【实战演练】使用PyMongo开发MongoDB应用
发布时间: 2024-06-27 14:26:34 阅读量: 72 订阅数: 121 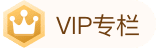
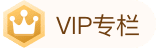
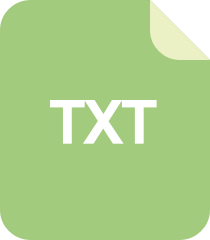
深入浅出MongoDB应用实战开发视频教学
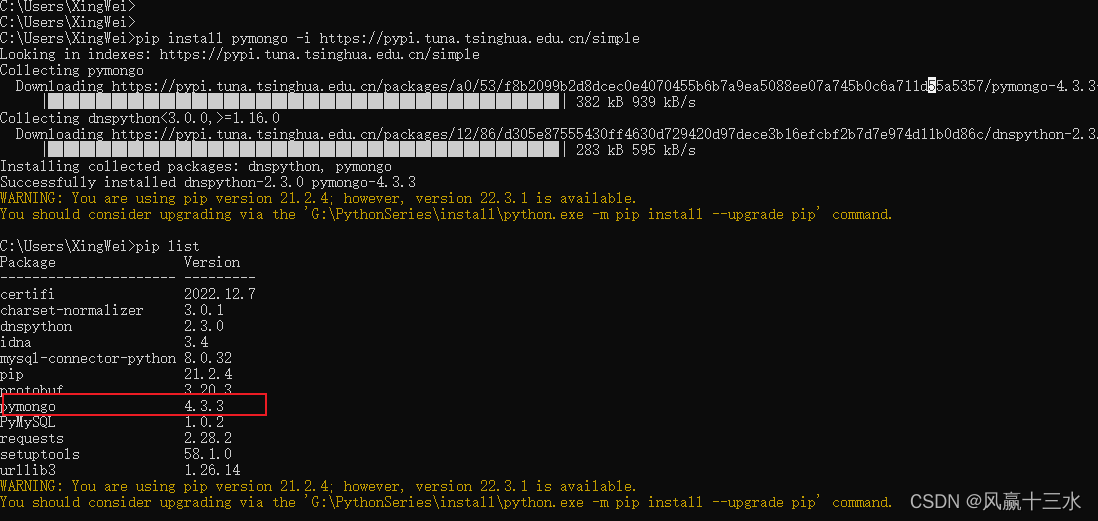
# 2.1 PyMongo的安装和使用
PyMongo是Python语言的MongoDB驱动程序,它允许Python程序与MongoDB数据库进行交互。要安装PyMongo,可以使用以下命令:
```
pip install pymongo
```
安装完成后,可以通过以下代码导入PyMongo模块:
```python
import pymongo
```
要连接到MongoDB数据库,可以使用`MongoClient`类:
```python
client = pymongo.MongoClient("mongodb://localhost:27017")
```
其中,`localhost`是MongoDB服务器的地址,`27017`是默认端口号。连接成功后,可以通过`client`对象访问数据库和集合。
# 2. PyMongo编程基础
### 2.1 PyMongo的安装和使用
**安装 PyMongo**
在 Python 环境中安装 PyMongo 非常简单,可以使用 pip 命令:
```
pip install pymongo
```
**导入 PyMongo**
安装完成后,可以在 Python 代码中导入 PyMongo 模块:
```python
import pymongo
```
**连接 MongoDB**
要连接到 MongoDB 数据库,需要使用 `MongoClient` 类:
```python
client = pymongo.MongoClient("mongodb://localhost:27017")
```
其中,`localhost` 为 MongoDB 服务器地址,`27017` 为默认端口号。
### 2.2 PyMongo的数据操作
#### 2.2.1 数据库和集合的创建与删除
**创建数据库**
```python
db = client.test_db
```
**创建集合**
```python
collection = db.test_collection
```
**删除数据库**
```python
client.drop_database("test_db")
```
**删除集合**
```python
collection.drop()
```
#### 2.2.2 文档的插入、查询和更新
**插入文档**
```python
post = {"title": "Hello World", "content": "This is my first post"}
collection.insert_one(post)
```
**查询文档**
```python
for post in collection.find({"title": "Hello World"}):
print(post)
```
**更新文档**
```python
collection.update_one({"title": "Hello World"}, {"$set": {"content": "This is my updated post"}})
```
### 2.3 PyMongo的查询语言
#### 2.3.1 查询条件的构建
**比较运算符**
```python
collection.find({"age": {"$gt": 18}}) # 查找年龄大于 18 的文档
```
**逻辑运算符**
```python
collection.find({"$or": [{"age": {"$gt": 18}}, {"name": "John"}]}) # 查找年龄大于 18 或姓名为 John 的文档
```
**正则表达式**
```python
collection.find({"name": {"$reg
```
0
0
相关推荐
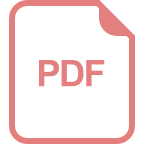
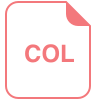
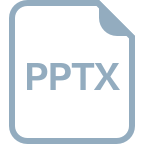
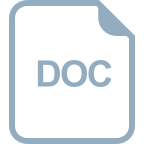
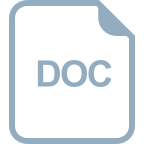
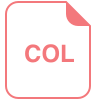
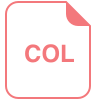