【机器学习在文本挖掘中的应用】:算法实践与案例分析
发布时间: 2024-09-07 20:14:10 阅读量: 244 订阅数: 40 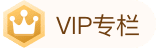
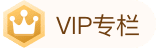
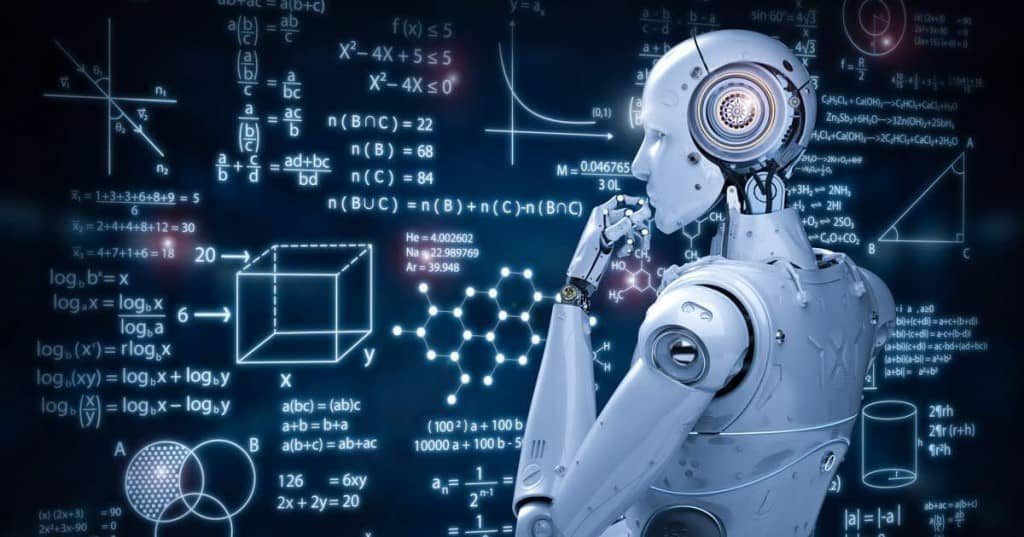
# 1. 文本挖掘与机器学习基础
## 1.1 文本挖掘的概念与重要性
文本挖掘是利用机器学习和统计学等方法对非结构化的文本数据进行结构化处理的过程,旨在从大量的文本数据中提取有价值的信息和知识。随着数字化信息的爆炸性增长,文本挖掘已经成为处理数据和挖掘数据中隐含意义的强大工具。
## 1.2 机器学习与文本挖掘的关系
机器学习是文本挖掘的核心技术之一。它通过算法从数据中学习模式和规律,用于文本的分类、聚类、情感分析、主题发现等任务。机器学习模型能够处理的语言数据复杂性,赋予了文本挖掘更深层次的分析能力。
## 1.3 文本挖掘的步骤概述
文本挖掘的过程通常包括数据的收集、预处理、特征提取、模型构建、评估与优化等步骤。每个步骤都对最终结果产生重要影响,合理的流程设计可以显著提高挖掘效率和准确性。
为了确保文本挖掘的高效执行,接下来的章节将详细探讨文本数据的预处理技巧以及机器学习算法在文本挖掘中的应用。
# 2. 文本数据预处理技巧
## 2.1 文本数据的收集与清洗
### 2.1.1 网络爬虫技术基础
网络爬虫是文本数据收集的利器,是自动化网页信息抓取的脚本或程序。通过模拟浏览器行为,爬虫可以高效地从互联网上搜集海量文本数据。在设计爬虫时,一般需要考虑以下几个基本要素:
- **请求处理**:爬虫通过发送HTTP请求到目标服务器,获取响应内容。
- **数据解析**:解析响应的HTML或JSON等格式数据,提取出所需信息。
- **数据存储**:将提取的数据保存至文件或数据库中。
- **异常处理**:对网络异常、请求超时、数据解析错误等进行处理。
- **反爬虫策略应对**:需要识别并应对目标网站可能设置的反爬机制,如IP限制、验证码等。
下面是一个简单的Python爬虫示例代码,使用了requests库获取网页内容,并用BeautifulSoup进行解析:
```python
import requests
from bs4 import BeautifulSoup
def simple_web_crawler(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# 提取特定元素,例如页面中的所有段落文本
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.get_text())
else:
print('Failed to retrieve the webpage')
# 使用爬虫抓取指定网页内容
simple_web_crawler('***')
```
在这个脚本中,我们首先设置了请求头,模拟浏览器请求,以避免被简单地拒绝服务。然后通过requests库向目标URL发送HTTP GET请求,并通过BeautifulSoup解析返回的HTML内容,提取并打印出所有段落文本。
