用C++实现:[问题描述] 商品货架可以看成一个栈,栈顶商品的生产日期最早,栈底商品的生产日期最近。上货时,需要倒货架,以保证生产日期较近的商品在较下的位置。 [基本要求] 针对一种特定商品,实现上述管理过程。货架信息从文件中读取,上货后在输出至文件和屏幕。 [实现提示] 用栈模拟货架和周转空间。 [测试数据] 注意测试边界数据,如空栈。
时间: 2024-01-24 16:17:15 浏览: 36
以下是用 C++ 实现的代码,实现了上货、展示货架和保存货架信息到文件的功能:
```cpp
#include <iostream>
#include <fstream>
#include <stack>
#include <vector>
#include <algorithm>
using namespace std;
struct Commodity {
int id; // 商品编号
string name; // 商品名称
string production; // 生产日期
};
bool cmp(const Commodity &a, const Commodity &b) {
return a.production < b.production;
}
class Shelf {
private:
stack<Commodity> shelfStack;
public:
void loadShelf(const vector<Commodity> &commodities) {
// 先将货架清空
while (!shelfStack.empty()) {
shelfStack.pop();
}
// 将新商品逐个入栈
for (auto &commodity : commodities) {
shelfStack.push(commodity);
}
// 反转栈,以保证生产日期较近的商品在较下的位置
stack<Commodity> tmpStack;
while (!shelfStack.empty()) {
tmpStack.push(shelfStack.top());
shelfStack.pop();
}
shelfStack = tmpStack;
}
void showShelf() const {
if (shelfStack.empty()) {
cout << "货架为空" << endl;
} else {
cout << "货架上的商品信息:" << endl;
stack<Commodity> tmpStack = shelfStack;
while (!tmpStack.empty()) {
cout << "商品编号:" << tmpStack.top().id << ", 商品名称:" << tmpStack.top().name << ", 生产日期:" << tmpStack.top().production << endl;
tmpStack.pop();
}
}
}
void saveShelfToFile(const string &filename) const {
ofstream outputFile(filename);
if (!outputFile) {
cerr << "无法打开文件 " << filename << " 进行写入" << endl;
return;
}
if (shelfStack.empty()) {
outputFile << "货架为空" << endl;
} else {
outputFile << "货架上的商品信息:" << endl;
stack<Commodity> tmpStack = shelfStack;
while (!tmpStack.empty()) {
outputFile << "商品编号:" << tmpStack.top().id << ", 商品名称:" << tmpStack.top().name << ", 生产日期:" << tmpStack.top().production << endl;
tmpStack.pop();
}
}
outputFile.close();
}
};
int main() {
vector<Commodity> commodities;
string filename = "commodities.txt";
ifstream inputFile(filename);
if (!inputFile) {
cerr << "无法打开文件 " << filename << " 进行读取" << endl;
return 1;
}
int id;
string name, production;
while (inputFile >> id >> name >> production) {
Commodity commodity = {id, name, production};
commodities.push_back(commodity);
}
inputFile.close();
sort(commodities.begin(), commodities.end(), cmp);
Shelf shelf;
shelf.loadShelf(commodities);
shelf.showShelf();
shelf.saveShelfToFile("shelf.txt");
return 0;
}
```
其中,`Commodity` 结构体表示一个商品,包含商品编号、名称和生产日期。`cmp` 函数用于比较两个商品的生产日期,以便在读取完所有商品信息后进行排序。`Shelf` 类表示一个货架,包含一个栈来模拟货架,以及上货、展示货架和保存货架信息到文件的方法。在 `main` 函数中,首先从文件中读取所有商品信息,并按生产日期排序。然后,创建一个 `Shelf` 对象,将排好序的商品逐个入栈,并展示货架信息和保存货架信息到文件。
相关推荐
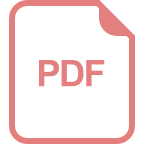
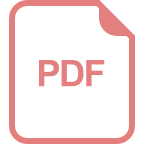
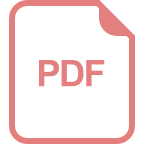














