图可视化技术实战:Java图形化表示绘制技巧
发布时间: 2024-09-11 04:00:34 阅读量: 75 订阅数: 43 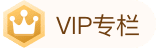
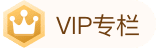
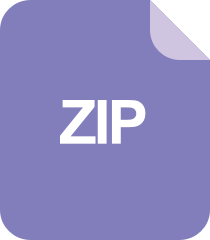
2IV35-project:可视化
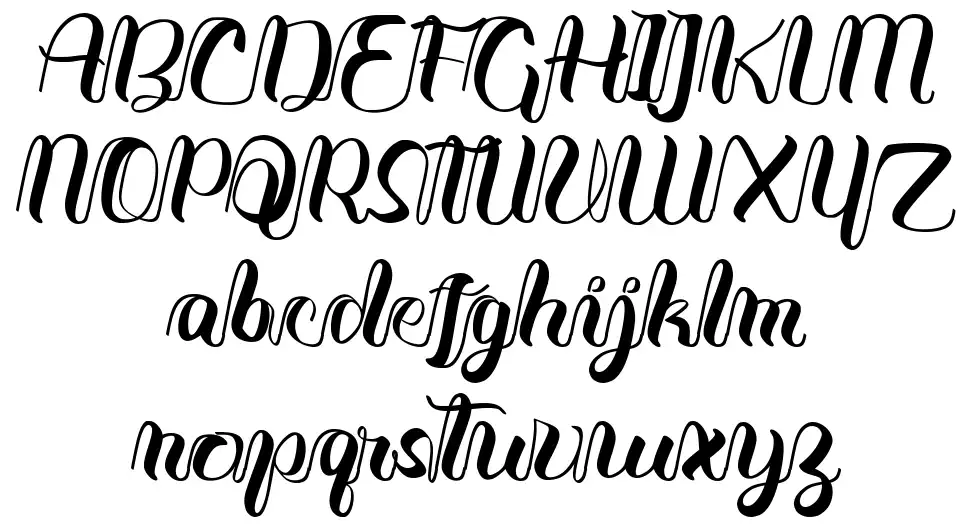
# 1. 图可视化技术基础
图可视化技术是将复杂的数据关系以图形的方式直观展示出来,这对于数据分析和理解复杂系统至关重要。本章将介绍图可视化的基础概念和重要性,为后续章节中在Java语言环境下实现图可视化技术打下坚实的理论基础。
## 1.1 图可视化的目的与意义
图可视化的目的在于简化复杂的信息结构,使用户能够迅速把握数据之间的关系和模式。无论是社交网络分析、生物信息学、物流管理还是网络监控,图可视化技术都能够帮助用户以图形的形式直观理解数据,从而做出更加科学的决策。其意义不仅在于提供视觉上的便利,更在于挖掘潜在的信息和趋势。
## 1.2 图可视化的基本元素
图是由节点(顶点)和边(连接)组成的,节点代表实体,边代表实体间的关系。在图可视化中,节点和边是基本的构建块。节点可以通过大小、形状、颜色来表示不同的属性,而边可以表示连接的权重、类型等特性。这些元素的合理组合和表达是图可视化设计的核心。
## 1.3 图可视化的应用场景
图可视化技术的应用范围非常广泛,它包括但不限于社交网络分析、网络拓扑结构展示、知识图谱构建、生物信息学中的基因网络分析等。每一种应用场景都可能需要不同类型的图(如有向图、无向图、加权图等),以及特定的布局和交互方式,以达到最佳的可视化效果。
```mermaid
graph LR
A[图可视化基础] -->|目的与意义| B[信息简化与模式识别]
A -->|基本元素| C[节点和边的可视化]
A -->|应用场景| D[社交网络、网络拓扑等]
```
上述内容仅为第一章内容的概述,其后各章节将深入探讨Java语言中的图可视化实践和高级应用。
# 2. Java中的图形绘制理论
### 2.1 Java图形基础
#### 2.1.1 Java图形API概览
Java通过AWT (Abstract Window Toolkit) 和Swing库为开发者提供了丰富的图形用户界面(GUI)组件和基本的图形绘制能力。这些API共同构成了Java的图形基础,使开发者能够创建和操作窗口、按钮、文本框等GUI元素,以及在这些元素上绘制各种图形。
在AWT中,所有界面元素都由`java.awt`包中的类来表示,而`Graphics`类是所有绘图操作的核心。`Graphics`类提供了绘制线条、矩形、圆形、多边形等基本几何图形的方法,同时也支持文本和图像的绘制。
对于更复杂的图形绘制需求,Java提供了`java.awt.geom`包,其中包含了一系列的几何形状和操作这些形状的类。例如,`GeneralPath`类可以用来构造复杂的路径和形状,而`Area`类则允许对形状进行布尔运算。
##### 示例代码
```java
import java.awt.Color;
import java.awt.Graphics;
public class SimpleDrawing extends javax.swing.JComponent {
public void paintComponent(Graphics g) {
super.paintComponent(g);
// 设置颜色并绘制矩形
g.setColor(Color.BLUE);
g.fillRect(10, 20, 100, 50);
// 绘制圆形
g.setColor(Color.RED);
g.fillOval(150, 100, 100, 100);
}
public static void main(String[] args) {
javax.swing.JFrame frame = new javax.swing.JFrame("Simple Drawing");
frame.setDefaultCloseOperation(javax.swing.JFrame.EXIT_ON_CLOSE);
frame.add(new SimpleDrawing());
frame.setSize(350, 250);
frame.setVisible(true);
}
}
```
此代码段创建了一个简单的组件,其中包含用蓝色填充的矩形和红色填充的圆形。通过覆盖`paintComponent`方法,我们可以在该组件上进行自定义绘图。
#### 2.1.2 基本图形元素的绘制
绘制基本图形元素是构建复杂图形和界面的基础。在Java中,`Graphics`类提供了多种方法来绘制不同类型的图形元素,包括但不限于点、线、矩形、圆形、椭圆和多边形等。