### 2.1.2 文本数据清洗的常用方法
文本数据通常需要经过清洗才能用于进一步的分析和挖掘。清洗步骤可能包括以下几个方面:
- **去除特殊字符**:例如HTML标签、JavaScript代码等。
- **分词**:将长字符串分解为单词或短语。
- **转换为小写**:统一文本格式,减少重复和歧义。
- **去除停用词**:停用词如“的”、“是”、“在”等,对文本意义贡献较小。
- **词干提取或词形还原**:将单词还原到基本形式。
- **纠正拼写错误**:提高文本质量。
接下来,我们将进行一个具体的文本清洗示例,使用Python中的nltk库来去除停用词。
```python
import nltk
from nltk.corpus import stopwords
nltk.download('stopwords')
nltk.download('punkt')
# 示例文本
text = "NLTK is a leading platform for building Python programs to work with human language data."
# 分词
tokens = nltk.word_tokenize(text)
# 加载英文停用词列表
stop_words = set(stopwords.words('english'))
# 去除停用词
filtered_tokens = [word for word in tokens if word.lower() not in stop_words]
print(filtered_tokens)
```
在这个例子中,我们首先导入了nltk库,并下载了英文停用词列表。然后对给定的文本字符串进行了分词处理。通过对分词结果应用列表推导式,我们过滤掉所有出现在停用词列表中的词汇,得到了清洗后的单词列表。
## 2.2 文本特征提取方法
### 2.2.1 词袋模型与TF-IDF
文本数据被清洗之后,接下来需要将其转化为计算机能够处理的数值型数据。词袋模型(Bag of Words, BoW)是将文本转换为特征向量的一种方法,其中每个唯一的词都对应一个特征维度,通过统计词频来表示其在文本中的重要性。
TF-IDF(Term Frequency-Inverse Document Frequency)是一种用于信息检索和文本挖掘的常用加权技术,可以对词袋模型中的特征进行权衡,降低常见词对模型的影响。
让我们来看看如何用Python实现一个简单的TF-IDF计算:
```python
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.feature_extraction.text import CountVectorizer
# 示例文本
documents = [
'NLTK is a leading platform for building Python programs to work with human language data.',
'The Natural Language Toolkit is a Python library supporting NLP.',
'It is called NLTK.'
