图的存储优化攻略:空间复杂度降低与压缩技术
发布时间: 2024-09-11 03:45:42 阅读量: 70 订阅数: 47 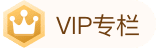
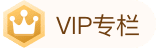
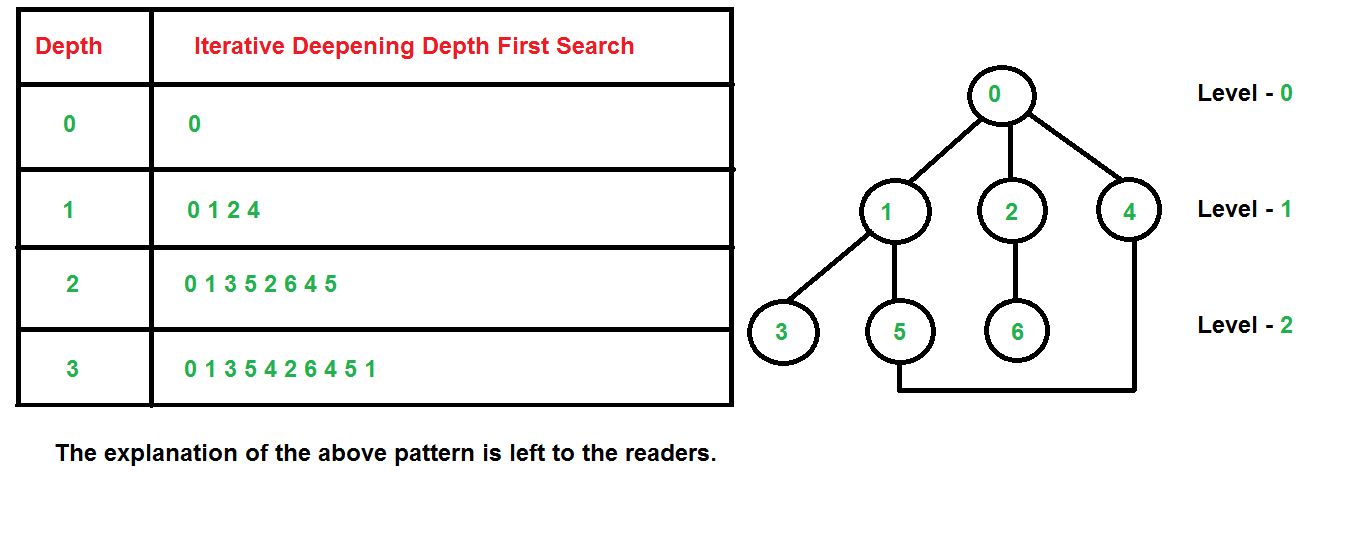
# 1. 图的存储基础与优化概述
在信息技术快速发展的今天,图数据的存储和优化方法对于数据科学家和工程师来说至关重要。图作为一种强大的数据结构,被广泛应用于社交网络分析、网络路由、生物信息学和推荐系统等领域。本章将带你了解图的基本存储原理,并概述如何优化这些存储结构以提高效率和性能。
## 1.1 图的存储基础
图由节点(顶点)和边组成,用于描述实体间的复杂关系。在计算机科学中,图的存储基础是将这些实体和关系转化为可操作的数据结构。有几种主要的图存储方法,包括邻接矩阵和邻接表,它们各有优势和不足。邻接矩阵适合密集图,而邻接表则更适合稀疏图,因为它能有效地表示节点之间的关系,同时节省空间。
## 1.2 存储优化的重要性
随着图数据规模的增长,存储和处理这些数据的效率成为了一个挑战。数据量的增加不仅导致内存消耗增大,还可能降低图遍历和查询的速度。因此,图的存储优化成为了提升大数据处理性能的关键步骤。优化的目的是减少内存占用,提高访问速度,并且减少计算资源的消耗。下一章节我们将深入探讨邻接矩阵和邻接表的优化策略。
# 2. 图的存储结构优化
## 2.1 图的邻接矩阵存储
### 2.1.1 理解邻接矩阵存储方法
邻接矩阵是图的一种直观而基本的存储方法。在邻接矩阵中,图的所有顶点由矩阵的行和列代表,而矩阵中的元素则表示顶点之间的连接关系。对于无向图来说,邻接矩阵是对称的;而有向图的邻接矩阵则不一定对称。具体到每个元素,如果顶点 i 和顶点 j 之间有边,则对应的矩阵元素值为 1;如果没有边,则为 0。
在图数据的处理中,邻接矩阵非常便于判断任意两个顶点之间是否存在边。同时,邻接矩阵表示法使得算法设计更加直观。然而,它也存在空间复杂度较高的问题,尤其是对于稀疏图来说,大部分的空间可能都是在存储无用信息。
```python
# 示例代码展示邻接矩阵的创建过程
def create_adjacency_matrix(num_vertices, edges):
matrix = [[0] * num_vertices for _ in range(num_vertices)]
for (src, dest) in edges:
matrix[src][dest] = 1
matrix[dest][src] = 1 if not directed else 0 # 无向图对称填充,有向图只填充单向
return matrix
num_vertices = 5
edges = [(0, 1), (0, 2), (1, 2), (2, 3), (3, 4)]
matrix = create_adjacency_matrix(num_vertices, edges)
# 打印矩阵以示例输出
for row in matrix:
print(row)
```
在上面的代码中,我们定义了一个`create_adjacency_matrix`函数,它接受顶点的数量和边的列表作为输入,并返回一个邻接矩阵。我们假设图中的边都是无向的。该矩阵用于表示图中的连接关系,对于无向图来说是对称的。
### 2.1.2 邻接矩阵的压缩技术
由于邻接矩阵的空间复杂度较高,特别是在稀疏图中,有效的压缩技术显得尤为重要。邻接矩阵压缩的目的是减少存储空间的需求。一种常见的方法是仅存储邻接矩阵的上三角或下三角,因为矩阵是对称的,这可以减少一半的存储需求。对于有向图,可以只存储矩阵的上三角部分。此外,还可以使用位图和稀疏矩阵表示法,如使用一维数组存储非零元素。
