Python实践:手把手教你实现高效的反向传播算法
发布时间: 2024-09-05 15:05:57 阅读量: 32 订阅数: 37 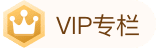
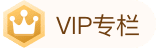

# 1. 反向传播算法基础概念
在深度学习领域,反向传播算法是实现模型训练的核心技术之一。本章我们将介绍反向传播的基本概念,为理解其在深度学习中的关键作用打下坚实的基础。
## 1.1 理解神经网络中的信号传递
反向传播算法是一种通过计算网络中每个参数的梯度来调整权重的方法,它是基于梯度下降算法的。在神经网络中,数据从输入层向前传递至输出层,此过程称为前向传播。在前向传播中,每个神经元的输入信号经过加权求和后,通过激活函数产生输出信号,再传递给下一层。
## 1.2 梯度与参数更新
反向传播的关键在于通过损失函数计算出损失值的梯度。梯度表示损失函数相对于网络参数的变化率,这些梯度用于指导权重的更新。更新规则通常遵循梯度下降算法的负梯度方向,即每次迭代将参数更新为减去学习率乘以梯度的值。
```python
# 梯度下降法参数更新示例
theta = theta - learning_rate * gradient
```
了解了反向传播算法的基础概念后,我们可以进一步探讨深度学习中的反向传播理论。
# 2. 深度学习中的反向传播理论
深度学习的核心之一是反向传播算法,它是一种用于训练神经网络的算法,通过计算损失函数相对于网络参数的梯度来优化网络。本章将从神经网络的前向传播原理开始,逐步深入到损失函数、参数更新以及梯度下降算法,为我们后续实现深度学习模型打下坚实的理论基础。
## 2.1 神经网络前向传播原理
在深入反向传播之前,理解前向传播原理是不可或缺的。前向传播是指信号在神经网络中的传递过程,从输入层开始,经过各隐藏层处理,最后到达输出层。
### 2.1.1 激活函数的作用与类型
激活函数是神经网络中极其重要的组成部分,它负责加入非线性因素,使得神经网络能够解决更复杂的问题。
#### 激活函数的作用
1. **引入非线性**:激活函数的非线性特性使模型能够学习和执行更加复杂的任务。
2. **选择性激活**:激活函数决定神经元是否应该被激活。
3. **促进学习**:通过梯度下降法更新权重时,激活函数的梯度决定了权重更新的幅度。
#### 激活函数的类型
- **Sigmoid函数**:将输入压缩至0和1之间,但存在梯度消失问题。
\[ \sigma(x) = \frac{1}{1 + e^{-x}} \]
- **Tanh函数**:类似于Sigmoid,但是输出值的范围在-1和1之间,同样存在梯度消失问题。
\[ \tanh(x) = \frac{e^{x} - e^{-x}}{e^{x} + e^{-x}} \]
- **ReLU函数**(Rectified Linear Unit):将所有负值置为零,正数不变。当前最常用的激活函数,因为它解决了梯度消失问题,并且计算简单。
\[ f(x) = \max(0, x) \]
- **Leaky ReLU** 和 **Parametric ReLU** 是 ReLU 的改进版本,用于解决 ReLU 函数在负区间不可导的问题。
### 2.1.2 神经网络层间的连接与数据流动
神经网络的层与层之间是通过权重矩阵相互连接的。每一层的输出是上一层输出与权重矩阵的乘积,然后加上偏置项,最后通过激活函数处理后输出到下一层。
这一过程可以用数学公式表达为:
\[
z^{(l)} = W^{(l)}a^{(l-1)} + b^{(l)} \\
a^{(l)} = g(z^{(l)})
\]
其中 \( z^{(l)} \) 是第 \( l \) 层的加权输入,\( W^{(l)} \) 是权重矩阵,\( a^{(l-1)} \) 是 \( l-1 \) 层的激活值,\( b^{(l)} \) 是偏置向量,\( g(\cdot) \) 是激活函数。
## 2.2 损失函数与反向传播
### 2.2.1 常用损失函数的数学原理
损失函数定义了模型预测值与实际值之间的差异,是学习过程中的重要组成部分。
#### 均方误差(MSE)
对于回归问题,最常用的损失函数是均方误差,它衡量了模型预测值与实际值差的平方的平均值。
\[
MSE = \frac{1}{n} \sum_{i=1}^{n}(y_i - \hat{y}_i)^2
