用python编写扫雷程序
时间: 2023-08-30 07:11:27 浏览: 61
好的,下面是一个简单的扫雷游戏程序,你可以在此基础上进行修改和扩展:
```python
import random
# 初始化游戏地图
def init_map(row, col, mine_num):
# 初始化地图
map = [[0 for i in range(col)] for j in range(row)]
# 随机布雷
for i in range(mine_num):
x = random.randint(0, row - 1)
y = random.randint(0, col - 1)
map[x][y] = -1
# 计算每个方格周围的雷数
for i in range(row):
for j in range(col):
if map[i][j] == -1:
continue
count = 0
if i > 0 and j > 0 and map[i-1][j-1] == -1:
count += 1
if i > 0 and map[i-1][j] == -1:
count += 1
if i > 0 and j < col - 1 and map[i-1][j+1] == -1:
count += 1
if j > 0 and map[i][j-1] == -1:
count += 1
if j < col - 1 and map[i][j+1] == -1:
count += 1
if i < row - 1 and j > 0 and map[i+1][j-1] == -1:
count += 1
if i < row - 1 and map[i+1][j] == -1:
count += 1
if i < row - 1 and j < col - 1 and map[i+1][j+1] == -1:
count += 1
map[i][j] = count
return map
# 打印地图
def print_map(map):
for row in map:
for col in row:
if col == -1:
print("*", end=" ")
else:
print(col, end=" ")
print()
# 点击一个方格
def click(map, row, col):
if map[row][col] == -1: # 点到雷了
return False, map
elif map[row][col] > 0: # 周围有雷
map[row][col] = -2 # 已经点击过
return True, map
else: # 周围没有雷
map[row][col] = -2 # 已经点击过
if row > 0 and col > 0 and map[row-1][col-1] != -2:
click(map, row-1, col-1)
if row > 0 and map[row-1][col] != -2:
click(map, row-1, col)
if row > 0 and col < len(map[0]) - 1 and map[row-1][col+1] != -2:
click(map, row-1, col+1)
if col > 0 and map[row][col-1] != -2:
click(map, row, col-1)
if col < len(map[0]) - 1 and map[row][col+1] != -2:
click(map, row, col+1)
if row < len(map) - 1 and col > 0 and map[row+1][col-1] != -2:
click(map, row+1, col-1)
if row < len(map) - 1 and map[row+1][col] != -2:
click(map, row+1, col)
if row < len(map) - 1 and col < len(map[0]) - 1 and map[row+1][col+1] != -2:
click(map, row+1, col+1)
return True, map
# 主函数
def main():
print("欢迎来到扫雷游戏!")
row = int(input("请输入行数:"))
col = int(input("请输入列数:"))
mine_num = int(input("请输入雷数:"))
map = init_map(row, col, mine_num)
print("地图如下:")
print_map(map)
while True:
row_input = input("请输入要点击的方格行数(1~{}),输入q退出:".format(row))
if row_input == "q":
break
col_input = input("请输入要点击的方格列数(1~{}),输入q退出:".format(col))
if col_input == "q":
break
row_click = int(row_input) - 1
col_click = int(col_input) - 1
if row_click < 0 or row_click >= row or col_click < 0 or col_click >= col:
print("输入不合法,请重新输入!")
continue
result, map = click(map, row_click, col_click)
if not result:
print("游戏结束,你输了!")
print_map(map)
break
else:
print("你点击了一个方格,地图如下:")
print_map(map)
# 判断是否胜利
win = True
for row in map:
if -2 not in row and -1 in row:
win = False
break
if win:
print("恭喜你,你赢了!")
print_map(map)
break
if __name__ == '__main__':
main()
```
这个程序使用了递归算法实现了自动翻开周围没有雷的方格的功能。你可以根据自己的需求进行修改和扩展。
相关推荐
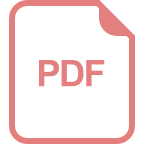
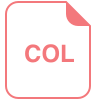
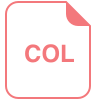
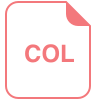
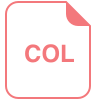
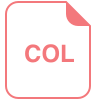