```python
def compress_adjacency_matrix(matrix):
compressed = []
for i in range(len(matrix)):
row = matrix[i]
compressed_row = ''.join(str(row[j]) for j in range(i + 1, len(row)))
compressed.append(int(compressed_row, 2)) # 转换为整型存储
return compressed
compressed_matrix = compress_adjacency_matrix(matrix)
print(compressed_matrix)
```
上述代码实现了对邻接矩阵的压缩。此方法适合无向图,它仅保存了邻接矩阵的下三角部分,并将每一行的下三角部分转换成二进制整数。这种压缩减少了存储需求,特别是当图非常稀疏时效果更佳。
## 2.2 图的邻接表存储
### 2.2.1 理解邻接表存储方法
邻接表是一种更为节约空间的图存储结构,尤其适合于稀疏图的表示。它由顶点数组和边链表数组组成。顶点数组中的每个元素对应图中的一个顶点,而边链表数组的每个元素则包含从对应顶点出发的所有边的列表。邻接表不仅节省空间,而且提供了比邻接矩阵更高效的遍历性能。
邻接表适合那些需要经常对图进行遍历的算法,因为它的数据结构设计能够迅速地访问顶点的邻接顶点。然而,在邻接表中查找顶点间是否存在边则较为复杂,因为需要遍历边列表。
```python
class Vertex:
def __init__(self, value):
self.value = value
self.edge_to = []
class Edge:
def __init__(self, vertex, weight=1):
self.vertex = vertex
self.weight = weight
class AdjacencyList:
def __init__(self, vertices):
self.vertices = [Vertex(vertex) for vertex in vertices]
def add_edge(self, src, dest):
edge = Edge(self.vertices[dest])
self.vertices[src].edge_to.append(edge)
# 对于有向图,还需要添加下面这行
# self.vertices[dest].edge_to.append(Edge(self.vertices[src], weight))
def print_adjacency_list(self):
for vertex in self.vertices:
print(f"{vertex.value}: {[(edge.vertex.value, edge.weight) for edge in vertex.edge_to]}")
adj_list = AdjacencyList(['A', 'B', 'C', 'D', 'E'])
adj_list.add_edge(0, 1)
adj_list.add_edge(0, 2)
adj_list.add_edge(1, 3)
adj_list.add_edge(2, 3)
adj_list.add_edge(3, 4)
adj_list.print_adjacency_list()
```
在上述代码中,我们定义了一个简单的邻接表结构。每个顶点都保存了一个边的列表,这样可以方便地访问每个顶点的所有邻接顶点。
