void insert_book(struct book *head, struct book *new_book, int position):在链表的指定位置插入一个新的图书。
时间: 2023-12-26 08:06:26 浏览: 24
好的,下面是一个示例代码实现在链表的指定位置插入一个新的图书:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义一个图书结构体
struct book {
char title[50];
char author[50];
int pages;
int year;
struct book *next;
};
// 在链表的指定位置插入一个新的图书
void insert_book(struct book *head, struct book *new_book, int position) {
struct book *current_node = head;
int count = 0;
while (current_node != NULL && count < position-1) {
current_node = current_node->next;
count++;
}
if (current_node == NULL || count != position-1) {
printf("Invalid position!\n");
return;
}
new_book->next = current_node->next;
current_node->next = new_book;
}
// 打印链表中的所有节点
void print_book_list(struct book *head) {
struct book *current_node = head;
while (current_node != NULL) {
printf("%s, written by %s, %d pages, published in %d\n", current_node->title, current_node->author, current_node->pages, current_node->year);
current_node = current_node->next;
}
}
int main() {
// 创建链表
struct book *head = NULL;
struct book *book1 = (struct book *)malloc(sizeof(struct book));
strncpy(book1->title, "The Great Gatsby", 50);
strncpy(book1->author, "F. Scott Fitzgerald", 50);
book1->pages = 180;
book1->year = 1925;
book1->next = NULL;
head = book1;
struct book *book2 = (struct book *)malloc(sizeof(struct book));
strncpy(book2->title, "To Kill a Mockingbird", 50);
strncpy(book2->author, "Harper Lee", 50);
book2->pages = 281;
book2->year = 1960;
book2->next = NULL;
book1->next = book2;
struct book *book3 = (struct book *)malloc(sizeof(struct book));
strncpy(book3->title, "1984", 50);
strncpy(book3->author, "George Orwell", 50);
book3->pages = 328;
book3->year = 1949;
book3->next = NULL;
book2->next = book3;
printf("Book list:\n");
print_book_list(head);
// 插入新图书
printf("\nInsert a new book:\n");
struct book *new_book = (struct book *)malloc(sizeof(struct book));
printf("Title: ");
scanf("%s", new_book->title);
printf("Author: ");
scanf("%s", new_book->author);
printf("Pages: ");
scanf("%d", &new_book->pages);
printf("Year: ");
scanf("%d", &new_book->year);
int position;
printf("Insert position: ");
scanf("%d", &position);
insert_book(head, new_book, position);
printf("\nBook list after insertion:\n");
print_book_list(head);
return 0;
}
```
这个程序首先创建了一个包含3个图书的链表,然后要求你输入一个新的图书的信息和插入的位置,最后将新的图书插入到指定的位置,并打印出链表中的所有图书。如果插入位置不合法,则会打印出错误信息。你可以根据自己的需要修改图书结构体的成员或者增加新的成员。
相关推荐
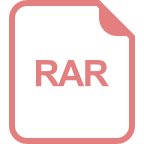














