Matlab Axis Translation Guide: Optimizing Layout, Enhancing Data Readability
发布时间: 2024-09-13 22:32:15 阅读量: 24 订阅数: 23 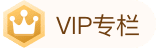
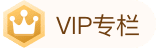
# 1. An Overview of Axis Translation in Matlab
Axis translation is an important graphical operation in Matlab that allows users to adjust the range and position of the axes, providing a better display of the data. By translating axes, users can control the way data is displayed on the graph, thereby optimizing the data visualization effect and improving data readability.
There are multiple methods to achieve axis translation, including the use of `xlim()` and `ylim()` functions, `set()` function, and `axis()` function. These functions offer different parameters and options, enabling users to customize axis translation operations according to specific needs.
# 2. Theoretical Foundation of Axis Translation
### 2.1 Coordinate Systems and Transformation Matrices
**Coordinate Systems**
A coordinate system is a mathematical tool used to describe spatial positions, consisting of an origin and a set of basis vectors. In two-dimensional space, the Cartesian coordinate system is commonly used, with its origin at `(0, 0)` and its basis vectors being the `x` and `y` axes.
**Transformation Matrices**
A transformation matrix is a mathematical tool used to perform operations such as translation, rotation, and scaling on a coordinate system. The expression for a two-dimensional translation transformation matrix `T` is as follows:
```
T = [1 0 tx;
0 1 ty;
0 0 1]
```
Here, `tx` and `ty` represent the translation distances along the `x` and `y` axes, respectively.
### 2.2 Mathematical Principles of Translation Transformation
Translation transformation refers to the operation of translating the coordinate system by a certain distance in a specified direction. The mathematical principle is as follows:
Let point `P(x, y)` be in the original coordinate system, and let point `P'(x', y')` be in the translated coordinate system. The coordinates after translation are:
```
x' = x + tx
y' = y + ty
```
where `tx` and `ty` are the translation distances.
Translation transformation can be achieved by multiplying the transformation matrix `T` by the homogeneous coordinates `[x, y, 1]` of point `P` in the original coordinate system:
```
[x', y', 1] = [x, y, 1] * T
```
**Code Block 1: Mathematical Principles of Translation Transformation**
```matlab
% Point in the original coordinate system
x = 2;
y = 3;
% Translation distances
tx = 1;
ty = 2;
% Transformation matrix
T = [1 0 tx;
0 1 ty;
0 0 1];
% Translation transformation
P_prime = [x, y, 1] * T;
% Output the translated coordinates
disp('Translated coordinates:');
disp(P_prime);
```
**Logical Analysis:**
This code demonstrates the mathematical principles of translation transformation. It first defines the point `P` in the original coordinate system and the translation distances `tx` and `ty`. Then, it creates the transformation matrix `T`. Finally, it multiplies `P` by `T` to obtain the translated coordinates `P'`.
**Parameter Description:**
* `x`: x-coordinate of the point in the original coordinate system
* `y`: y-coordinate of the point in the original coordinate system
* `tx`: Translation distance along the x-axis
* `ty`: Translation distance along the y-axis
* `T`: Transformation matrix
* `P_prime`: Translated coordinates
# 3.1 Translating Axes Using xlim() and ylim() Functions
The `xlim()` and `ylim()` functions are used in Matlab to set the range of the x-axis and y-axis of the axes. By modifying the parameters of these functions, axis translation can be achieved.
**xlim() Function**
The syntax of the `xlim()` function is:
```
xlim([xmin xmax])
```
Here,
0
0
相关推荐
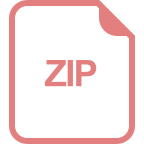
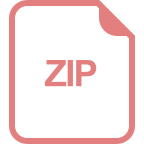