- **绘制线条**:`drawLine(int x1, int y1, int x2, int y2)`方法用于绘制两点之间的连线。其中`(x1, y1)`和`(x2, y2)`分别是线段起点和终点的坐标。
- **绘制矩形**:`drawRect(int x, int y, int width, int height)`方法用于绘制一个矩形框。其中`(x, y)`是矩形左上角的坐标,`width`和`height`分别是矩形的宽度和高度。
- **绘制圆形**:`drawOval(int x, int y, int width, int height)`方法用于绘制一个椭圆,其外接矩形的位置和尺寸由参数指定。如果外接矩形是正方形,则绘制出的是一个圆形。
- **绘制多边形**:`drawPolygon(int[] xPoints, int[] yPoints, int nPoints)`方法用于绘制一个由多个点定义的多边形。其中`xPoints`和`yPoints`数组分别包含多边形顶点的x和y坐标,`nPoints`是顶点的数量。
绘制这些图形元素时,可以通过修改`Graphics`对象的状态来实现不同的效果。例如,改变颜色、笔刷样式和线宽等。
### 2.2 图形绘制的坐标系统
#### 2.2.1 坐标系统简介
在Java中,图形绘制是在一个虚拟的二维平面上进行的,这个平面被称为“用户空间”。用户空间中的坐标原点`(0, 0)`位于组件左上角,X轴正方向指向右方,Y轴正方向指向下方。这种坐标系统被称为笛卡尔坐标系。
Java的坐标系统允许开发者进行坐标转换和缩放,从而实现图形的精确放置和调整大小。组件的绘制表面上,开发者可以自由地定义坐标系,使其适应应用程序的需要。
#### 2.2.2 坐标转换和缩放技巧
要改变图形的绘制位置或大小,可以使用坐标转换和缩放功能。`Graphics`类提供了以下方法:
- **平移**:`translate(int x, int y)`方法可以将坐标原点移动到一个新的位置。这对图形位置的绝对调整非常有用。
- **缩放**:`scale(double x, double y)`方法可以根据给定的因子放大或缩小图形。`x`是水平方向的缩放因子,`y`是垂直方向的缩放因子,大于1表示放大,小于1表示缩小。
除了这些基础操作外,还可以使用`Graphics2D`类中的方法进行更高级的图形变换。`Graphics2D`是`Graphics`类的子类,它提供了渲染2D图形的功能。使用`Graphics2D`可以实现旋转、倾斜和复杂的仿射变换。
##### 示例代码
```java
import java.awt.Graphics;
import java.awt.Graphics2D;
import javax.swing.JComponent;
public class TransformationsComponent extends JComponent {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g;
g2d.setTransform(java.awt.geom.AffineTransform.getScaleInstance(2.0, 2.0));
g2d.fillRect(10, 10, 50, 50); // 绘制一个缩放后的矩形
}
}
```
在这个示例中,我们通过设置`Graphics2D`对象的变换属性,使得后续绘制的所有图形都被自动缩放为原来的两倍。
### 2.3 Java图形绘制的高级话题
#### 2.3.1 反走样和颜色模式
图形学中的反走样技术,也称为抗锯齿技术,用于平滑图形边缘,避免呈现锯齿状,尤其是在绘制斜线和曲线时。Java通过`Graphics`类中的`setRenderingHint()`方法提供了简单的反走样功能。
```java
import java.awt.Graphics;
import java.awt.RenderingHints;
import javax.swing.JComponent;
public class AntiAliasingComponent extends JComponent {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// 设置渲染提示来启用反走样
g.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g.fillOval(10, 10, 100, 100); // 使用反走样绘制圆形
}
}
```
Java中的颜色模式通常指的是颜色的表示方法。在Java中,颜色是通过`java.awt.Color`类来表示的,支持RGB(红绿蓝)、HSB(色相、饱和度、亮度)等颜色模式。颜色可以通过这些模式的不同参数来创建,并且可以用来填充图形或者设置文本颜色。
