贪心算法在数据结构中的应用:解锁高效解决方案
发布时间: 2024-08-24 14:47:29 阅读量: 12 订阅数: 11 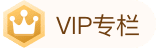
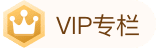
# 1. 贪心算法简介
贪心算法是一种解决优化问题的经典算法,其特点是每次做出局部最优的选择,以期最终得到全局最优解。贪心算法的适用场景是问题满足以下条件:
- **局部最优解可以推导出全局最优解**:即每次贪心选择局部最优解,最终得到的解就是全局最优解。
- **问题具有子结构最优性**:即问题的子问题最优解可以用来构造问题的最优解。
# 2. 贪心算法在数据结构中的应用原理
### 2.1 贪心算法的定义和特点
贪心算法是一种在每一步中做出局部最优选择,以期得到全局最优解的算法。其主要特点如下:
- **局部最优性:**贪心算法在每一步中选择当前可行的最优解,而不会考虑未来可能产生的影响。
- **渐进性:**贪心算法逐步构建解,每一小步都基于上一步的决策。
- **不可回溯性:**一旦做出选择,贪心算法不会回溯或修改之前的决策。
### 2.2 贪心算法的适用场景
贪心算法适用于满足以下条件的问题:
- **局部最优即全局最优:**局部最优决策必然导致全局最优解。
- **子问题独立:**问题的子问题之间相互独立,不会影响整体解。
- **无后效性:**当前决策不会影响未来的决策。
**代码块:**
```python
def greedy_algorithm(problem):
"""
贪心算法通用框架
参数:
problem: 问题实例
返回:
贪心算法得到的解
"""
solution = []
while problem.has_next_step():
current_step = problem.get_next_step()
solution.append(current_step)
problem.update(current_step)
return solution
```
**逻辑分析:**
该代码块提供了贪心算法的通用框架。它以问题实例为输入,并逐步执行以下步骤:
1. 检查问题实例中是否有可行的下一步。
2. 如果有,则选择当前可行的最优下一步。
3. 将该步骤添加到解中。
4. 更新问题实例,反映该步骤的影响。
5. 重复步骤 1-4,直到问题实例中没有可行的下一步。
**参数说明:**
- `problem`: 问题实例,包含问题描述、可行步骤和更新规则。
**扩展性说明:**
贪心算法的通用框架可以应用于各种数据结构问题。通过实现特定的 `problem` 类,可以解决不同的问题。例如,在栈和队列中应用贪心算法时,`problem` 类可以表示栈或队列的数据结构和操作。
# 3. 贪心算法在栈和队列中的应用
### 3.1 贪心算法在栈中的应用
#### 3.1.1 栈的贪心算法示例:括号匹配
栈是一种后进先出的数据结构,其特点是只能从栈顶进行元素的压入和弹出操作。贪心算法在栈中的应用主要体现在括号匹配问题上。
括号匹配问题是指给定一个由括号组成的字符串,判断该字符串中的括号是否匹配。括号匹配的规则是:
- 左括号必须与右括号匹配
- 括号必须成对出现,不能出现孤立的括号
贪心算法解决括号匹配问题的方法是:
1. 初始化一个空栈
2. 遍历给定的字符串
3. 如果遇到左括号,则将其压入栈中
4. 如果遇到右括号,则检查栈顶元素是否为左括号。如果是,则弹出栈顶元素,表示一对括号匹配。如果不是,则说明括号不匹配
5. 遍历结束后,如果栈为空,则说明所有括号都匹配。否则,说明括号不匹配
**代码块:**
```python
def is_bracket_matched(string):
stack = []
for char in string:
if char in ['(', '[', '{']:
stack.append(char)
elif char in [')', ']', '}']:
if not stack or char != ')':
return False
stack.pop()
return not stack
```
**逻辑分析:**
代码首先初始化一个空栈,然后遍历给定的字符串。对于每个字符,如果它是左括号,则将其压入栈中。如果它是右括号,则检查栈顶元素是否为左括号。如果是,则弹出栈顶元素,表示一对括号匹配。如果不是,则说明括号不匹配。遍历结束后,如果栈为空,则说明所有括号都匹配。否则,说明括号不匹配。
**参数说明:**
- `string`:给定的由括号组成的字符串
### 3.2 贪心算法在队列中的应用
#### 3.2.1 队列的贪心算法示例:任务调度
队列是一种先进先出的数据结构,其特点是只能从队尾进行元素的入队和从队首进行元素的出队操作。贪心算法在队列中的应用主要体现在任务调度问题上。
任务调度问题是指给定一组任务,每个任务都有一个到达时间和一个执行时间,在满足以下条件的情况下调度这些任务:
- 任务只能按照到达时间的顺序执行
- 每个
0
0
相关推荐
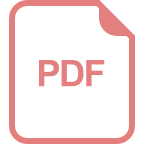
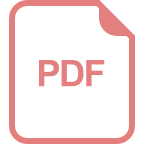
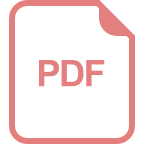