]
# 使用词袋模型进行向量化
vectorizer = CountVectorizer()
transformed_documents = vectorizer.fit_transform(documents)
# 计算TF-IDF
tfidf_vectorizer = TfidfVectorizer()
tfidf_matrix = tfidf_vectorizer.fit_transform(transformed_documents)
print(tfidf_matrix.toarray())
```
在这个例子中,我们首先使用了`CountVectorizer`来实现词袋模型,然后应用`TfidfVectorizer`来将词频转化为TF-IDF权重。`fit_transform`方法不仅拟合了模型,还转换了原始文本数据。
### 2.2.2 词嵌入技术与Word2Vec
词嵌入是将单词转换为实数向量的技术,是目前最流行的文本表示方法。词嵌入模型通过学习大量的文本数据,将语义上相似的词映射到距离相近的向量空间。Word2Vec是其中最著名的模型之一,使用神经网络学习词向量表示。
Word2Vec模型有两种训练方式:CBOW(Continuous Bag of Words)和Skip-Gram。CBOW预测目标词,基于它的上下文;Skip-Gram则相反,目标是预测一个词的上下文,基于目标词。
下面是一个使用gensim库训练Word2Vec模型的简单例子:
```python
import gensim.downloader as api
# 下载预先训练好的Word2Vec模型
model = api.load('word2vec-google-news-300')
# 示例文本
words = ['NLTK', 'Python', 'language', 'data']
# 使用训练好的模型获取词向量
vectors = [model[word] for word in words]
print(vectors)
```
在这个例子中,我们首先加载了预先训练好的Word2Vec模型,然后获取了几个单词的向量表示。这些向量可以用于后续的文本分析任务。
### 2.2.3 主题模型LDA的原理与应用
LDA(Latent Dirichlet Allocation)是一种文档主题生成模型,用于发现文档集合中的隐含主题。LDA模型假定每个文档是由多个主题按照一定的比例混合而成的,每个主题又由多个词汇按照一定的比例组成。
LDA模型可以通过多项式分布或狄利克雷分布来描述文档、主题和词汇之间的概率关系。文档集合中的每个文档都对应一组主题分布,每个主题对应一组词汇分布。
让我们看看如何使用Python的gensim库实现LDA模型:
```python
from gensim import corpora
from gensim.models.ldamodel import LdaModel
from nltk.corpus import stopwords
nltk.download('stopwords')
# 示例文本
documents = [
"NLTK is a leading platform for building Python programs to work with human language data.",
"The Natural Language Toolkit is a Python library supporting NLP.",
"It is called NLTK."
]
# 清洗文本
stoplist = stopwords.words('english')
texts = [[word for word in document.lower().split() if word not in stoplist] for document in documents]
# 构建词典
dictionary = corpora.Dictionary(texts)
# 构建语料库
corpus = [dictionary.doc2bow(text) for text in texts]
# 使用LDA模型
lda = LdaModel(corpus, id2word=dictionary, num_topics=2)
print(lda.print_topics(num_words=4))
```
在这个例子中,首先对文本数据进行了简单的清洗处理,然后创建了一个词典和语料库。之后,我们使用LDA模型对文档集合进行了主题生成,最后打印了两个主题及其对应的关键词。
## 2.3 文本数据的维度缩减
### 2.3.1 主成分分析(PCA)在文本数据中的应用
主成分分析(PCA)是一种常用的降维技术,它通过正交变换将一组可能相关的变量转换成一组线性不相关的变量,称为主成分。主成分保留了原始数据中的大部分变异(信息),通常用于可视化和数据预处理。
在文本数据中,PCA可以用来减少高维词向量空间的维度。这对于可视化和减少后续模型训练时间特别有用。
下面是一个使用PCA对词嵌入数据降维并可视化(以二维散点图形式)的示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
from sklearn.manifold import TSNE
# 假设我们已经从文本中提取了一些词的词向量
word_vectors = np.array([
# 词向量示例数据
])
# 将词向量标准化(零均值,单位方差)
word_vectors_normalized = (word_vectors - np.mean(word
```
0
0
相关推荐
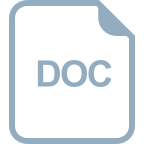
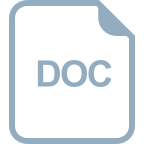
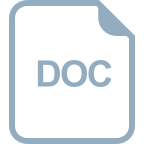
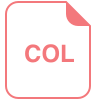
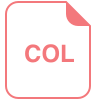
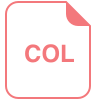
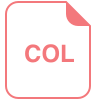
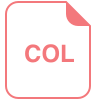
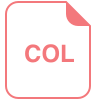