```java
import java.awt.Graphics;
import java.awt.Color;
import javax.swing.JComponent;
public class ColorModeComponent extends JComponent {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// 使用RGB模式创建颜色并填充矩形
Color redColor = new Color(255, 0, 0);
g.setColor(redColor);
g.fillRect(10, 10, 100, 50);
}
}
```
#### 2.3.2 硬件加速和图形渲染
随着计算机硬件的发展,许多Java图形绘制操作可以利用GPU进行加速,这在处理复杂的图形和动画时尤为重要。从Java 9开始,Java虚拟机(JVM)开始支持硬件加速渲染。
要启用硬件加速,可以通过在创建窗口时传递特定的属性来实现。然而,需要注意的是,硬件加速的可用性依赖于操作系统和驱动程序的支持,并不是在所有平台上都能开启。
```java
import javax.swing.JFrame;
public class HardwareAccelerationExample {
public static void main(String[] args) {
// 启用硬件加速
System.setProperty("sun.java2d.opengl", "true");
JFrame frame = new JFrame("Hardware Acceleration Example");
frame.setSize(300, 300);
frame.setVisible(true);
}
}
```
请注意,上述代码仅是一个示例,实际上启用硬件加速可能需要更多的配置,且在不同Java版本上可能会有不同的支持情况。
在本章节中,我们介绍了Java图形绘制的基础概念、坐标系统、以及如何进行反走样处理和颜色模式的使用。同时,我们还探讨了硬件加速如何为图形绘制带来性能上的提升。这些知识点对于深入理解Java中的图形绘制机制和性能优化至关重要,也为第三章的图可视化实践打下了坚实的基础。
# 3. 使用Java进行图可视化实践
## 3.1 图的表示方法
### 3.1.1 邻接矩阵与邻接表
在图数据结构的表示中,邻接矩阵和邻接表是两种基础且常用的方法。它们各自具有不同的特点和适用场景,能够影响到后续的图操作和算法实现。
- **邻接矩阵(Adjacency Matrix)**
邻接矩阵是用一个二维数组来表示图中所有顶点之间的连接关系。如果顶点`i`和顶点`j`之间存在一条边,则`matrix[i][j]`的值为1(或边的权重),否则为0。邻接矩阵易于实现且能够快速判断任意两个顶点之间是否存在边,但空间复杂度较高,尤其在顶点数多边数少的稀疏图中浪费空间明显。
- **邻接表(Adjacency List)**
与邻接矩阵相比,邻接表通过链表或数组列表来存储每条边的信息,每个顶点有一个对应的链表,存储与之相连的所有顶点。邻接表节省空间,更适合表示稀疏图,并且能够快速迭代出某顶点的所有邻居,但遍历图中所有边可能需要额外的计算。
接下来,展示如何在Java中实现这两种图的表示方式,并进行比较分析。
```java
import java.util.*;
// 邻接矩阵表示图
public class GraphMatrix {
private int[][] adjMatrix;
private int numVertices;
public GraphMatrix(int numVertices) {
this.numVertices = numVertices;
adjMatrix = new int[numVertices][numVertices];
}
public void addEdge(int source, int dest) {
```
0
0
相关推荐
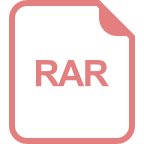
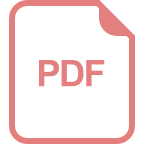
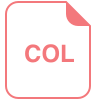
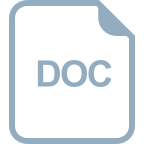
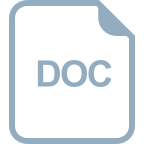
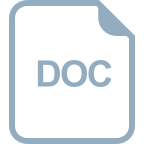
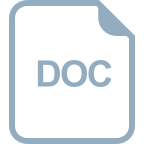
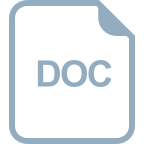