"Python 3 对象导向编程" 在Python编程中,面向对象编程(Object-Oriented Programming,简称OOP)是一种强大的编程范式,它允许开发者通过创建和使用对象来组织代码,提高可读性和可维护性。Python 3 对象导向编程的核心概念包括类(Class)、对象(Object)、继承(Inheritance)、封装(Encapsulation)和多态(Polymorphism)。 1. 类(Class):类是创建对象的蓝图或模板,它定义了一组属性(Attributes)和方法(Methods)。在Python中,你可以使用`class`关键字来定义一个类。例如: ```python class Person: def __init__(self, name, age): self.name = name self.age = age def introduce(self): print(f"My name is {self.name} and I am {self.age} years old.") ``` 在这个例子中,`Person`类有两个属性(`name`和`age`)以及一个方法(`introduce`)。 2. 对象(Object):基于类创建的实例称为对象。通过调用类的构造函数(如上面的`__init__`),我们可以创建一个新对象。例如: ```python john = Person("John", 30) john.introduce() # 输出: My name is John and I am 30 years old. ``` 3. 继承(Inheritance):继承允许我们创建一个新类,该类从现有类(基类或父类)继承其属性和方法。这有助于代码重用和构建分层的类结构。例如: ```python class Student(Person): def __init__(self, name, age, grade): super().__init__(name, age) self.grade = grade student = Student("Alice", 18, "Grade 12") student.introduce() # 输出: My name is Alice and I am 18 years old. ``` 4. 封装(Encapsulation):封装是将数据和操作这些数据的方法绑定在一起的过程,以保护数据不被外部直接访问。在Python中,我们通常使用私有属性(前缀`_`)和getter/setter方法实现封装。例如: ```python class BankAccount: def __init__(self, initial_balance=0): self.__balance = initial_balance def deposit(self, amount): if amount > 0: self.__balance += amount def withdraw(self, amount): if amount <= self.__balance: self.__balance -= amount @property def balance(self): return self.__balance ``` 5. 多态(Polymorphism):多态允许我们使用一个接口来代表不同类的实例。Python通过方法重写(Override)实现多态,子类可以覆盖父类的方法,赋予其不同的实现。例如: ```python class Animal: def sound(self): pass class Dog(Animal): def sound(self): print("Woof!") class Cat(Animal): def sound(self): print("Meow!") def make_sound(animal): animal.sound() dog = Dog() cat = Cat() make_sound(dog) # 输出: Woof! make_sound(cat) # 输出: Meow! ``` Python 3 对象导向编程的实践还包括元类(Metaclasses)、抽象基类(Abstract Base Classes, ABCs)和属性装饰器等高级主题。通过熟练掌握这些概念,开发者可以创建出更加灵活、可扩展的代码库,满足各种复杂的需求。
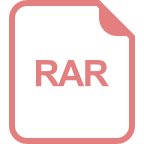

















- 粉丝: 4
- 资源: 99
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- AirKiss技术详解:无线传递信息与智能家居连接
- Hibernate主键生成策略详解
- 操作系统实验:位示图法管理磁盘空闲空间
- JSON详解:数据交换的主流格式
- Win7安装Ubuntu双系统详细指南
- FPGA内部结构与工作原理探索
- 信用评分模型解析:WOE、IV与ROC
- 使用LVS+Keepalived构建高可用负载均衡集群
- 微信小程序驱动餐饮与服装业创新转型:便捷管理与低成本优势
- 机器学习入门指南:从基础到进阶
- 解决Win7 IIS配置错误500.22与0x80070032
- SQL-DFS:优化HDFS小文件存储的解决方案
- Hadoop、Hbase、Spark环境部署与主机配置详解
- Kisso:加密会话Cookie实现的单点登录SSO
- OpenCV读取与拼接多幅图像教程
- QT实战:轻松生成与解析JSON数据

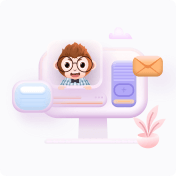
