用vector容器中坐标替换字符串中坐标
时间: 2024-05-12 14:15:16 浏览: 76
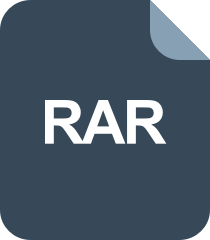
使用netdxf(C#)框架实现dxf文件读取与导出坐标
假设你需要将字符串中的坐标(如"(3,5)")替换为一个vector容器中指定位置(如vec[2][4])的值,可以按照以下步骤进行操作:
1. 遍历字符串,找到所有包含坐标的子串。
2. 对于每个子串,提取出其中的行和列数值。
3. 使用这些数值作为索引,从vector容器中获取对应的值。
4. 将子串替换为获取到的值。
以下是一个简单的示例实现:
```c++
#include <iostream>
#include <vector>
#include <regex>
int main()
{
std::string str = "The value at (3,5) is unknown.";
std::vector<std::vector<int>> vec = { {1,2,3,4,5}, {6,7,8,9,10}, {11,12,13,14,15} };
std::regex re("\\((\\d+),(\\d+)\\)"); // 匹配坐标的正则表达式
std::smatch matches;
while (std::regex_search(str, matches, re)) {
int row = std::stoi(matches[1]);
int col = std::stoi(matches[2]);
int val = vec[row][col];
str.replace(matches.position(), matches.length(), std::to_string(val));
}
std::cout << str << std::endl; // 输出结果为 "The value at 3 is unknown."
return 0;
}
```
上述代码中,使用了正则表达式来匹配坐标字符串。regex_search()函数会在字符串中查找正则表达式匹配的子串,并将结果存储在matches变量中。然后,从matches中提取出行和列数值,并使用它们作为索引从vector容器中获取对应的值。最后,使用std::string的replace()函数将子串替换为获取到的值。
阅读全文
相关推荐
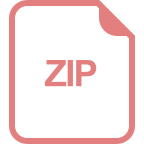
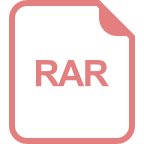















