python俄罗斯方块代码
时间: 2023-08-29 17:10:46 浏览: 41
以下是一个简单的 Python 俄罗斯方块游戏代码示例:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 游戏区域大小
screen_width = 800
screen_height = 600
# 创建游戏窗口
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("俄罗斯方块")
# 方块大小
block_size = 30
# 方块颜色
colors = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0)]
# 方块形状
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
# 游戏区域
play_area = [[0] * (screen_width // block_size) for _ in range(screen_height // block_size)]
# 当前方块
current_shape = []
current_color = 0
current_x = 0
current_y = 0
# 随机生成方块和颜色
def new_shape():
global current_shape, current_color, current_x, current_y
current_shape = random.choice(shapes)
current_color = random.randint(1, len(colors)-1)
current_x = len(play_area[0]) // 2 - len(current_shape[0]) // 2
current_y = 0
# 绘制游戏区域
def draw_play_area():
for y, row in enumerate(play_area):
for x, val in enumerate(row):
pygame.draw.rect(screen, colors[val], (x * block_size, y * block_size, block_size, block_size), 0)
# 绘制当前方块
def draw_current_shape():
for y, row in enumerate(current_shape):
for x, val in enumerate(row):
if val:
pygame.draw.rect(screen, colors[current_color], ((current_x + x) * block_size, (current_y + y) * block_size, block_size, block_size), 0)
# 检测方块是否碰到游戏区域边界或其他方块
def check_collision():
for y, row in enumerate(current_shape):
for x, val in enumerate(row):
if val:
if y + current_y >= len(play_area) or x + current_x < 0 or x + current_x >= len(play_area[0]) or play_area[y + current_y][x + current_x]:
return True
return False
# 将当前方块固定到游戏区域
def fix_current_shape():
for y, row in enumerate(current_shape):
for x, val in enumerate(row):
if val:
play_area[y + current_y][x + current_x] = current_color
# 消除完整的行
def clear_rows():
global play_area
play_area = [row for row in play_area if 0 not in row]
cleared_rows = [[0] * len(play_area[0])] * (screen_height // block_size - len(play_area))
play_area = cleared_rows + play_area
# 游戏循环
clock = pygame.time.Clock()
new_shape()
game_over = False
while not game_over:
clock.tick(5)
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_x -= 1
if check_collision():
current_x += 1
elif event.key == pygame.K_RIGHT:
current_x += 1
if check_collision():
current_x -= 1
elif event.key == pygame.K_DOWN:
current_y += 1
if check_collision():
current_y -= 1
elif event.key == pygame.K_UP:
current_shape = [list(reversed(row)) for row in zip(*current_shape)]
if check_collision():
current_shape = [list(row) for row in zip(*reversed(current_shape))]
elif event.key == pygame.K_SPACE:
while not check_collision():
current_y += 1
current_y -= 1
# 更新游戏区域和当前方块
screen.fill(colors[0])
draw_play_area()
draw_current_shape()
# 检测是否需要固定当前方块
if check_collision():
current_y -= 1
fix_current_shape()
clear_rows()
new_shape()
if check_collision():
game_over = True
# 更新屏幕
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这个示例代码可能有些简单,但可以作为一个基础框架来进行扩展和优化。
相关推荐
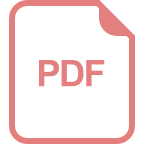
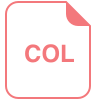
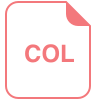
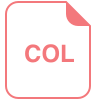
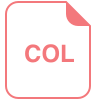
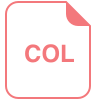








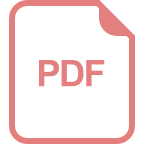