PyOpenGL将屏幕坐标转换为世界坐标的代码
时间: 2023-05-25 16:05:16 浏览: 125
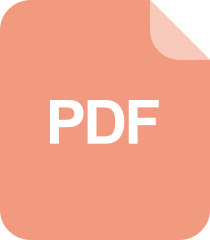
使用PyOpenGL绘制三维坐标系实例
以下是使用PyOpenGL将屏幕坐标转换为世界坐标的代码示例:
```python
import numpy as np
from OpenGL.GLUT import *
# 在窗口大小改变时调用
def reshape(width, height):
glViewport(0, 0, width, height)
glMatrixMode(GL_PROJECTION)
glLoadIdentity()
gluPerspective(45.0, float(width) / height, 0.1, 100.0)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
# 在窗口绘制时调用
def display():
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
# 设置相机位置和朝向
gluLookAt(0, 0, 5, 0, 0, 0, 0, 1, 0)
# 绘制一个红色的三角形
glColor3f(1.0, 0.0, 0.0)
glBegin(GL_TRIANGLES)
glVertex3f(0.0, 1.0, 0.0)
glVertex3f(-1.0, -1.0, 0.0)
glVertex3f(1.0, -1.0, 0.0)
glEnd()
glutSwapBuffers()
# 在鼠标点击时调用
def mouse(button, state, x, y):
if button == GLUT_LEFT_BUTTON and state == GLUT_DOWN:
# 获取当前窗口的大小
width, height = glutGet(GLUT_WINDOW_WIDTH), glutGet(GLUT_WINDOW_HEIGHT)
# 将屏幕坐标转换为NDC坐标
x_ndc = 2.0 * x / width - 1.0
y_ndc = 1.0 - 2.0 * y / height
# 将NDC坐标转换为世界坐标
modelview = np.array(glGetDoublev(GL_MODELVIEW_MATRIX))
projection = np.array(glGetDoublev(GL_PROJECTION_MATRIX))
viewport = np.array(glGetIntegerv(GL_VIEWPORT))
x, y, z = gluUnProject(x_ndc, y_ndc, 0.0, modelview, projection, viewport)
print("World coordinate:", x, y, z)
glutInit()
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGBA | GLUT_DEPTH)
glutInitWindowSize(640, 480)
glutCreateWindow("PyOpenGL Example")
glutReshapeFunc(reshape)
glutDisplayFunc(display)
glutMouseFunc(mouse)
glutMainLoop()
```
在`mouse`函数中,我们先将屏幕坐标(`x`,`y`)转换为NDC坐标(`x_ndc`,`y_ndc`),然后再使用`gluUnProject`函数将NDC坐标转换为世界坐标(`x`,`y`,`z`)。为了使用`gluUnProject`函数,我们需要先获得当前的`modelview`,`projection`和`viewport`矩阵。这些矩阵可以使用`glGetDoublev`和`glGetIntegerv`函数获取。
阅读全文
相关推荐
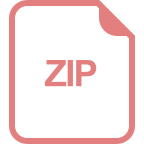
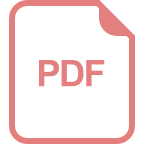















