贪心算法在图论中的妙用:最短路径与最大匹配问题轻松搞定
发布时间: 2024-08-24 14:50:05 阅读量: 31 订阅数: 36 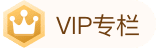
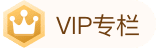
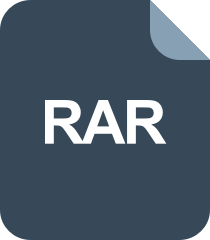
探索Dijkstra算法:贪心策略在最短路径中的妙用
.png)
# 1. 图论基础
图论是计算机科学中研究图结构及其性质的学科。图是一种数据结构,由顶点(节点)和边(连接顶点的线)组成。图论在计算机科学的许多领域都有应用,包括网络、数据库和算法。
图论中的一些基本概念包括:
- **顶点**:图中的基本单位,表示图中的对象。
- **边**:连接两个顶点的线段,表示顶点之间的关系。
- **度**:顶点连接的边的数量。
- **路径**:顶点之间的有序顶点序列。
- **连通性**:两个顶点之间是否存在路径。
# 2. 贪心算法的基本原理
### 2.1 贪心算法的定义和特点
贪心算法是一种自顶向下的启发式算法,它在解决问题时,总是做出当前看来最优的选择,而不管这种选择对未来可能产生的影响。
**特点:**
* **局部最优性:**贪心算法只考虑当前的局部最优解,而不考虑全局最优解。
* **迭代性:**贪心算法通过迭代的方式逐步逼近最优解。
* **不可回溯性:**一旦做出选择,贪心算法不能回溯到之前的状态。
* **时间复杂度低:**贪心算法通常具有较低的时间复杂度,适合处理大规模问题。
### 2.2 贪心算法的适用场景
贪心算法适用于以下场景:
* **局部最优解即全局最优解:**当问题具有子问题最优解即全局最优解的性质时,贪心算法可以得到最优解。
* **决策相互独立:**当问题中各个子问题的决策相互独立时,贪心算法可以有效地解决问题。
* **规模较大:**当问题规模较大,难以使用穷举法或动态规划等方法求解时,贪心算法可以提供近似最优解。
**示例:**
考虑一个求解背包问题的场景,背包容量为 W,有 n 个物品,每个物品有重量 w_i 和价值 v_i。贪心算法可以按照以下步骤求解:
```python
def greedy_knapsack(W, items):
"""贪心算法求解背包问题
Args:
W: 背包容量
items: 物品列表,每个物品包含重量和价值
Returns:
背包中物品的价值和
"""
# 按价值密度(价值/重量)降序排列物品
items.sort(key=lambda item: item.value / item.weight, reverse=True)
total_value = 0
current_weight = 0
# 遍历物品
for item in items:
# 如果背包还有剩余容量
if current_weight + item.weight <= W:
# 将物品放入背包
total_value += item.value
current_weight += item.weight
else:
# 背包容量不足,跳过当前物品
break
return total_value
```
**逻辑分析:**
* 贪心算法按照价值密度降序排列物品,优先选择价值密度较高的物品放入背包。
* 算法遍历物品,只要背包有剩余容量,就将当前价值密度最高的物品放入背包。
* 算法的复杂度为 O(n log n),其中 n 为物品的数量。
# 3. 贪心算法在最短路径问题中的应用
### 3.1 最短路径问题的定义和求解方法
**定义:**
最短路径问题是指在给定一个带权有向图或无向图中,求解从一个指定的起点到其他所有顶点的最短路径。
**求解方法:**
常用的最短路径求解算法有:
- **迪杰斯特拉算法:**适用于非负权重的有向图或无向图。
- **贝尔曼-福特算法:**适用于有负权重的有向图。
- **弗洛伊德-沃舍尔算法:**适用于所有类型的图,但时间复杂度较高。
### 3.2 贪心算法求解最短路径问题的步骤和示例
**步骤:**
1. 初始化一个优先队列,将起点加入优先队列。
2. 重复以下步骤,直到优先队列为空:
- 从优先队列中取出权重最小的顶点 `v`。
- 对于 `v` 的所有邻接顶点 `u`:
- 如果 `u` 不在优先队列中,则将其加入优先队列。
- 如果存在从起点到 `u` 的路径,且通过 `v` 的路径更短,则更新 `u` 的最短路径和父节点。
**示例:**
给定一个带权有向图:
```
A -> B (1)
A -> C (2)
B -> C (3)
C -> D (4)
```
求解从顶点 `A` 到所有其他顶点的最短路径:
**初始化:**
- 优先队列:`[A(0)]`
**步骤 1:**
- 取出权重最小的顶点 `A`。
**步骤 2:**
- 对于 `A` 的邻接顶点 `B` 和 `C`:
- `B` 不在优先队列中,加入优先队列:`[B(1)]`
- `C` 不在优先队列中,加入优先队列:`[C(2)]`
**步骤 3:**
- 取出权重最小的顶点 `B`。
**步骤 4:**
- 对于 `B` 的邻接顶点 `C`:
- `C` 已在优先队列中,但通过 `B` 的路径更短,更新 `C` 的最短路径:`C(4) -> C(3)`
**步骤 5:**
- 取出权重最小的顶点 `C`。
**步骤 6:**
- 对于 `C` 的邻接顶点 `D`:
- `D` 不在优先队列中,加入优先队列:`[D(7)]`
**结果:**
- 从 `A` 到 `B` 的最短路径:`A -> B (1)`
- 从 `A` 到 `C` 的最短路径:`A -> C (2)`
- 从 `A` 到 `D` 的最短路径:`A -> C -> D (6)`
**代码示例:**
```python
import heapq
class Graph:
def __init__(self):
self.edges = {}
def add_edge(self, u, v, weight):
if u not in self.edges:
self.edges[u] = []
self.
```
0
0
相关推荐
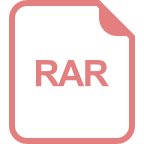
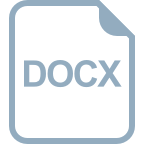
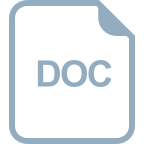
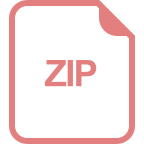
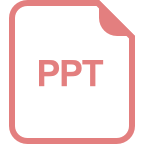
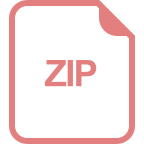
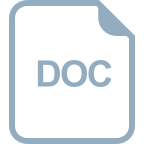