python中通过牛顿迭代法求解平方根
时间: 2024-04-08 13:23:16 浏览: 238
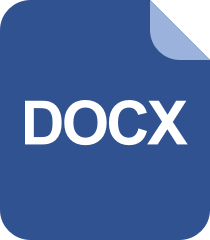
python迭代法求正数的平方根
可以使用以下代码实现牛顿迭代法求解平方根:
```
def newton_sqrt(num, accuracy=0.0001):
if num < 0:
return None
x = num
while True:
root = 0.5 * (x + num / x)
if abs(root - x) < accuracy:
return root
x = root
```
这是一个计算平方根的函数,它接受一个数字和一个精度参数,返回这个数字的平方根。它使用牛顿迭代法来逼近平方根的值,直到达到所需的精度为止。
阅读全文
相关推荐
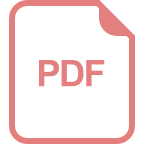



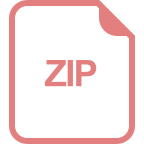
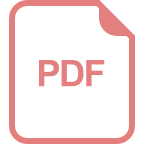










