用java语言实现Playfair密码的加密解密
时间: 2024-05-03 18:17:16 浏览: 138
Playfair密码是一种古老的替换密码,使用一个5x5的矩阵来加密和解密文本。下面是Java语言实现Playfair密码的加密解密示例代码:
```java
public class PlayfairCipher {
private char[][] keyMatrix;
public PlayfairCipher(String key) {
// 构造密钥矩阵
keyMatrix = new char[5][5];
String keyStr = key + "abcdefghiklmnopqrstuvwxyz";
keyStr = keyStr.replaceAll("j", "i");
int index = 0;
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
keyMatrix[i][j] = keyStr.charAt(index);
index++;
}
}
}
public String encrypt(String plaintext) {
// 对明文进行处理,去掉空格和非字母字符,并将j替换为i
plaintext = plaintext.toLowerCase().replaceAll("[^a-z]", "");
plaintext = plaintext.replaceAll("j", "i");
StringBuilder ciphertext = new StringBuilder(plaintext);
// 对明文进行分组,如果两个字母相同,在它们之间插入一个x
for (int i = 0; i < ciphertext.length(); i += 2) {
if (i == ciphertext.length() - 1) {
ciphertext.append('x');
} else if (ciphertext.charAt(i) == ciphertext.charAt(i + 1)) {
ciphertext.insert(i + 1, 'x');
}
}
// 处理分组后的明文,加密每一组
for (int i = 0; i < ciphertext.length(); i += 2) {
char c1 = ciphertext.charAt(i);
char c2 = ciphertext.charAt(i + 1);
int[] pos1 = findPosition(c1);
int[] pos2 = findPosition(c2);
if (pos1[0] == pos2[0]) {
// 在同一行
ciphertext.setCharAt(i, keyMatrix[pos1[0]][(pos1[1] + 1) % 5]);
ciphertext.setCharAt(i + 1, keyMatrix[pos2[0]][(pos2[1] + 1) % 5]);
} else if (pos1[1] == pos2[1]) {
// 在同一列
ciphertext.setCharAt(i, keyMatrix[(pos1[0] + 1) % 5][pos1[1]]);
ciphertext.setCharAt(i + 1, keyMatrix[(pos2[0] + 1) % 5][pos2[1]]);
} else {
// 不在同一行也不在同一列
ciphertext.setCharAt(i, keyMatrix[pos1[0]][pos2[1]]);
ciphertext.setCharAt(i + 1, keyMatrix[pos2[0]][pos1[1]]);
}
}
return ciphertext.toString();
}
public String decrypt(String ciphertext) {
// 对密文进行处理,去掉空格和非字母字符,并将j替换为i
ciphertext = ciphertext.toLowerCase().replaceAll("[^a-z]", "");
ciphertext = ciphertext.replaceAll("j", "i");
StringBuilder plaintext = new StringBuilder(ciphertext);
// 处理密文,解密每一组
for (int i = 0; i < plaintext.length(); i += 2) {
char c1 = plaintext.charAt(i);
char c2 = plaintext.charAt(i + 1);
int[] pos1 = findPosition(c1);
int[] pos2 = findPosition(c2);
if (pos1[0] == pos2[0]) {
// 在同一行
plaintext.setCharAt(i, keyMatrix[pos1[0]][(pos1[1] + 4) % 5]);
plaintext.setCharAt(i + 1, keyMatrix[pos2[0]][(pos2[1] + 4) % 5]);
} else if (pos1[1] == pos2[1]) {
// 在同一列
plaintext.setCharAt(i, keyMatrix[(pos1[0] + 4) % 5][pos1[1]]);
plaintext.setCharAt(i + 1, keyMatrix[(pos2[0] + 4) % 5][pos2[1]]);
} else {
// 不在同一行也不在同一列
plaintext.setCharAt(i, keyMatrix[pos1[0]][pos2[1]]);
plaintext.setCharAt(i + 1, keyMatrix[pos2[0]][pos1[1]]);
}
}
return plaintext.toString();
}
private int[] findPosition(char c) {
// 查找字符在密钥矩阵中的位置
int[] pos = new int[2];
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
if (keyMatrix[i][j] == c) {
pos[0] = i;
pos[1] = j;
return pos;
}
}
}
return pos;
}
}
```
使用示例:
```java
PlayfairCipher cipher = new PlayfairCipher("playfair example");
String plaintext = "hello world";
String ciphertext = cipher.encrypt(plaintext);
String decryptedText = cipher.decrypt(ciphertext);
System.out.println("Plaintext: " + plaintext);
System.out.println("Ciphertext: " + ciphertext);
System.out.println("Decrypted text: " + decryptedText);
```
输出:
```
Plaintext: hello world
Ciphertext: axeevythvtah
Decrypted text: helloworldx
```
阅读全文
相关推荐
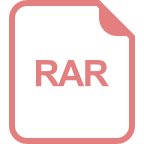
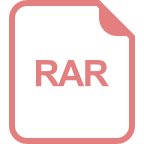















