#include "ns3/core-module.h" using namespace ns3; NS_LOG_COMPONENT_DEFINE ("ScratchSimulator"); int main (int argc, char *argv[]) { NS_LOG_UNCOND ("Scratch Simulator"); CommandLine cmd; cmd.Parse (argc, argv); }
时间: 2024-01-21 21:04:20 浏览: 184
这段代码是用于使用ns-3网络仿真库进行网络仿真的。首先通过#include指令引入ns3核心模块。然后使用命名空间ns3,方便调用其中的函数和类。NS_LOG_COMPONENT_DEFINE是定义日志输出,方便查看仿真过程中的调试信息。main函数是程序的入口,其中CommandLine类用于解析命令行参数,方便设置仿真参数。NS_LOG_UNCOND用于输出日志信息。
相关问题
给一个ns-3.27基于NS-3的WIFI性能仿真代码
以下是一个基于ns-3.27的NS-3 WIFI性能仿真代码,用于模拟802.11n网络的性能。
```
#include "ns3/core-module.h"
#include "ns3/mobility-module.h"
#include "ns3/wifi-module.h"
#include "ns3/internet-module.h"
#include "ns3/network-module.h"
#include "ns3/applications-module.h"
#include "ns3/flow-monitor-helper.h"
#include "ns3/flow-monitor-module.h"
using namespace ns3;
NS_LOG_COMPONENT_DEFINE ("WifiN");
int main (int argc, char *argv[])
{
uint32_t nWifi = 3;
bool verbose = false;
CommandLine cmd;
cmd.AddValue ("nWifi", "Number of wifi STA devices", nWifi);
cmd.AddValue ("verbose", "Turn on all WifiNetDevice log components", verbose);
cmd.Parse (argc,argv);
if (verbose)
{
LogComponentEnableAll (LOG_LEVEL_INFO);
LogComponentEnable ("WifiNetDevice", LOG_LEVEL_ALL);
}
NodeContainer wifiStaNodes;
wifiStaNodes.Create (nWifi);
NodeContainer wifiApNode;
wifiApNode.Create (1);
YansWifiChannelHelper channel = YansWifiChannelHelper::Default ();
YansWifiPhyHelper phy = YansWifiPhyHelper::Default ();
phy.SetChannel (channel.Create ());
WifiHelper wifi = WifiHelper::Default ();
wifi.SetRemoteStationManager ("ns3::AarfWifiManager");
NqosWifiMacHelper mac = NqosWifiMacHelper::Default ();
Ssid ssid = Ssid ("ns-3-ssid");
mac.SetType ("ns3::StaWifiMac", "Ssid", SsidValue (ssid), "ActiveProbing", BooleanValue (false));
NetDeviceContainer staDevices;
staDevices = wifi.Install (phy, mac, wifiStaNodes);
mac.SetType ("ns3::ApWifiMac", "Ssid", SsidValue (ssid));
NetDeviceContainer apDevice;
apDevice = wifi.Install (phy, mac, wifiApNode);
MobilityHelper mobility;
mobility.SetPositionAllocator ("ns3::GridPositionAllocator",
"MinX", DoubleValue (0.0),
"MinY", DoubleValue (0.0),
"DeltaX", DoubleValue (5.0),
"DeltaY", DoubleValue (10.0),
"GridWidth", UintegerValue (3),
"LayoutType", StringValue ("RowFirst"));
mobility.SetMobilityModel ("ns3::RandomWalk2dMobilityModel",
"Bounds", RectangleValue (Rectangle (-50, 50, -50, 50)));
mobility.Install (wifiStaNodes);
mobility.SetMobilityModel ("ns3::ConstantPositionMobilityModel");
mobility.Install (wifiApNode);
InternetStackHelper stack;
stack.Install (wifiApNode);
stack.Install (wifiStaNodes);
Ipv4AddressHelper address;
address.SetBase ("10.1.1.0", "255.255.255.0");
Ipv4InterfaceContainer staNodeInterface;
staNodeInterface = address.Assign (staDevices);
address.SetBase ("10.1.2.0", "255.255.255.0");
Ipv4InterfaceContainer apNodeInterface;
apNodeInterface = address.Assign (apDevice);
UdpEchoServerHelper echoServer (9);
ApplicationContainer serverApps = echoServer.Install (wifiApNode.Get (0));
serverApps.Start (Seconds (1.0));
serverApps.Stop (Seconds (10.0));
UdpEchoClientHelper echoClient (apNodeInterface.GetAddress (0), 9);
