:提升递归效率:尾递归优化的神奇力量
发布时间: 2024-08-25 14:31:53 阅读量: 11 订阅数: 19 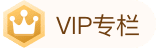
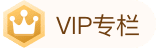
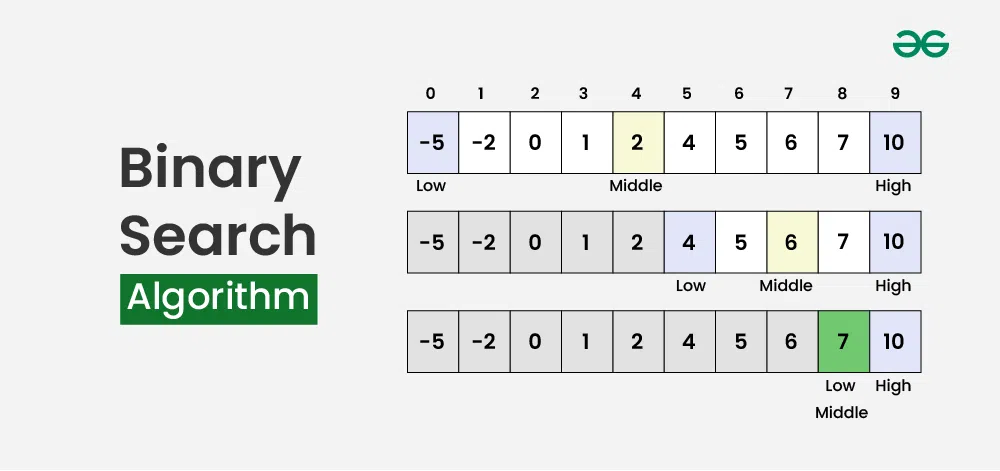
# 1. 递归的本质与优化策略
递归是一种强大的编程技术,它允许函数调用自身。虽然递归可以简化代码,但它也可能导致堆栈溢出等性能问题。为了解决这些问题,可以使用优化策略,例如尾递归优化。
尾递归优化是一种编译器技术,它将递归调用转换为循环,从而消除堆栈溢出的风险。尾递归优化的关键在于识别尾递归,即函数在自身调用后没有其他操作。通过将尾递归转换为循环,编译器可以避免创建新的堆栈帧,从而提高性能。
# 2. 尾递归优化原理
### 2.1 尾递归的定义和识别
**定义:**
尾递归是指函数在自身调用后,作为最后一步直接返回该调用的结果。
**识别方法:**
识别尾递归可以遵循以下步骤:
1. **确定函数的基线条件:**基线条件是函数终止调用的条件。
2. **检查函数的最后一步:**如果函数的最后一步是直接返回自身调用的结果,则该函数是尾递归。
### 2.2 尾递归优化的原理和实现方式
**原理:**
尾递归优化利用了编译器的优化技术,将尾递归调用转换为循环。这避免了函数调用的开销,从而提高了执行效率。
**实现方式:**
编译器通常通过以下方式实现尾递归优化:
1. **检测尾递归调用:**编译器识别尾递归调用并将其标记为可优化。
2. **将尾递归转换为循环:**编译器将尾递归调用转换为循环,从而避免了函数调用的开销。
3. **使用循环寄存器:**编译器使用循环寄存器来存储循环变量,从而进一步提高了效率。
**代码示例:**
以下代码展示了尾递归优化的实现:
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
```
在该代码中,`factorial` 函数是尾递归的。编译器会将尾递归调用转换为循环,从而提高执行效率。
**逻辑分析:**
`factorial` 函数通过递归调用自身来计算阶乘。如果 `n` 为 0,则函数返回 1。否则,函数将 `n` 与自身调用 `factorial(n - 1)` 的结果相乘,并返回该乘积。
**参数说明:**
* `n`:要计算阶乘的非负整数。
**扩展性说明:**
尾递归优化对于具有深度递归调用的函数特别有效。它可以显著提高执行效率,并减少内存开销。
# 3. 尾递归优化实践
### 3.1 斐波那契数列的尾递归优化
斐波那契数列是一个经典的递归问题,其定义如下:
```python
def fib(n):
if n <= 1:
return n
else:
return fib(n-1) + fib(n-2)
```
这个递归实现存在尾递归调用的问题,因为递归调用出现在函数的末尾。我们可以通过以下步骤将其优化为尾递归形式:
1. **引入一个辅助函数:**引入一个辅助函数 `fib_tail`,它将接受两个参数:`n` 和一个累加器 `acc`。
2. **修改递归调用:**在 `fib_tail` 函数中,将递归调用替换为对自身函数的调用,并传递更新后的 `n` 和 `acc` 值。
3. **返回累加器:**在 `fib_tail` 函数中,返回累加器 `acc` 作为结果。
优化后的尾递归实现如下:
```python
def fib_tail(n, acc):
if n <= 1:
return acc
else:
return fib_tail(n-1, acc+n-1)
def fib(n):
return fib_tail(n, 0)
```
### 3.2 汉诺塔问题的尾递归优化
汉诺塔问题是一个经典的递归问题,其定义如下:
```
将 n 个圆盘从 A 柱移动到 C 柱,中间使用 B 柱作为辅助。每次只能移动一个圆盘,并且不能将较大的圆盘放在较小的圆盘上。
```
这个递归实现也存在尾递归调用的问题,因为递归调用出现在函数的末尾。我们可以通过以下步骤将其优化为尾递归形式:
1. **引入一个辅助函数:**引入一个辅助函数 `hanoi_tail`,它将接受三个参数:`n`、`from` 和 `to`。
2. **修改递归调用:**在 `hanoi_tail` 函数中,将递归调用替换为对自身函数的调用,并传递更新后的 `n`、`from` 和 `to` 值。
3. **返回空值:**
0
0
相关推荐
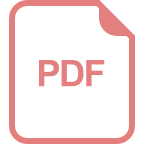
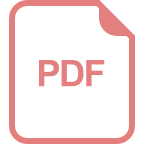
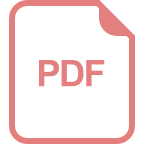
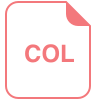
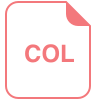
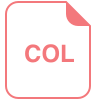

