public float moveSpeed = 10; Transform target; private int pointIndex = 0; // Start is called before the first frame update void Start() { target = PathPoints.pathPoints[pointIndex]; } // Update is called once per frame void Update() { Vector3 dir = target.position - transform.position; transform.Translate(dir.normalized * moveSpeed * Time.deltaTime, Space.World); if (Vector3.Distance(target.position, transform.position) < 0.2f) { pointIndex++; target = PathPoints.pathPoints[pointIndex]; if (pointIndex >= PathPoints.pathPoints.Length) { Destroy(gameObject); return; } } }
时间: 2024-02-14 09:04:49 浏览: 32
这段代码是一个移动物体的脚本。其中moveSpeed是移动的速度,target是物体要移动到的目标点(使用了另一个脚本PathPoints中的pathPoints数组),pointIndex是当前目标点在路径数组中的索引。
在Start方法中,获取了路径数组中第一个目标点作为初始目标点。在Update方法中,先计算出物体到目标点的方向向量dir,然后使用Translate方法按照该方向向量将物体移动一定的距离。如果物体到达了目标点,就将目标点索引加1,并获取下一个目标点作为新的目标点。如果已经到达了路径数组的末尾,则销毁这个物体。
相关问题
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Obstacle : MonoBehaviour { public int hurtValue = 1; public int moveSpeed = 0; // Use this for initialization void Start () { } // Update is called once per frame void Update () { transform.Translate(0, 0, moveSpeed * Time.deltaTime); } public virtual void OnTriggerEnter(Collider other) { if (other.tag == "Player") { CameraManager.instance.CameraShake(); GameAttribute.instance.life -= hurtValue; AudioManager.instance.PlayHitAudio(); } if (other.tag != "Road" && other.tag !="MagnetCollider") { moveSpeed = 0; } } }
这是一个在Unity引擎中使用C#编写的脚本,名为Obstacle。该脚本被应用到了游戏中的障碍物物体上,用于处理障碍物的行为逻辑。
其中定义了hurtValue和moveSpeed两个变量,分别表示障碍物的伤害值和移动速度。
Start函数和Update函数是Unity引擎提供的生命周期函数,Start函数在脚本启动时调用,Update函数在每一帧都会被调用。在这里,Update函数实现了障碍物的移动,每一帧根据moveSpeed变量的值在z轴方向上进行平移。
OnTriggerEnter函数是Unity引擎提供的触发器碰撞事件处理函数,当障碍物与其他碰撞体产生碰撞时会被调用。在这里,当碰撞体标签为"Player"时,会进行摄像机震动、扣除生命值和播放受伤音效等操作。如果碰撞体标签不是"Road"或"MagnetCollider",则将moveSpeed设为0,表示障碍物停止移动。需要注意的是,OnTriggerEnter函数被定义为virtual,意味着它可以被子类覆盖并重新实现。
你上面的解释错了,我的Spline脚本里有的公共变量如下: public GameObject DraggablePrefab; public float NewNodeOffset; public List<SplineSegment> Segments = new List<SplineSegment>(); public float SpawnStartDelay = 0; public float SpawnDelay = 1; public int SpawnCount = 0; public float MoveSpeed = 0.5f; public DraggableSplineEndAction DraggableSplineEndAction; public float PreWarmTime = 100f;解释一下
非常抱歉,我之前的解释有误。下面是对您提供的Spline脚本中各个公共变量的解释:
1. `public GameObject DraggablePrefab` - 这个变量指定了一个可拖动的预制体,可以用于在曲线上创建新的节点。
2. `public float NewNodeOffset` - 这个变量用于指定新节点在曲线上的位置。如果值为0,则新节点将创建在当前选择的节点后面。
3. `public List<SplineSegment> Segments = new List<SplineSegment>()` - 这个变量是一个SplineSegment类型的列表,用于存储曲线的所有段。
4. `public float SpawnStartDelay = 0` - 这个变量用于指定在开始生成曲线节点之前的延迟时间。
5. `public float SpawnDelay = 1` - 这个变量用于指定生成曲线节点之间的延迟时间。
6. `public int SpawnCount = 0` - 这个变量用于指定要生成的曲线节点的数量。如果设置为0,则不会生成任何节点。
7. `public float MoveSpeed = 0.5f` - 这个变量用于指定曲线节点运动的速度。
8. `public DraggableSplineEndAction DraggableSplineEndAction` - 这个变量是一个枚举类型,用于指定在拖动曲线节点时的行为。
9. `public float PreWarmTime = 100f` - 这个变量用于指定在游戏开始时预热曲线的时间。这可以确保曲线在游戏开始时已经准备好了。
相关推荐
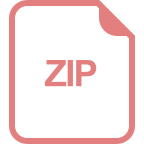
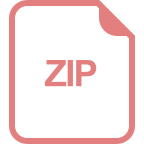
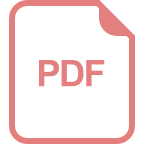













