Java面向对象编程第五版简介

"面向对象编程与Java第5版,作者C. Thomas Wu,是一本针对初学者详细讲解Java编程的书籍。" 本书是《面向对象编程与Java》的第五版,作者C. Thomas Wu,他在美国海军研究生院任职。这本书是Java编程的入门教程,适合对编程感兴趣或刚刚接触Java语言的读者。书中详细介绍了面向对象编程的基本概念和原理,旨在帮助读者掌握Java编程的基础知识。 在Java编程中,面向对象编程(Object-Oriented Programming, OOP)是一种核心的编程范式,它以对象为中心,强调数据和操作数据的方法的封装、继承和多态性。以下是一些关键的知识点: 1. **类与对象**:类是创建对象的蓝图,它定义了对象的属性(数据成员)和行为(方法)。对象是类的实例,具有类所定义的特征和功能。 2. **封装**:封装是将数据和操作数据的方法捆绑在一起的过程,防止外部代码直接访问对象的内部细节,保证数据的安全性。 3. **继承**:继承允许子类(派生类)继承父类(基类)的属性和方法,这样可以减少代码重复,提高代码复用性。 4. **多态性**:多态性是指相同的操作或函数可以作用于不同的对象,使得代码更具灵活性和扩展性。Java中的接口和抽象类都是实现多态的方式。 5. **基础语法**:书中会涵盖Java的基础语法,如变量声明、数据类型、控制流语句(if、for、while)、数组等。 6. **异常处理**:Java的异常处理机制有助于程序在遇到错误时能够优雅地处理,避免程序崩溃。 7. **集合框架**:Java集合框架包括List、Set、Map等接口和ArrayList、LinkedList、HashSet、HashMap等实现类,它们提供了存储和管理对象的容器。 8. **输入/输出(I/O)**:Java的I/O系统支持读写文件、网络通信等,包括File类、InputStream/OutputStream接口及其子类。 9. **线程与并发**:Java提供了对多线程的支持,通过Thread类和Runnable接口可以创建并运行线程,理解并发编程是编写高效Java程序的关键。 10. **泛型**:泛型是Java 5引入的新特性,用于增强类型安全,减少类型转换的繁琐工作。 11. **Java Swing与GUI编程**:Java Swing库提供了一组组件用于构建图形用户界面,包括按钮、文本框、菜单等。 12. **Java标准库API**:介绍如何使用Java标准库中的各种类和接口,如Math类、Date类、Collections类等。 13. **JDBC与数据库连接**:Java数据库连接(JDBC)API用于连接和操作数据库,如执行SQL查询、处理结果集等。 14. **设计模式**:书中可能还会涉及一些常见的设计模式,如单例模式、工厂模式、观察者模式等,这些模式是解决特定问题的通用解决方案。 通过这本书的学习,读者不仅能够掌握Java语言的基础,还能了解到面向对象编程的核心理念,为后续的Java开发工作打下坚实的基础。此外,书中可能还包括练习题和案例研究,以巩固所学知识并提高实践能力。
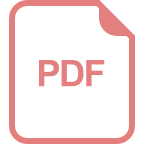
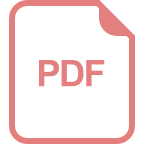

















- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- AirKiss技术详解:无线传递信息与智能家居连接
- Hibernate主键生成策略详解
- 操作系统实验:位示图法管理磁盘空闲空间
- JSON详解:数据交换的主流格式
- Win7安装Ubuntu双系统详细指南
- FPGA内部结构与工作原理探索
- 信用评分模型解析:WOE、IV与ROC
- 使用LVS+Keepalived构建高可用负载均衡集群
- 微信小程序驱动餐饮与服装业创新转型:便捷管理与低成本优势
- 机器学习入门指南:从基础到进阶
- 解决Win7 IIS配置错误500.22与0x80070032
- SQL-DFS:优化HDFS小文件存储的解决方案
- Hadoop、Hbase、Spark环境部署与主机配置详解
- Kisso:加密会话Cookie实现的单点登录SSO
- OpenCV读取与拼接多幅图像教程
- QT实战:轻松生成与解析JSON数据

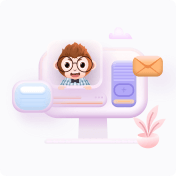
