atan函数在图像处理中的妙用:图像旋转与透视变换,让你的图像处理技能更上一层楼
发布时间: 2024-07-09 02:00:15 阅读量: 40 订阅数: 50 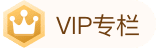
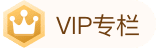
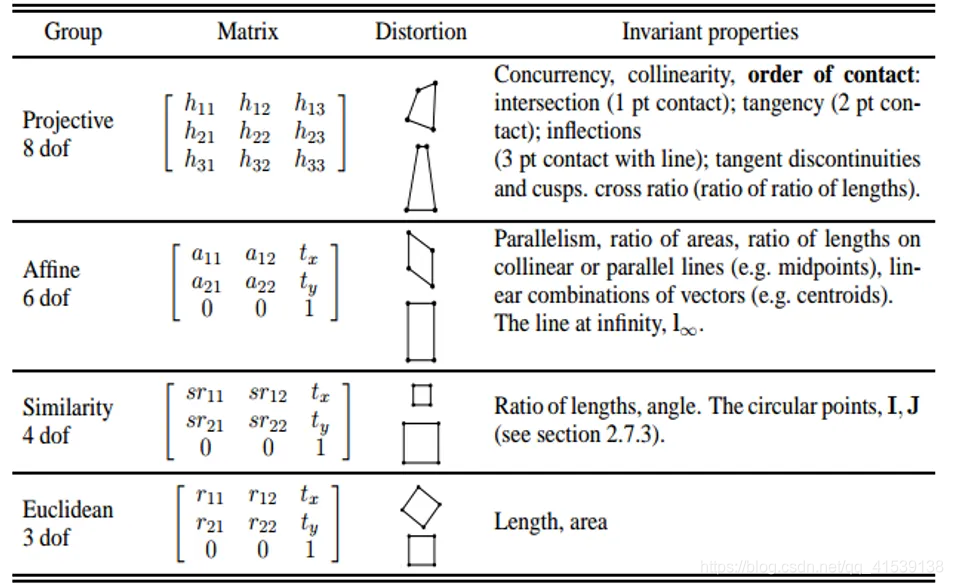
# 1. 图像处理中的atan函数简介
**1.1 atan函数简介**
atan函数是反切函数,用于计算给定正切值的弧度值。在图像处理中,atan函数主要用于计算图像旋转和透视变换所需的旋转角度和透视参数。
**1.2 atan函数在图像处理中的应用**
atan函数在图像处理中有着广泛的应用,包括:
- 图像旋转:计算图像旋转所需的旋转角度。
- 图像透视变换:计算图像透视变换所需的透视参数。
- 图像倾斜校正:计算图像倾斜角并进行校正。
- 图像裁剪:确定图像裁剪区域并进行裁剪。
# 2. 图像旋转
### 2.1 图像旋转原理
#### 2.1.1 旋转矩阵与atan函数
图像旋转可以通过应用旋转矩阵来实现。旋转矩阵是一个 2x2 矩阵,它描述了图像中每个像素的旋转。
```python
import numpy as np
# 定义旋转角度
theta = np.pi / 3 # 60 度
# 计算旋转矩阵
rotation_matrix = np.array([[np.cos(theta), -np.sin(theta)],
[np.sin(theta), np.cos(theta)]])
```
atan 函数可以用来计算旋转矩阵中的角度。atan 函数的输入是正切值,输出是角度(以弧度为单位)。在图像旋转中,正切值是旋转矩阵中 y 坐标与 x 坐标的比值。
```python
# 计算旋转角度
angle = np.arctan(rotation_matrix[1, 0] / rotation_matrix[0, 0])
```
#### 2.1.2 旋转角度的计算
旋转角度可以根据图像的旋转需求进行计算。例如,要将图像旋转 60 度,可以使用以下公式:
```python
angle = 60 * np.pi / 180 # 将角度转换为弧度
```
### 2.2 图像旋转实践
#### 2.2.1 OpenCV中的图像旋转函数
OpenCV 提供了 `cv2.warpAffine` 函数来执行图像旋转。该函数需要旋转矩阵和图像作为输入,并返回旋转后的图像。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 应用旋转
rotated_image = cv2.warpAffine(image, rotation_matrix, (image.shape[1], image.shape[0]))
```
#### 2.2.2 图像旋转代码示例
以下代码示例演示了如何使用 OpenCV 旋转图像:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 定义旋转角度
theta = np.pi / 3 # 60 度
# 计算旋转矩阵
rotation_matrix = np.array([[np.cos(theta), -np.sin(theta)],
[np.sin(theta), np.cos(theta)]])
# 应用旋转
rotated_image = cv2.warpAffine(image, rotation_matrix, (image.shape[1], image.shape[0]))
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 3.1 透视变换原理
透视变换是一种几何变换,它可以将图像中的一个平面投影到另一个平面上。在图像处理中,透视变换通常用于纠正图像的透视失真,例如当相机与拍摄对象不平行时产生的失真。
#### 3.1.1 透视投影矩阵与atan函数
透视投影矩阵是一个 3x3 矩阵,它定义了图像中的一个平面如何投影到另一个平面上。透视投影矩阵包含 9 个参数,其中 8 个参数定义了投影变换,而第 9 个参数为 1。
```python
import numpy as np
# 透视投影矩阵
P = np.array([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0]])
```
atan 函数在透视投影矩阵中用于计算投影变换的参数。atan 函数计算一个点的切线值,它可以用来计算投影变换中旋转和缩放的参数。
#### 3.1.2 透视变换参数的计算
透视变换参数可以通过求解透视投影矩阵的逆矩阵来计算。逆矩阵可以通过使用 NumPy 的 `linalg.inv()` 函数来计算。
```python
# 求解透视投影矩阵的逆矩阵
P_inv = np.linalg.inv(P)
# 透视变换参数
params = P_inv.flatten()
```
透视变换参数包含 8 个元素,它们定义了投影变换的旋转、缩放和位移。
```
params = [
rotation_x, rotation_y, rotation_z,
scale_x, scale_y, scale_z,