### 2.2.2 邻接表的优化策略
尽管邻接表本身已经很节省空间,我们还可以采取进一步的优化策略来改善性能。一个常见的优化手段是使用链表的替代数据结构,比如跳表或哈希表。跳表可以加快搜索速度,而哈希表可以将顶点到其边列表的映射时间降低到常数时间。
```python
class OptimizedAdjacencyList:
def __init__(self, vertices):
self.vertices = {vertex: [] for vertex in vertices}
def add_edge(self, src, dest):
self.vertices[src].append(dest)
def print_adjacency_list(self):
for vertex, edges in self.vertices.items():
print(f"{vertex}: {edges}")
# 使用优化后的邻接表
optimized_adj_list = OptimizedAdjacencyList(['A', 'B', 'C', 'D', 'E'])
optimized_adj_list.add_edge(0, 1)
optimized_adj_list.add_edge(0, 2)
optimized_adj_list.add_edge(1, 3)
optimized_adj_list.add_edge(2, 3)
optimized_adj_list.add_edge(3, 4)
optimized_adj_list.print_adjacency_list()
```
在优化后的邻接表实现中,我们使用Python字典来代替数组。这允许我们以O(1)的时间复杂度访问任何顶点的边列表。这种结构对于动态图来说尤其有用,其中顶点和边经常被添加或删除。
## 2.3 图的边存储表示
### 2.3.1 边存储表示的优势与不足
边存储表示是另一种图数据的存储方式,它只记录图中的边而不记录所有顶点。这种表示法在处理大规模稀疏图时尤其有用,因为它只需要存储图中实际存在的边,从而极大地减少了存储空间的需求。然而,它的缺点是失去了对顶点信息的直接访问,可能会使得某些图操作变得复杂。
在边存储表示中,通常会有一个边数组,其中每个元素包含了边的起点、终点和(可选的)边的权重。这种结构对于一些特定的图算法来说非常有效,比如图的遍历、生成树的搜索等。
```python
class EdgeStorage:
def __init__(self, edges):
self.edges = edges # 顶点对列表
def print_edges(self):
for edge in self.edges:
print(f"{edge[0]} -- {edge[1]}")
```
在上述代码中,我们定义了一个简单的边存储表示。它只记录了图中的边,并且可以快速地遍历所有边。不过,这种方法无法立即知道图中某个顶点的所有邻接顶点。
### 2.3.2 实践中的边存储优化技巧
在实际应用中,我们可以对边存储方式进行多种优化以适应不同的场景。一种常见的优化方法是将边存储到文件系统中,而不是内存中。这种方法使得边存储表示能够处理非常大的图数据集,因为数据的读取是按需加载的。
此外,可以将边存储在一个数据库中,并通过索引提高访问效率。这种方法特别适合于数据需要频繁更新和查询的场景。还可以实现边的归档压缩,通过一些压缩算法减少边存储的大小,如行程长度编码(Run-Length Encoding, RLE)。
```python
import csv
import gzip
def read_edge_list_from_f
```
0
0
相关推荐
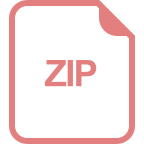
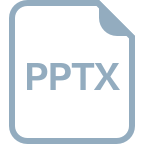






