Understanding Basic Methods of Reading Excel in MATLAB
发布时间: 2024-09-15 16:03:58 阅读量: 27 订阅数: 28 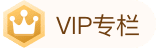
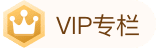
# 1. Introduction
## 1.1 What is MATLAB?
MATLAB (Matrix Laboratory) is a powerful scientific computing software extensively used in engineering, mathematics, and scientific fields. It offers a plethora of function libraries and tools suitable for data analysis, visualization, modeling, simulation, and various other tasks.
## 1.2 The Importance of Excel in Scientific Computing
Excel, as a spreadsheet processing software, also plays a significant role in scientific computing. Many experimental data, statistical information, etc., exist in the form of Excel files, making it common to import Excel data into MATLAB for further processing and analysis.
## 1.3 Overview of the Main Content of This Article
This article will focus on how to use MATLAB to read data from Excel files, including importing Excel data into the MATLAB workspace, methods for reading different types of Excel files, data processing and analysis, advanced application tips, etc. Through the study of this article, readers can master how to effectively use MATLAB to handle Excel data, enhancing work efficiency and the accuracy of data analysis.
# 2. Preliminary Work
Before starting to use MATLAB to read Excel, some preliminary work needs to be done to ensure the smooth progress of data reading and processing.
### 2.1 Installing MATLAB and Other Necessary Tools
First, ensure that you have correctly installed the MATLAB software. MATLAB is a powerful tool for mathematical computation, data analysis, and visualization, which is very practical when dealing with Excel data. You can download and install MATLAB from the MathWorks official website.
Additionally, to better handle Excel files, it is recommended to install the Microsoft Office suite, which includes Excel software, to ensure that files can be correctly read and processed.
### 2.2 Preparing Excel Data Files
Before starting to read Excel, you need to prepare the Excel data files you want to read. These could be experimental data, statistical data, report data, etc. Ensure that the file path is correct and that you have the permission to read it.
### 2.3 Ensuring Data File Format and Structure Meet Requirements
Before importing Excel data into MATLAB, it is necessary to ensure that the Excel data file's format and structure meet the requirements. For example, whether the data table has appropriate column names, whether the data is complete, and whether there are missing values. These factors will affect the results of subsequent data processing and analysis. If the data file requires cleaning or preprocessing, it can be done in advance to facilitate subsequent analytical work.
# 3. Using MATLAB to Read Excel
In scientific computation and data analysis, importing Excel data into MATLAB for processing is a very common operation. MATLAB provides abundant functions and tools that can easily read and process data from Excel files. Next, we will introduce how to use MATLAB to read Excel files.
#### 3.1 Importing Excel Data into the MATLAB Workspace
First, we need to use the `xlsread()` function in MATLAB to read the content of the Excel file and import it into the MATLAB workspace. Below is a simple example code:
```matlab
% Reading data from an Excel file
filename = 'example.xlsx'; % Setting the Excel file name
sheet = 1; % Setting the worksheet to read
range = 'A1:C10'; % Setting the cell range to read
[data, headers] = xlsread(filename, sheet, range);
% Displaying the reading results
disp('Data read:');
disp(data);
disp('Column headers of the worksheet:');
disp(headers);
```
In this example, we use the `xlsread()` function to read the data within the range of A1 to C10 on the first worksheet of the Excel file named "example.xlsx" and store the data in the `data` variable and the column headers of the worksheet in the `headers` variable.
#### 3.2 Using Different Functions to Read Different Types of Excel Files
In addition to the `xlsread()` function, MATLAB also provides other functions for reading Excel files of different formats, such as the `readtable()` function for reading Excel files containing mixed data types and the `readcell()` function for reading Excel files containing cell arrays, etc. Depending on the format and structure of the Excel file, choosing the appropriate function to read the data will be more convenient and efficient.
#### 3.3 Processing Excel Data for Subsequent Analysis
After importing Excel data into MATLAB, we can use various functions and tools provided by MATLAB to process the data, such as data cleaning, data transformation, data filtering, etc., to facilitate subsequent analysis and visualization. By combining MATLAB's powerful data processing and analysis capabilities, we can better understand and utilize the data information in Excel.
During the process of importing and processing Excel data, it is necessary to pay attention to the format and structure of the data to ensure the integrity and accuracy of the data, so that subsequent scientific computation and data analysis can proceed smoothly. The flexibility and powerful features of MATLAB can help us process Excel data more efficiently and enhance work efficiency and the accuracy of data analysis.
# 4. Data Processing and Analysis
After using MATLAB to read Excel data, the next critical step is to process and analyze the data. Below, we will详细介绍 how to perform data processing and analysis in MATLAB.
#### 4.1 Data Cleaning and Preprocessing
Data cleaning is the first step in data processing, which includes dealing with missing values, outliers, duplicate values, and other data quality issues. In MATLAB, various functions and tools can be used for data cleaning, such as the `isnan` and `unique` functions, which can help you handle missing values and duplicate values in the data.
