面向对象之扫雷小游戏代码
时间: 2023-07-02 13:16:38 浏览: 84
好的,下面是一个简单的使用面向对象思想实现的扫雷小游戏的代码示例:
```python
import random
class Square:
def __init__(self, x, y):
self.x = x
self.y = y
self.is_clicked = False
self.has_mine = False
self.adj_mine_count = 0
def set_mine(self):
self.has_mine = True
def set_adj_mine_count(self, count):
self.adj_mine_count = count
def click(self):
self.is_clicked = True
def __str__(self):
if self.is_clicked:
if self.has_mine:
return '*'
else:
return str(self.adj_mine_count)
else:
return '.'
class Board:
def __init__(self, width, height, num_mines):
self.width = width
self.height = height
self.num_mines = num_mines
self.grid = [[Square(x, y) for y in range(height)] for x in range(width)]
self.generate_mines()
def generate_mines(self):
for i in range(self.num_mines):
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
square = self.grid[x][y]
while square.has_mine:
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
square = self.grid[x][y]
square.set_mine()
for adj_square in self.get_adj_squares(square):
adj_square.set_adj_mine_count(adj_square.adj_mine_count + 1)
def get_adj_squares(self, square):
adj_squares = []
for x in range(square.x - 1, square.x + 2):
for y in range(square.y - 1, square.y + 2):
if x >= 0 and x < self.width and y >= 0 and y < self.height and not (x == square.x and y == square.y):
adj_squares.append(self.grid[x][y])
return adj_squares
def click_square(self, x, y):
square = self.grid[x][y]
square.click()
if square.has_mine:
return False
if square.adj_mine_count == 0:
for adj_square in self.get_adj_squares(square):
if not adj_square.is_clicked:
self.click_square(adj_square.x, adj_square.y)
return True
def is_game_over(self):
for x in range(self.width):
for y in range(self.height):
square = self.grid[x][y]
if not square.is_clicked and not square.has_mine:
return False
return True
def __str__(self):
return '\n'.join([''.join([str(self.grid[x][y]) for y in range(self.height)]) for x in range(self.width)])
class Game:
def __init__(self, width, height, num_mines):
self.board = Board(width, height, num_mines)
self.game_over = False
self.num_mines = num_mines
def play(self):
while not self.game_over:
print(self.board)
x = int(input("Enter x coordinate: "))
y = int(input("Enter y coordinate: "))
if not self.board.click_square(x, y):
self.game_over = True
print("Game over! You clicked on a mine.")
elif self.board.is_game_over():
self.game_over = True
print("Congratulations! You have cleared all the squares without clicking on a mine.")
else:
print("Remaining mines: ", self.num_mines - self.count_mines_clicked())
def count_mines_clicked(self):
count = 0
for x in range(self.board.width):
for y in range(self.board.height):
square = self.board.grid[x][y]
if square.has_mine and square.is_clicked:
count += 1
return count
if __name__ == '__main__':
game = Game(8, 8, 10)
game.play()
```
这个代码实现了一个简单的扫雷游戏,使用了面向对象的思想,将方块和游戏板都抽象成了对象,方便维护和拓展。
相关推荐
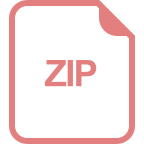
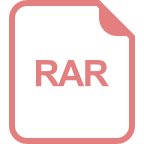
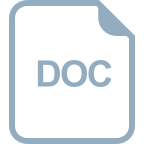
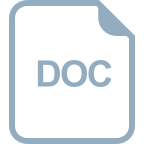
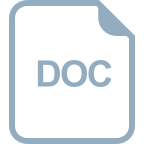
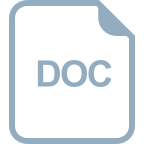
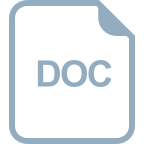