\]
其中 \( y_i \) 是实际值,\( \hat{y}_i \) 是预测值,\( n \) 是样本数量。
#### 交叉熵损失(Cross-Entropy)
对于分类问题,交叉熵损失是比较常用的损失函数,它衡量了模型输出的概率分布与实际标签的概率分布之间的差异。
\[
CE = -\frac{1}{n}\sum_{i=1}^{n} \sum_{c=1}^{M} y_{ic} \log(p_{ic})
\]
其中 \( y_{ic} \) 是标签为 \( c \) 的第 \( i \) 个样本的实际值(通常为0或1),\( p_{ic} \) 是模型预测属于类别 \( c \) 的概率,\( M \) 是类别数。
### 2.2.2 反向传播的数学推导
反向传播算法的核心在于通过链式法则计算损失函数相对于每个参数的偏导数,即梯度。
以一个简单的两层网络为例,假设 \( L \) 为损失函数,\( w \) 为权重,\( x \) 为输入,\( a \) 为激活值,我们有:
\[
\frac{\partial L}{\partial w} = \frac{\partial L}{\partial a} \cdot \frac{\partial a}{\partial z} \cdot \frac{\partial z}{\partial w}
\]
其中 \( z \) 是神经元的加权输入,\( a \) 是激活函数的输出。对于每个参数 \( w \),计算出梯度后,我们就可以使用梯度下降算法来更新参数了。
## 2.3 参数更新与梯度下降算法
### 2.3.1 梯度下降算法的基本概念
梯度下降算法是一种迭代优化算法,用于找到函数的局部最小值。基本思想是从一个初始点开始,计算损失函数在该点的梯度,并沿梯度反方向进行一步(或几步)移动,以期望减少损失。
梯度下降的基本步骤如下:
1. 初始化参数 \( w \) 和学习率 \( \alpha \)。
2. 计算损失函数关于参数 \( w \) 的梯度 \( \nabla_w L \)。
3. 更新参数 \( w \):\( w = w - \alpha \nabla_w L \)。
4. 重复步骤2和3,直到收敛。
### 2.3.2 动量与自适应学习率的优化方法
#### 动量(Momentum)
动量法通过加入惯性使得参数更新更加平滑,避免在梯度较小时震荡。
更新规则变为:
\[
v = \gamma v - \alpha \nabla_w L \\
w = w + v
\]
其中 \( v \) 代表速度(velocity),\( \gamma \) 是动量参数(通常设为0.9)。
#### 自适应学习率
传统的梯度下降中,所有参数使用相同的学习率,这在实际应用中往往效率不高。因此,自适应学习率优化算法应运而生,例如Adam算法。
Adam算法结合了动量法和RMSprop的优点,它不仅考虑了梯度的一阶矩估计,还考虑了二阶矩估计。
更新规则:
\[
m_t = \beta_1 m_{t-1} + (1 - \beta_1) \nabla_w L \\
v_t = \beta_2 v_{t-1} + (1 - \beta_2) (\nabla_w L)^2 \\
w = w - \frac{\alpha}{\sqrt{v_t + \epsilon}} m_t
\]
其中 \( m_t \) 和 \( v_t \) 分别是梯度的一阶和二阶矩估计,\( \epsilon \) 是一个很小的常数以避免分母为零,\( \beta_1 \) 和 \( \beta_2 \) 是衰减率参数。
以上就是深度学习中反向传播算法的一些理论基础。在实际应用中,反向传播算法的实现需要依赖于强大的编程能力,特别是对数学原理的精确理解和算法的精确编码。在下一章中,我们将通过Python来实现基础的反向传播算法,并通过案例加深对这些理论知识的理解。
# 3. Python实现反向传播基础
## 3.1 Python环境与库的搭建
为了实现反向传播算法,我们需要配置适当的Python环境和安装必要的库。在这一小节中,我们将介绍如何安装NumPy和TensorFlow,以及如何使用这些库来搭建我们的第一个神经网络。
### 3.1.1 安装与配置NumPy和TensorFlow
NumPy是Python中用于科学计算的核心库,提供高性能的多维数组对象和这些数组的操作工具。TensorFlow是由Google开发的开源机器学习库,特别适合大规模的深度学习模型。首先,确保Python环境已经安装。