translation_x, translation_y
]
```
# 4. atan函数在图像处理中的其他应用
除了图像旋转和透视变换之外,atan函数在图像处理中还有其他广泛的应用,包括图像倾斜校正和图像裁剪。
### 4.1 图像倾斜校正
图像倾斜校正是一种将倾斜的图像恢复到正常位置的技术。它在扫描文档、图像稳定和透视校正中至关重要。
#### 4.1.1 倾斜角的计算
图像倾斜角的计算是图像倾斜校正的关键步骤。可以使用atan函数来计算图像中直线的倾斜角。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('tilted_image.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测器检测边缘
edges = cv2.Canny(gray, 100, 200)
# 使用霍夫变换检测直线
lines = cv2.HoughLinesP(edges, 1, np.pi / 180, 100, minLineLength=100, maxLineGap=10)
# 计算倾斜角
if lines is not None:
for line in lines:
x1, y1, x2, y2 = line[0]
angle = np.arctan((y2 - y1) / (x2 - x1)) * 180 / np.pi
print("倾斜角:", angle)
```
#### 4.1.2 图像倾斜校正代码示例
一旦计算出倾斜角,就可以使用旋转变换来校正图像。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('tilted_image.jpg')
# 计算倾斜角
angle = ... # 使用上述方法计算的倾斜角
# 创建旋转矩阵
M = cv2.getRotationMatrix2D((image.shape[1] / 2, image.shape[0] / 2), -angle, 1)
# 旋转图像
corrected_image = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]))
# 显示校正后的图像
cv2.imshow('校正后的图像', corrected_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 4.2 图像裁剪
图像裁剪是一种从图像中提取特定区域的技术。它在对象识别、人脸检测和图像编辑中非常有用。
#### 4.2.1 裁剪区域的确定
裁剪区域的确定是图像裁剪的关键步骤。可以使用atan函数来计算图像中对象的边界框。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image_with_object.jpg')
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用二值化阈值分割图像
thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)[1]
# 查找图像中的轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 计算边界框
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
print("边界框:", (x, y, w, h))
```
#### 4.2.2 图像裁剪代码示例
一旦确定了裁剪区域,就可以使用切片操作来裁剪图像。
```python
import cv2
# 读取图像
image = cv2.imread('image_with_object.jpg')
# 计算边界框
x, y, w, h = ... # 使用上述方法计算的边界框
# 裁剪图像
cropped_image = image[y:y+h, x:x+w]
# 显示裁剪后的图像
cv2.imshow('裁剪后的图像', cropped_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
# 5. 图像处理中的atan函数总结
### 5.1 atan函数在图像处理中的优势
- **数学基础牢固:**atan函数基于三角函数,在图像处理中具有坚实的数学基础,可用于解决各种几何变换问题。
- **计算高效:**atan函数的计算速度较快,尤其是在处理大图像时,可以有效提高图像处理效率。
- **广泛应用:**atan函数广泛应用于图像旋转、透视变换、倾斜校正和裁剪等图像处理任务中。
### 5.2 图像处理中atan函数的注意事项
- **角度范围:**atan函数的输出范围为[-π/2, π/2],在使用时需要考虑角度范围的限制。
- **奇点:**atan函数在输入为π/2或-π/2时会出现奇点,需要特殊处理。
- **精度:**atan函数的精度受浮点运算的影响,在某些情况下可能导致精度损失。
0
0
相关推荐
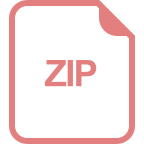
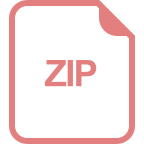