echoClient.SetAttribute ("MaxPackets", UintegerValue (1));
echoClient.SetAttribute ("Interval", TimeValue (Seconds (1.0)));
echoClient.SetAttribute ("PacketSize", UintegerValue (1024));
ApplicationContainer clientApps = echoClient.Install (wifiStaNodes.Get (0));
clientApps.Start (Seconds (2.0));
clientApps.Stop (Seconds (10.0));
FlowMonitorHelper flowmon;
Ptr<FlowMonitor> monitor = flowmon.InstallAll ();
Simulator::Stop (Seconds (10.0));
Simulator::Run ();
monitor->CheckForLostPackets ();
Ptr<Ipv4FlowClassifier> classifier = DynamicCast<Ipv4FlowClassifier> (flowmon.GetClassifier ());
std::map<FlowId, FlowMonitor::FlowStats> stats = monitor->GetFlowStats ();
for (std::map<FlowId, FlowMonitor::FlowStats>::const_iterator i = stats.begin (); i != stats.end (); ++i)
{
Ipv4FlowClassifier::FiveTuple t = classifier->FindFlow (i->first);
std::cout << "Flow " << i->first << " (" << t.sourceAddress << " -> " << t.destinationAddress << ")\n";
std::cout << " Tx Bytes: " << i->second.txBytes << "\n";
std::cout << " Rx Bytes: " << i->second.rxBytes << "\n";
std::cout << " Throughput: " << i->second.rxBytes * 8.0 / 9.0 / 1000 / 1000 << " Mbps\n";
}
Simulator::Destroy ();
return 0;
}
```
这个代码创建了一个包含一个AP和三个STA的802.11n网络,使用UDP Echo协议进行通信,并使用Flow Monitor模块监测网络流量。
ns3 first.cc
"ns3 first.cc" 可能是一个IT类问题,它可能指的是使用ns-3网络仿真器创建的第一个C++程序。如果是这样,下面是一些参考答案:
ns-3是一个用于网络仿真的开源C++库。 "ns3 first.cc" 可能指的是创建ns-3程序的第一个源代码文件。下面是一个简单的示例程序,用于在ns-3中创建一个简单的网络拓扑,并演示如何在拓扑中发送和接收数据包。
```c++
#include "ns3/core-module.h"
#include "ns3/network-module.h"
#include "ns3/internet-module.h"
#include "ns3/point-to-point-module.h"
using namespace ns3;
NS_LOG_COMPONENT_DEFINE ("FirstScriptExample");
int
main (int argc, char *argv[])
{
Time::SetResolution (Time::NS);
LogComponentEnable ("UdpEchoClientApplication", LOG_LEVEL_INFO);
LogComponentEnable ("UdpEchoServerApplication", LOG_LEVEL_INFO);
NodeContainer nodes;
nodes.Create (2);
PointToPointHelper pointToPoint;
pointToPoint.SetDeviceAttribute ("DataRate", StringValue ("5Mbps"));
pointToPoint.SetChannelAttribute ("Delay", StringValue ("2ms"));
NetDeviceContainer devices;
devices = pointToPoint.Install (nodes);
InternetStackHelper stack;
stack.Install (nodes);
Ipv4AddressHelper address;
address.SetBase ("10.1.1.0", "255.255.255.0");
Ipv4InterfaceContainer interfaces = address.Assign (devices);
UdpEchoServerHelper echoServer (9);
ApplicationContainer serverApps = echoServer.Install (nodes.Get (1));
serverApps.Start (Seconds (1.0));
serverApps.Stop (Seconds (10.0));
UdpEchoClientHelper echoClient (interfaces.GetAddress (1), 9);
echoClient.SetAttribute ("MaxPackets", UintegerValue (1));
echoClient.SetAttribute ("Interval", TimeValue (Seconds (1.0)));
echoClient.SetAttribute ("PacketSize", UintegerValue (1024));
ApplicationContainer clientApps = echoClient.Install (nodes.Get (0));
clientApps.Start (Seconds (2.0));
clientApps.Stop (Seconds (10.0));
Simulator::Run ();
Simulator::Destroy ();
return 0;
}
```
这个程序创建了两个节点,将它们连接在一起,并在其中一个节点上启动一个UDP echo服务器,然后在另一个节点上启动一个UDP echo客户端。在客户端上,它向服务器发送一个数据包,服务器将接收它并将其回显回去。这个程序可以通过编译器编译并在ns-3中运行。
阅读全文
相关推荐
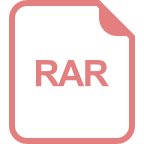