对于NumPy,可以使用pip进行安装:
```bash
pip install numpy
```
安装TensorFlow之前,建议先更新pip到最新版本以避免兼容性问题:
```bash
pip install --upgrade pip
```
然后安装TensorFlow:
```bash
pip install tensorflow
```
安装完成后,可以在Python中进行检查:
```python
import numpy as np
import tensorflow as tf
print(np.__version__)
print(tf.__version__)
```
### 3.1.2 创建基础的神经网络结构
安装好NumPy和TensorFlow后,我们可以开始创建我们的第一个神经网络。下面的代码展示了如何构建一个简单的神经网络结构,包含一个输入层、一个隐藏层和一个输出层:
```python
import tensorflow as tf
# 定义神经网络结构
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=64, activation='relu', input_shape=(10,)), # 输入层及隐藏层,使用ReLU激活函数
tf.keras.layers.Dense(units=10, activation='softmax') # 输出层,使用Softmax激活函数
])
# 编译模型
***pile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 打印模型概况
model.summary()
```
在上面的代码中,我们使用了`tf.keras.Sequential`来创建一个顺序模型,并添加了两个全连接层。`input_shape`参数指定了输入层的大小。`units`参数表示每层的神经元数量,`activation`参数指定了激活函数。模型使用了Adam优化器和交叉熵损失函数进行编译,这对于分类问题是一个很好的选择。
## 3.2 简单线性回归的反向传播
### 3.2.1 实现线性回归模型
线性回归是最简单的模型之一,其目标是找到最佳的权重系数,使得模型预测结果与实际数据之间的差异最小化。下面的代码展示了一个简单的线性回归模型的实现:
```python
import numpy as np
import tensorflow as tf
# 创建一组简单的数据
X = np.array([1, 2, 3, 4, 5], dtype=np.float32)
y = np.array([5, 7, 9, 11, 13], dtype=np.float32)
# 创建模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=1, input_shape=(1,))
])
# 编译模型
***pile(optimizer='sgd', loss='mse')
# 训练模型
model.fit(X, y, epochs=1000, verbose=0)
# 预测结果
print(model.predict(X))
```
在这段代码中,我们首先创建了一组简单的一维数据集,并使用`tf.keras.Sequential`构建了一个只有一个神经元的全连接层的模型,即一个线性回归模型。我们使用了随机梯度下降(SGD)优化器和均方误差(MSE)损失函数。通过调用`fit`方法,我们让模型在数据上训练了1000个周期(epochs)。
### 3.2.2 编写反向传播算
0
0
相关推荐
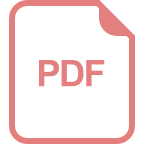
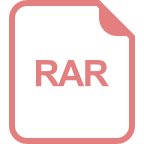
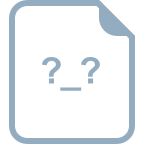
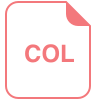
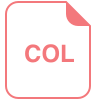
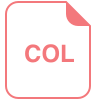
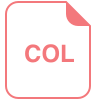

