Matlab Axis Rotation Guide: Adapting to Needs for More Comprehensive Data Visualization
发布时间: 2024-09-13 22:28:33 阅读量: 19 订阅数: 21 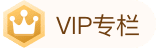
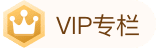
# Guide to Rotating Axes in Matlab: Adapting to Needs for More Comprehensive Data Visualization
## 1. Overview of Matlab Coordinate Axis Rotation
Axis rotation plays a crucial role in Matlab data visualization, allowing users to view and explore data from different angles. This chapter will introduce the basic concepts of axis rotation, including rotation matrices, coordinate system transformations, and rotation operations. By understanding these fundamental concepts, users can effectively leverage Matlab for complex data visualization.
## 2. Theoretical Foundations of Coordinate Axis Rotation
### 2.1 Rotation Matrices and Their Geometric Meaning
**Rotation Matrix**
A rotation matrix is an orthogonal matrix used to represent rotation transformations around a specific axis. It is defined by the following formula:
```
R = [cos(θ) -sin(θ) 0]
[sin(θ) cos(θ) 0]
[0 0 1]
```
Where:
* θ is the rotation angle around the specified axis.
* R[i, j] represents the element in the i-th row and j-th column of the rotation matrix.
**Geometric Meaning**
The geometric meaning of the rotation matrix can be understood as follows:
* The first row of the matrix represents the new coordinates of the unit vector along the x-axis after rotation.
* The second row of the matrix represents the new coordinates of the unit vector along the y-axis after rotation.
* The third row of the matrix represents the new coordinates of the unit vector along the z-axis after rotation.
### 2.2 Coordinate System Transformations and Rotation
Coordinate system transformation is the process of converting points from one coordinate system to another. Rotation transformation is a special type of coordinate system transformation that involves rotating the coordinate system around a specific axis.
**Coordinate System Transformation Formula**
The coordinate system transformation formula is as follows:
```
P' = R * P
```
Where:
* P is a point in the original coordinate system.
* P' is a point in the rotated coordinate system.
* R is the rotation matrix.
**Geometric Meaning of Rotation Transformation**
The geometric meaning of rotation transformation can be understood as follows:
* The rotation transformation rotates points in the original coordinate system around the specified axis by θ degrees.
* The rotated points are located in a new coordinate system that is rotated θ degrees relative to the original coordinate system around the specified axis.
**Code Example**
The following MATLAB code demonstrates how to rotate points around the z-axis using a rotation matrix:
```
% Define rotation angle
theta = pi/4;
% Define rotation matrix
R = [cos(theta) -sin(theta) 0;
sin(theta) cos(theta) 0;
0 0 1];
% Define original point
P = [1; 2; 3];
% Rotate point
P_rotated = R * P;
% Display rotated point
disp(P_rotated);
```
**Code Logic Analysis**
* The code first defines the rotation angle θ.
* Then, it uses `cos()` and `sin()` functions to create the rotation matrix R.
* Next, it defines the original point P.
* Then, it uses the rotation matrix R to rotate the point P, obtaining the rotated point P_rotated.
* Finally, it displays the rotated point P_rotated.
**Parameter Description**
* `theta`: Rotation angle (in radians)
* `R`: Rotation matrix
* `P`: Original point
* `P_rotated`: Rotated point
## 3. Practical Operations of Coordinate Axis Rotation
### 3.1 Using the `view` Function for Basic Rotation
The `view` function is the basic function in MATLAB for setting the graphical viewpoint. It accepts three parameters: azimuth, elevation, and tilt, in degrees. Azimuth represents the angle rotated counterclockwise from the positive x-axis direction, elevation represents the angle rotated counterclockwise from the xy-plane, and tilt represents the angle rotated counterclockwise from the positive z-axis direction.
```
figure;
plot3(x, y, z);
view(30, 45, 20); % Set azimuth to 30 degrees, elevation to 45 degrees, tilt to 20 degrees
```
0
0
相关推荐
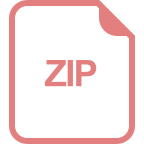
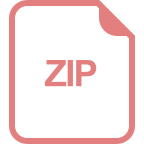
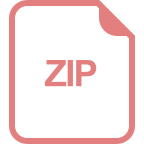
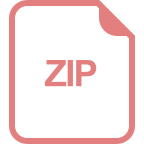
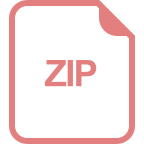
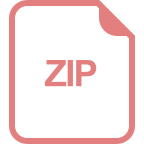
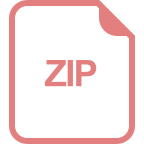
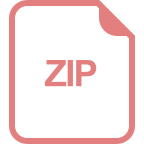
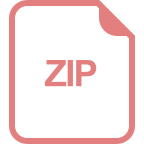