```matlab
% Example: Handling missing values
data = [1, 2, NaN, 4, 5];
cleaned_data = data(~isnan(data)); % Remove missing values
% Example: Handling duplicate values
data = [1, 2, 3, 3, 4, 4, 5];
unique_data = unique(data); % Remove duplicate values
```
#### 4.2 Data Analysis and Visualization
Data analysis is the process of using statistical and mathematical methods to deeply analyze data, while data visualization is the presentation of analytical results in graphical form, which helps to understand the data more intuitively. MATLAB offers a variety of data analysis and visualization functions, such as `mean`, `std`, `histogram`, etc.
```matlab
% Example: Calculating mean and standard deviation
data = [1, 2, 3, 4, 5];
mean_value = mean(data); % Calculate the mean
std_value = std(data); % Calculate the standard deviation
% Example: Drawing a histogram
data = randn(1000,1); % Generate 1000 normally distributed random numbers
histogram(data, 'Normalization', 'pdf'); % Draw a histogram
```
#### 4.3 Data Export and Report Generation
After data processing and analysis, you may need to export the results to an Excel file or generate reports. MATLAB provides functions like `writematrix` and `writetable` to conveniently export data to Excel files, and you can also use the MATLAB Report Generator tool to generate professional reports.
```matlab
% Example: Export data to an Excel file
data = [1, 2, 3, 4, 5];
writematrix(data, 'output.xlsx'); % Write data into an Excel file
% Example: Generate a report
report = mlreportgen.report.Report('Data Analysis Report', 'pdf');
chapter = mlreportgen.report.Chapter('Title', 'Data Summary');
section = mlreportgen.report.Section;
para = mlreportgen.report.Paragraph('Data analysis results...');
append(chapter, section);
append(section, para);
append(report, chapter);
close(report);
```
With these examples, you can learn how to clean, analyze, and generate reports from Excel data in MATLAB. Hopefully, this information will be helpful to you.
# 5. Advanced Applications
In this chapter, we will introduce some advanced scenarios and techniques for MATLAB to read Excel, which will help users process Excel data more flexibly and efficiently.
#### 5.1 Using MATLAB for Data Interaction and Update
In real applications, Excel data often needs to be continuously updated and processed. MATLAB provides various methods for data interaction that allow direct modification and update of Excel data within the MATLAB environment. For instance, you can read Excel data using the `xlsread` function, process the data with MATLAB's numerical computation features, and then write the results back to an Excel file using the `xlswrite` function.
```matlab
% Reading Excel data
data = xlsread('data.xlsx');
% Data processing
processed_data = some_processing_function(data);
% Writing the processed data back to an Excel file
xlswrite('processed_data.xlsx', processed_data);
```
#### 5.2 Writing MATLAB-Processed Data into a New Excel File
In addition to data interaction, you can also directly write MATLAB-processed data into a new Excel file. This is very convenient for generating reports and outputting results. The `xlswrite` function can be used to write matrices or data from MATLAB into an Excel file.
```matlab
% Generating some data
data = magic(5);
% Writing data into an Excel file
xlswrite('output_data.xlsx', data);
```
#### 5.3 Customizing Functions and Scripts for More Flexible Data Processing
To better handle Excel data, you can write custom MATLAB functions and scripts. These custom tools can implement a variety of complex data processing functions based on specific requirements, increasing work efficiency. For example, you can write functions to process specific formats of Excel files or implement specific data cleaning algorithms.
```matlab
% Custom function example
function cleaned_data = data_cleaning(data)
% Write the data cleaning logic here
cleaned_data = some_cleaning_algorithm(data);
end
% Using a custom function to process data
data = xlsread('raw_data.xlsx');
cleaned_data = data_cleaning(data);
xlswrite('cleaned_data.xlsx', cleaned_data);
```
Through the above advanced application techniques, users can more flexibly utilize MATLAB in interaction with Excel for data processing and interaction, meeting requirements in various scenarios and improving work efficiency and the quality of data processing.
# 6. Conclusion and Outlook
In this article, we have delved into the fundamental methods of using MATLAB to read Excel files. Through introducing the importance of MATLAB and Excel in scientific computation and explaining the steps of data preparation, reading, processing, and analysis in detail, we hope readers can better master this important skill.
#### 6.1 Summary of This Article
In this article, we first introduced the basic concepts of MATLAB and Excel, and then explained in detail how to prepare your work environment and Excel files in MATLAB for smooth data reading. Subsequently, we demonstrated how to use different functions to read Excel files and process and analyze the data that has been read. Finally, we explored some advanced applications, such as data interaction, writing data into Excel, and the use of custom functions and scripts.
#### 6.2 The Value and Significance of Learning MATLAB to Read Excel
Mastering the method of MATLAB to read Excel is very important for scientific computation and data analysis. Excel, as a widely used data processing tool, combined with MATLAB's powerful computational capabilities, can help users process and analyze data more efficiently, enhancing work efficiency and accuracy.
#### 6.3 Future Development Trends and Suggestions
With the continuous development of the data science field, the demand for data processing and analysis is also increasing. In the future, we suggest further in-depth research on the combined application of MATLAB and Excel, exploring more data processing technologies and methods to meet the evolving challenges of data processing.
Through the study of this article, we believe that readers can better understand the basic methods of MATLAB to read Excel and flexibly apply them in practical work, providing more possibilities for scientific computation and data analysis.
0
0
相关推荐








