectors typically include components such as year, month, day, hour, minute, and second; serial numbers count the continuous days from a specific starting date; character formats can be common date string representations, such as 'yyyy-mm-dd'.
## 1.3 Common Date Formats in MATLAB
In MATLAB, date data can be represented in different formats, including:
- 'dd-mmm-yyyy': For example, '01-Jan-2022'
- 'yyyy-mm-dd': For example, '2022-01-01'
- 'dd/mm/yyyy': For example, '01/01/2022'
Understanding how date data is represented and the common formats is essential for correctly handling date data. Next, we'll discuss methods for inputting and outputting date data.
# 2. Date Data Input and Output
When working with date data in MATLAB, it's necessary to understand how to perform input and output operations. We'll cover methods for inputting and outputting date data, as well as setting display formats for date data.
### 2.1 Methods for Inputting Date Data
In MATLAB, you can use various functions to input date data, including the `datetime()` function and the `datenum()` function. The `datetime()` function can input date data in different formats and convert them to MATLAB's internal date-time format. Examples are as follows:
```matlab
% Using the datetime() function to input date data
date1 = datetime('24-Dec-2022');
date2 = datetime('2022-12-24');
disp(date1);
disp(date2);
```
### 2.2 Methods for Outputting Date Data
To output date data in a specific format, you can use the `datestr()` function. This function can convert MATLAB date data into character date data in the specified format. Examples are as follows:
```matlab
% Using the datestr() function to output date data
date = datetime('2022-12-24');
dateStr = datestr(date, 'yyyy/mm/dd');
disp(dateStr);
```
### 2.3 Setting the Display Format for Date Data
In MATLAB, you can use the `format` function to set the display format for date data. Date display formats that can be set include `'default'` (default format), `'short'` (short format), `'long'` (long format), etc. Examples are as follows:
```matlab
% Set the display format for date data to short format
format short;
disp(datetime('2022-12-24'));
% Set the display format for date data to long format
format long;
disp(datetime('2022-12-24'));
```
With these methods, you can flexibly input, output, and set display formats for date data, making it convenient for processing and presenting date data.
# 3. Operations and Transformations of Date Data
In MATLAB, operations and transformations of date data are very common. We'll discuss date data arithmetic, conversions of date units, and comparisons of date data in detail.
#### 3.1 Date Data Arithmetic Operations
Date data arithmetic operations can achieve date offsets, with common
0
0
相关推荐
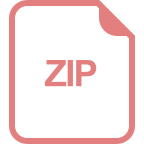
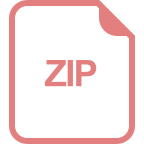





