编写map根据value值排序代码
时间: 2023-08-31 14:40:38 浏览: 121
### 回答1:
根据value值排序map的代码如下所示:Map<String, Integer> map = new HashMap<>();
map.put("A", 10);
map.put("B", 5);
map.put("C", 20);List<Map.Entry<String, Integer> > list = new ArrayList<Map.Entry<String, Integer> >(map.entrySet());
Collections.sort(list, new Comparator<Map.Entry<String, Integer> >() {
public int compare(Map.Entry<String, Integer> o1,
Map.Entry<String, Integer> o2)
{
return (o1.getValue()).compareTo(o2.getValue());
}
});
// Storing the list into Linked HashMap
// to preserve the order of insertion.
Map<String, Integer> sortedMap =
new LinkedHashMap<String, Integer>();
for (Map.Entry<String, Integer> entry : list)
{
sortedMap.put(entry.getKey(), entry.getValue());
}
### 回答2:
编写map根据value值排序的代码可以使用Java语言来实现,具体步骤如下:
1. 首先创建一个Map对象,并添加一些键值对,其中键为String类型,值为Integer类型。
2. 创建一个ArrayList对象,用于存放Map中的键值对。
3. 使用Map的entrySet()方法获取Map中的所有键值对,并将其添加到ArrayList中。
4. 创建一个比较器Comparator,重写compare()方法,比较两个键值对的value值大小。
5. 使用Collections的sort()方法,传入ArrayList和自定义的Comparator对象,实现对ArrayList中的键值对按照value值进行排序。
6. 遍历排序后的ArrayList,输出排序结果。
以下是示例代码:
```java
import java.util.*;
public class MapSortByValue {
public static void main(String[] args) {
// 创建Map对象并添加键值对
Map<String, Integer> map = new HashMap<>();
map.put("A", 5);
map.put("B", 3);
map.put("C", 8);
map.put("D", 1);
// 创建ArrayList对象并将键值对添加到其中
List<Map.Entry<String, Integer>> entryList = new ArrayList<>(map.entrySet());
// 使用Comparator比较器对ArrayList中的键值对按照value值进行排序
Collections.sort(entryList, new Comparator<Map.Entry<String, Integer>>() {
@Override
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o1.getValue().compareTo(o2.getValue());
}
});
// 遍历排序后的ArrayList,输出排序结果
for (Map.Entry<String, Integer> entry : entryList) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
}
}
```
以上代码会输出排序后的结果:
```
D : 1
B : 3
A : 5
C : 8
```
这段代码通过将Map中的键值对转换为ArrayList,再使用Collections的sort()方法,传入自定义的Comparator对象实现了根据value值进行排序的功能。
### 回答3:
要根据值对一个Map进行排序,可以按照以下步骤编写代码。
1. 首先,将原始的Map转换为List,以便后续操作。可以使用Map的entrySet()方法获取键值对的集合,再将其转换为List。
2. 在对List排序之前,需要创建一个自定义的比较器(Comparator)来实现按照值排序的逻辑。比较器需要实现Comparator接口,并重写compare方法。在compare方法中,获取两个Map.Entry的值进行比较。
3. 使用Collections.sort()方法对List进行排序,传入自定义的比较器作为参数。这样,List中的元素就按照值的大小进行了排序。
4. 最后,将排序后的List转换回Map。可以使用LinkedHashMap来保存排序后的结果,因为LinkedHashMap保留了元素的插入顺序。
下面是示例代码:
```
import java.util.*;
public class MapSortByValue {
public static void main(String[] args) {
// 原始的Map示例
Map<String, Integer> map = new HashMap<>();
map.put("apple", 5);
map.put("orange", 3);
map.put("banana", 2);
map.put("grape", 4);
// 将原始的Map转换为List
List<Map.Entry<String, Integer>> list = new ArrayList<>(map.entrySet());
// 创建自定义的比较器
Comparator<Map.Entry<String, Integer>> comparator = new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> entry1, Map.Entry<String, Integer> entry2) {
return entry1.getValue().compareTo(entry2.getValue());
}
};
// 对List进行排序
Collections.sort(list, comparator);
// 将排序后的List转换为Map
Map<String, Integer> sortedMap = new LinkedHashMap<>();
for (Map.Entry<String, Integer> entry : list) {
sortedMap.put(entry.getKey(), entry.getValue());
}
// 输出排序后的Map
for (Map.Entry<String, Integer> entry : sortedMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
上述代码在原始的Map中添加了几个水果和对应的数量,然后按照数量进行排序,并输出排序后的结果。运行代码后,输出顺序为:banana: 2, orange: 3, grape: 4, apple: 5。说明根据值排序的逻辑已经生效。
阅读全文
相关推荐
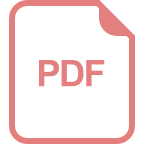

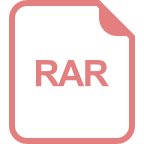
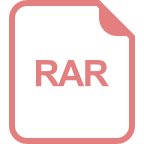
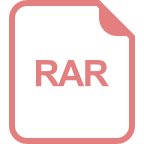
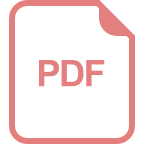
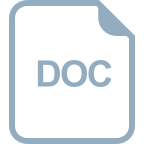
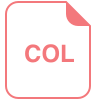
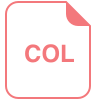







