How to Handle Missing Date Data in Excel Using MATLAB
发布时间: 2024-09-15 16:17:31 阅读量: 16 订阅数: 18 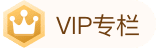
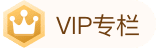
# 1. Introduction to Date Data in Excel
Processing date data in Excel is one of the common tasks in data analysis. Understanding the storage format and common representations of date data in Excel is crucial for subsequent processing. This chapter will introduce the storage and representation of date data in Excel, preparing for subsequent handling.
# 2. Importing Excel Data into MATLAB
Before handling date data in Excel, it is necessary to import the Excel data into MATLAB first. The following will introduce how to use MATLAB functions to import Excel data and resolve the potential date data importing issues encountered during the process.
### 2.1 Importing Excel Data Using MATLAB Functions
In MATLAB, the `xlsread` function can be used to import data from Excel files, including date data. The basic syntax of the function is as follows:
```matlab
[num,txt,raw] = xlsread('filename.xlsx');
```
- `filename.xlsx`: The name of the Excel file to be imported.
- `num`: A matrix containing numerical data.
- `txt`: A matrix containing text data.
- `raw`: A cell array containing raw data.
### 2.2 Handling Date Data Import Issues in Excel
When importing date data from Excel, you might encounter issues with mismatched date formats. To ensure correct import of date data, you can use the `readtable` function, which supports automatic inference of date, time, and text data formats and stores them in a table.
Here is an example code for importing Excel data using the `readtable` function:
```matlab
T = readtable('filename.xlsx');
```
With these methods, date data in Excel can be accurately imported into MATLAB, laying the foundation for subsequent date data processing.
# 3. Analyzing Missing Date Data in Excel
Missing date data is a common issue when dealing with data in Excel. Missing date data can impact subsequent analysis and processing, so it requires analysis and handling.
#### 3.1 How to Identify Missing Date Data in Excel
In MATLAB, after reading Excel data, functions such as `ismissing` can be used to identify missing data in Excel. For date data, missing dates might be empty values or specific placeholders. You can iterate over each date data to determine if it is missing and handle it accordingly.
```python
import pandas as pd
# Read Excel data
data = pd.read_excel('data.xlsx')
# Iterate through each data, determine if the date data is missing
for index, row in data.iterrows():
if pd.isnull(row['Date']):
print(f"Row {index+1}: Missing date value!"
```
0
0
相关推荐
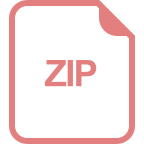
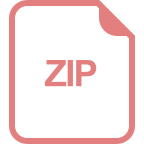
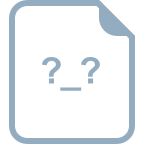
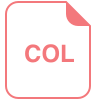
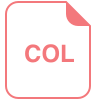
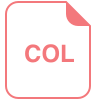
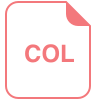
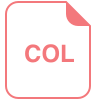
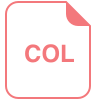